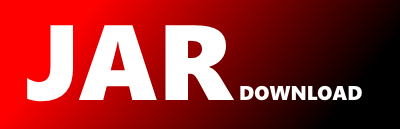
co.elastic.clients.elasticsearch.indices.DeleteIndexTemplateRequest Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.indices;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.elasticsearch._types.RequestBase;
import co.elastic.clients.elasticsearch._types.Time;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.endpoints.SimpleEndpoint;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import jakarta.json.stream.JsonGenerator;
import java.lang.String;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: indices.delete_index_template.Request
/**
* Delete an index template. The provided <index-template> may contain
* multiple template names separated by a comma. If multiple template names are
* specified then there is no wildcard support and the provided names should
* match completely with existing templates.
*
* @see API
* specification
*/
public class DeleteIndexTemplateRequest extends RequestBase {
@Nullable
private final Time masterTimeout;
private final List name;
@Nullable
private final Time timeout;
// ---------------------------------------------------------------------------------------------
private DeleteIndexTemplateRequest(Builder builder) {
this.masterTimeout = builder.masterTimeout;
this.name = ApiTypeHelper.unmodifiableRequired(builder.name, this, "name");
this.timeout = builder.timeout;
}
public static DeleteIndexTemplateRequest of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* Period to wait for a connection to the master node. If no response is
* received before the timeout expires, the request fails and returns an error.
*
* API name: {@code master_timeout}
*/
@Nullable
public final Time masterTimeout() {
return this.masterTimeout;
}
/**
* Required - Comma-separated list of index template names used to limit the
* request. Wildcard (*) expressions are supported.
*
* API name: {@code name}
*/
public final List name() {
return this.name;
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
@Nullable
public final Time timeout() {
return this.timeout;
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link DeleteIndexTemplateRequest}.
*/
public static class Builder extends RequestBase.AbstractBuilder
implements
ObjectBuilder {
@Nullable
private Time masterTimeout;
private List name;
@Nullable
private Time timeout;
/**
* Period to wait for a connection to the master node. If no response is
* received before the timeout expires, the request fails and returns an error.
*
* API name: {@code master_timeout}
*/
public final Builder masterTimeout(@Nullable Time value) {
this.masterTimeout = value;
return this;
}
/**
* Period to wait for a connection to the master node. If no response is
* received before the timeout expires, the request fails and returns an error.
*
* API name: {@code master_timeout}
*/
public final Builder masterTimeout(Function> fn) {
return this.masterTimeout(fn.apply(new Time.Builder()).build());
}
/**
* Required - Comma-separated list of index template names used to limit the
* request. Wildcard (*) expressions are supported.
*
* API name: {@code name}
*
* Adds all elements of list
to name
.
*/
public final Builder name(List list) {
this.name = _listAddAll(this.name, list);
return this;
}
/**
* Required - Comma-separated list of index template names used to limit the
* request. Wildcard (*) expressions are supported.
*
* API name: {@code name}
*
* Adds one or more values to name
.
*/
public final Builder name(String value, String... values) {
this.name = _listAdd(this.name, value, values);
return this;
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
public final Builder timeout(@Nullable Time value) {
this.timeout = value;
return this;
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
public final Builder timeout(Function> fn) {
return this.timeout(fn.apply(new Time.Builder()).build());
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link DeleteIndexTemplateRequest}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public DeleteIndexTemplateRequest build() {
_checkSingleUse();
return new DeleteIndexTemplateRequest(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Endpoint "{@code indices.delete_index_template}".
*/
public static final Endpoint _ENDPOINT = new SimpleEndpoint<>(
"es/indices.delete_index_template",
// Request method
request -> {
return "DELETE";
},
// Request path
request -> {
final int _name = 1 << 0;
int propsSet = 0;
propsSet |= _name;
if (propsSet == (_name)) {
StringBuilder buf = new StringBuilder();
buf.append("/_index_template");
buf.append("/");
SimpleEndpoint.pathEncode(request.name.stream().map(v -> v).collect(Collectors.joining(",")), buf);
return buf.toString();
}
throw SimpleEndpoint.noPathTemplateFound("path");
},
// Path parameters
request -> {
Map params = new HashMap<>();
final int _name = 1 << 0;
int propsSet = 0;
propsSet |= _name;
if (propsSet == (_name)) {
params.put("name", request.name.stream().map(v -> v).collect(Collectors.joining(",")));
}
return params;
},
// Request parameters
request -> {
Map params = new HashMap<>();
if (request.masterTimeout != null) {
params.put("master_timeout", request.masterTimeout._toJsonString());
}
if (request.timeout != null) {
params.put("timeout", request.timeout._toJsonString());
}
return params;
}, SimpleEndpoint.emptyMap(), false, DeleteIndexTemplateResponse._DESERIALIZER);
}