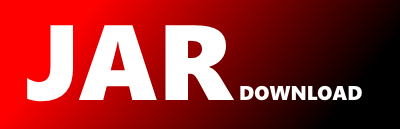
co.elastic.clients.elasticsearch.indices.ElasticsearchIndicesAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
The newest version!
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.indices;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the indices namespace.
*/
public class ElasticsearchIndicesAsyncClient
extends
ApiClient {
public ElasticsearchIndicesAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchIndicesAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchIndicesAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchIndicesAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: indices.add_block
/**
* Add an index block. Limits the operations allowed on an index by blocking
* specific operation types.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture addBlock(AddBlockRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AddBlockRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Add an index block. Limits the operations allowed on an index by blocking
* specific operation types.
*
* @param fn
* a function that initializes a builder to create the
* {@link AddBlockRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture addBlock(
Function> fn) {
return addBlock(fn.apply(new AddBlockRequest.Builder()).build());
}
// ----- Endpoint: indices.analyze
/**
* Get tokens from text analysis. The analyze API performs analysis
* on a text string and returns the resulting tokens.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture analyze(AnalyzeRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AnalyzeRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get tokens from text analysis. The analyze API performs analysis
* on a text string and returns the resulting tokens.
*
* @param fn
* a function that initializes a builder to create the
* {@link AnalyzeRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture analyze(
Function> fn) {
return analyze(fn.apply(new AnalyzeRequest.Builder()).build());
}
/**
* Get tokens from text analysis. The analyze API performs analysis
* on a text string and returns the resulting tokens.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture analyze() {
return this.transport.performRequestAsync(new AnalyzeRequest.Builder().build(), AnalyzeRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.clear_cache
/**
* Clears the caches of one or more indices. For data streams, the API clears
* the caches of the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCache(ClearCacheRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCacheRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clears the caches of one or more indices. For data streams, the API clears
* the caches of the stream’s backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCacheRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCache(
Function> fn) {
return clearCache(fn.apply(new ClearCacheRequest.Builder()).build());
}
/**
* Clears the caches of one or more indices. For data streams, the API clears
* the caches of the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCache() {
return this.transport.performRequestAsync(new ClearCacheRequest.Builder().build(), ClearCacheRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.clone
/**
* Clones an existing index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clone(CloneIndexRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloneIndexRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clones an existing index.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloneIndexRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clone(
Function> fn) {
return clone(fn.apply(new CloneIndexRequest.Builder()).build());
}
// ----- Endpoint: indices.close
/**
* Closes an index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture close(CloseIndexRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloseIndexRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Closes an index.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloseIndexRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture close(
Function> fn) {
return close(fn.apply(new CloseIndexRequest.Builder()).build());
}
// ----- Endpoint: indices.create
/**
* Create an index. Creates a new index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture create(CreateIndexRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateIndexRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create an index. Creates a new index.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateIndexRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture create(
Function> fn) {
return create(fn.apply(new CreateIndexRequest.Builder()).build());
}
// ----- Endpoint: indices.create_data_stream
/**
* Create a data stream. Creates a data stream. You must have a matching index
* template with data stream enabled.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createDataStream(CreateDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create a data stream. Creates a data stream. You must have a matching index
* template with data stream enabled.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createDataStream(
Function> fn) {
return createDataStream(fn.apply(new CreateDataStreamRequest.Builder()).build());
}
// ----- Endpoint: indices.data_streams_stats
/**
* Get data stream stats. Retrieves statistics for one or more data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture dataStreamsStats(DataStreamsStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DataStreamsStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data stream stats. Retrieves statistics for one or more data streams.
*
* @param fn
* a function that initializes a builder to create the
* {@link DataStreamsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture dataStreamsStats(
Function> fn) {
return dataStreamsStats(fn.apply(new DataStreamsStatsRequest.Builder()).build());
}
/**
* Get data stream stats. Retrieves statistics for one or more data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture dataStreamsStats() {
return this.transport.performRequestAsync(new DataStreamsStatsRequest.Builder().build(),
DataStreamsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.delete
/**
* Delete indices. Deletes one or more indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture delete(DeleteIndexRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteIndexRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete indices. Deletes one or more indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteIndexRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture delete(
Function> fn) {
return delete(fn.apply(new DeleteIndexRequest.Builder()).build());
}
// ----- Endpoint: indices.delete_alias
/**
* Delete an alias. Removes a data stream or index from an alias.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteAlias(DeleteAliasRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteAliasRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete an alias. Removes a data stream or index from an alias.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteAliasRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteAlias(
Function> fn) {
return deleteAlias(fn.apply(new DeleteAliasRequest.Builder()).build());
}
// ----- Endpoint: indices.delete_data_lifecycle
/**
* Delete data stream lifecycles. Removes the data stream lifecycle from a data
* stream, rendering it not managed by the data stream lifecycle.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteDataLifecycle(DeleteDataLifecycleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDataLifecycleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete data stream lifecycles. Removes the data stream lifecycle from a data
* stream, rendering it not managed by the data stream lifecycle.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDataLifecycleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteDataLifecycle(
Function> fn) {
return deleteDataLifecycle(fn.apply(new DeleteDataLifecycleRequest.Builder()).build());
}
// ----- Endpoint: indices.delete_data_stream
/**
* Delete data streams. Deletes one or more data streams and their backing
* indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteDataStream(DeleteDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete data streams. Deletes one or more data streams and their backing
* indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteDataStream(
Function> fn) {
return deleteDataStream(fn.apply(new DeleteDataStreamRequest.Builder()).build());
}
// ----- Endpoint: indices.delete_index_template
/**
* Delete an index template. The provided <index-template> may contain
* multiple template names separated by a comma. If multiple template names are
* specified then there is no wildcard support and the provided names should
* match completely with existing templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteIndexTemplate(DeleteIndexTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteIndexTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete an index template. The provided <index-template> may contain
* multiple template names separated by a comma. If multiple template names are
* specified then there is no wildcard support and the provided names should
* match completely with existing templates.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteIndexTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteIndexTemplate(
Function> fn) {
return deleteIndexTemplate(fn.apply(new DeleteIndexTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.delete_template
/**
* Deletes a legacy index template.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteTemplate(DeleteTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Deletes a legacy index template.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteTemplate(
Function> fn) {
return deleteTemplate(fn.apply(new DeleteTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.disk_usage
/**
* Analyzes the disk usage of each field of an index or data stream.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture diskUsage(DiskUsageRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DiskUsageRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Analyzes the disk usage of each field of an index or data stream.
*
* @param fn
* a function that initializes a builder to create the
* {@link DiskUsageRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture diskUsage(
Function> fn) {
return diskUsage(fn.apply(new DiskUsageRequest.Builder()).build());
}
// ----- Endpoint: indices.downsample
/**
* Aggregates a time series (TSDS) index and stores pre-computed statistical
* summaries (min
, max
, sum
,
* value_count
and avg
) for each metric field grouped
* by a configured time interval.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture downsample(DownsampleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DownsampleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Aggregates a time series (TSDS) index and stores pre-computed statistical
* summaries (min
, max
, sum
,
* value_count
and avg
) for each metric field grouped
* by a configured time interval.
*
* @param fn
* a function that initializes a builder to create the
* {@link DownsampleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture downsample(
Function> fn) {
return downsample(fn.apply(new DownsampleRequest.Builder()).build());
}
// ----- Endpoint: indices.exists
/**
* Check indices. Checks if one or more indices, index aliases, or data streams
* exist.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture exists(ExistsRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) ExistsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check indices. Checks if one or more indices, index aliases, or data streams
* exist.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture exists(
Function> fn) {
return exists(fn.apply(new ExistsRequest.Builder()).build());
}
// ----- Endpoint: indices.exists_alias
/**
* Check aliases. Checks if one or more data stream or index aliases exist.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture existsAlias(ExistsAliasRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) ExistsAliasRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check aliases. Checks if one or more data stream or index aliases exist.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsAliasRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture existsAlias(
Function> fn) {
return existsAlias(fn.apply(new ExistsAliasRequest.Builder()).build());
}
// ----- Endpoint: indices.exists_index_template
/**
* Returns information about whether a particular index template exists.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture existsIndexTemplate(ExistsIndexTemplateRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) ExistsIndexTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about whether a particular index template exists.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsIndexTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture existsIndexTemplate(
Function> fn) {
return existsIndexTemplate(fn.apply(new ExistsIndexTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.exists_template
/**
* Check existence of index templates. Returns information about whether a
* particular index template exists.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture existsTemplate(ExistsTemplateRequest request) {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) ExistsTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check existence of index templates. Returns information about whether a
* particular index template exists.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture existsTemplate(
Function> fn) {
return existsTemplate(fn.apply(new ExistsTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.explain_data_lifecycle
/**
* Get the status for a data stream lifecycle. Retrieves information about an
* index or data stream’s current data stream lifecycle status, such as time
* since index creation, time since rollover, the lifecycle configuration
* managing the index, or any errors encountered during lifecycle execution.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture explainDataLifecycle(ExplainDataLifecycleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExplainDataLifecycleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get the status for a data stream lifecycle. Retrieves information about an
* index or data stream’s current data stream lifecycle status, such as time
* since index creation, time since rollover, the lifecycle configuration
* managing the index, or any errors encountered during lifecycle execution.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExplainDataLifecycleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture explainDataLifecycle(
Function> fn) {
return explainDataLifecycle(fn.apply(new ExplainDataLifecycleRequest.Builder()).build());
}
// ----- Endpoint: indices.field_usage_stats
/**
* Returns field usage information for each shard and field of an index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture fieldUsageStats(FieldUsageStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FieldUsageStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns field usage information for each shard and field of an index.
*
* @param fn
* a function that initializes a builder to create the
* {@link FieldUsageStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture fieldUsageStats(
Function> fn) {
return fieldUsageStats(fn.apply(new FieldUsageStatsRequest.Builder()).build());
}
// ----- Endpoint: indices.flush
/**
* Flushes one or more data streams or indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture flush(FlushRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FlushRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Flushes one or more data streams or indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link FlushRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture flush(
Function> fn) {
return flush(fn.apply(new FlushRequest.Builder()).build());
}
/**
* Flushes one or more data streams or indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture flush() {
return this.transport.performRequestAsync(new FlushRequest.Builder().build(), FlushRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.forcemerge
/**
* Performs the force merge operation on one or more indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture forcemerge(ForcemergeRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ForcemergeRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Performs the force merge operation on one or more indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link ForcemergeRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture forcemerge(
Function> fn) {
return forcemerge(fn.apply(new ForcemergeRequest.Builder()).build());
}
/**
* Performs the force merge operation on one or more indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture forcemerge() {
return this.transport.performRequestAsync(new ForcemergeRequest.Builder().build(), ForcemergeRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.get
/**
* Get index information. Returns information about one or more indices. For
* data streams, the API returns information about the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture get(GetIndexRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetIndexRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get index information. Returns information about one or more indices. For
* data streams, the API returns information about the stream’s backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetIndexRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture get(
Function> fn) {
return get(fn.apply(new GetIndexRequest.Builder()).build());
}
// ----- Endpoint: indices.get_alias
/**
* Get aliases. Retrieves information for one or more data stream or index
* aliases.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getAlias(GetAliasRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetAliasRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get aliases. Retrieves information for one or more data stream or index
* aliases.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetAliasRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getAlias(
Function> fn) {
return getAlias(fn.apply(new GetAliasRequest.Builder()).build());
}
/**
* Get aliases. Retrieves information for one or more data stream or index
* aliases.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getAlias() {
return this.transport.performRequestAsync(new GetAliasRequest.Builder().build(), GetAliasRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.get_data_lifecycle
/**
* Get data stream lifecycles. Retrieves the data stream lifecycle configuration
* of one or more data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataLifecycle(GetDataLifecycleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataLifecycleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data stream lifecycles. Retrieves the data stream lifecycle configuration
* of one or more data streams.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataLifecycleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDataLifecycle(
Function> fn) {
return getDataLifecycle(fn.apply(new GetDataLifecycleRequest.Builder()).build());
}
// ----- Endpoint: indices.get_data_stream
/**
* Get data streams. Retrieves information about one or more data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataStream(GetDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data streams. Retrieves information about one or more data streams.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDataStream(
Function> fn) {
return getDataStream(fn.apply(new GetDataStreamRequest.Builder()).build());
}
/**
* Get data streams. Retrieves information about one or more data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataStream() {
return this.transport.performRequestAsync(new GetDataStreamRequest.Builder().build(),
GetDataStreamRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.get_field_mapping
/**
* Get mapping definitions. Retrieves mapping definitions for one or more
* fields. For data streams, the API retrieves field mappings for the stream’s
* backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getFieldMapping(GetFieldMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetFieldMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get mapping definitions. Retrieves mapping definitions for one or more
* fields. For data streams, the API retrieves field mappings for the stream’s
* backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetFieldMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getFieldMapping(
Function> fn) {
return getFieldMapping(fn.apply(new GetFieldMappingRequest.Builder()).build());
}
// ----- Endpoint: indices.get_index_template
/**
* Get index templates. Returns information about one or more index templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getIndexTemplate(GetIndexTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetIndexTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get index templates. Returns information about one or more index templates.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetIndexTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getIndexTemplate(
Function> fn) {
return getIndexTemplate(fn.apply(new GetIndexTemplateRequest.Builder()).build());
}
/**
* Get index templates. Returns information about one or more index templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getIndexTemplate() {
return this.transport.performRequestAsync(new GetIndexTemplateRequest.Builder().build(),
GetIndexTemplateRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.get_mapping
/**
* Get mapping definitions. Retrieves mapping definitions for one or more
* indices. For data streams, the API retrieves mappings for the stream’s
* backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getMapping(GetMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get mapping definitions. Retrieves mapping definitions for one or more
* indices. For data streams, the API retrieves mappings for the stream’s
* backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getMapping(
Function> fn) {
return getMapping(fn.apply(new GetMappingRequest.Builder()).build());
}
/**
* Get mapping definitions. Retrieves mapping definitions for one or more
* indices. For data streams, the API retrieves mappings for the stream’s
* backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getMapping() {
return this.transport.performRequestAsync(new GetMappingRequest.Builder().build(), GetMappingRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.get_settings
/**
* Get index settings. Returns setting information for one or more indices. For
* data streams, returns setting information for the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getSettings(GetIndicesSettingsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetIndicesSettingsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get index settings. Returns setting information for one or more indices. For
* data streams, returns setting information for the stream’s backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetIndicesSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getSettings(
Function> fn) {
return getSettings(fn.apply(new GetIndicesSettingsRequest.Builder()).build());
}
/**
* Get index settings. Returns setting information for one or more indices. For
* data streams, returns setting information for the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getSettings() {
return this.transport.performRequestAsync(new GetIndicesSettingsRequest.Builder().build(),
GetIndicesSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.get_template
/**
* Get index templates. Retrieves information about one or more index templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTemplate(GetTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get index templates. Retrieves information about one or more index templates.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getTemplate(
Function> fn) {
return getTemplate(fn.apply(new GetTemplateRequest.Builder()).build());
}
/**
* Get index templates. Retrieves information about one or more index templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTemplate() {
return this.transport.performRequestAsync(new GetTemplateRequest.Builder().build(),
GetTemplateRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.migrate_to_data_stream
/**
* Convert an index alias to a data stream. Converts an index alias to a data
* stream. You must have a matching index template that is data stream enabled.
* The alias must meet the following criteria: The alias must have a write
* index; All indices for the alias must have a @timestamp
field
* mapping of a date
or date_nanos
field type; The
* alias must not have any filters; The alias must not use custom routing. If
* successful, the request removes the alias and creates a data stream with the
* same name. The indices for the alias become hidden backing indices for the
* stream. The write index for the alias becomes the write index for the stream.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture migrateToDataStream(MigrateToDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MigrateToDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Convert an index alias to a data stream. Converts an index alias to a data
* stream. You must have a matching index template that is data stream enabled.
* The alias must meet the following criteria: The alias must have a write
* index; All indices for the alias must have a @timestamp
field
* mapping of a date
or date_nanos
field type; The
* alias must not have any filters; The alias must not use custom routing. If
* successful, the request removes the alias and creates a data stream with the
* same name. The indices for the alias become hidden backing indices for the
* stream. The write index for the alias becomes the write index for the stream.
*
* @param fn
* a function that initializes a builder to create the
* {@link MigrateToDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture migrateToDataStream(
Function> fn) {
return migrateToDataStream(fn.apply(new MigrateToDataStreamRequest.Builder()).build());
}
// ----- Endpoint: indices.modify_data_stream
/**
* Update data streams. Performs one or more data stream modification actions in
* a single atomic operation.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture modifyDataStream(ModifyDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ModifyDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update data streams. Performs one or more data stream modification actions in
* a single atomic operation.
*
* @param fn
* a function that initializes a builder to create the
* {@link ModifyDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture modifyDataStream(
Function> fn) {
return modifyDataStream(fn.apply(new ModifyDataStreamRequest.Builder()).build());
}
// ----- Endpoint: indices.open
/**
* Opens a closed index. For data streams, the API opens any closed backing
* indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture open(OpenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) OpenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Opens a closed index. For data streams, the API opens any closed backing
* indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link OpenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture open(Function> fn) {
return open(fn.apply(new OpenRequest.Builder()).build());
}
// ----- Endpoint: indices.promote_data_stream
/**
* Promotes a data stream from a replicated data stream managed by CCR to a
* regular data stream
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture promoteDataStream(PromoteDataStreamRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PromoteDataStreamRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Promotes a data stream from a replicated data stream managed by CCR to a
* regular data stream
*
* @param fn
* a function that initializes a builder to create the
* {@link PromoteDataStreamRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture promoteDataStream(
Function> fn) {
return promoteDataStream(fn.apply(new PromoteDataStreamRequest.Builder()).build());
}
// ----- Endpoint: indices.put_alias
/**
* Create or update an alias. Adds a data stream or index to an alias.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putAlias(PutAliasRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutAliasRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update an alias. Adds a data stream or index to an alias.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutAliasRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putAlias(
Function> fn) {
return putAlias(fn.apply(new PutAliasRequest.Builder()).build());
}
// ----- Endpoint: indices.put_data_lifecycle
/**
* Update data stream lifecycles. Update the data stream lifecycle of the
* specified data streams.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putDataLifecycle(PutDataLifecycleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutDataLifecycleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update data stream lifecycles. Update the data stream lifecycle of the
* specified data streams.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutDataLifecycleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putDataLifecycle(
Function> fn) {
return putDataLifecycle(fn.apply(new PutDataLifecycleRequest.Builder()).build());
}
// ----- Endpoint: indices.put_index_template
/**
* Create or update an index template. Index templates define settings,
* mappings, and aliases that can be applied automatically to new indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putIndexTemplate(PutIndexTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutIndexTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update an index template. Index templates define settings,
* mappings, and aliases that can be applied automatically to new indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutIndexTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putIndexTemplate(
Function> fn) {
return putIndexTemplate(fn.apply(new PutIndexTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.put_mapping
/**
* Update field mappings. Adds new fields to an existing data stream or index.
* You can also use this API to change the search settings of existing fields.
* For data streams, these changes are applied to all backing indices by
* default.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putMapping(PutMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update field mappings. Adds new fields to an existing data stream or index.
* You can also use this API to change the search settings of existing fields.
* For data streams, these changes are applied to all backing indices by
* default.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putMapping(
Function> fn) {
return putMapping(fn.apply(new PutMappingRequest.Builder()).build());
}
// ----- Endpoint: indices.put_settings
/**
* Update index settings. Changes dynamic index settings in real time. For data
* streams, index setting changes are applied to all backing indices by default.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putSettings(PutIndicesSettingsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutIndicesSettingsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Update index settings. Changes dynamic index settings in real time. For data
* streams, index setting changes are applied to all backing indices by default.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutIndicesSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putSettings(
Function> fn) {
return putSettings(fn.apply(new PutIndicesSettingsRequest.Builder()).build());
}
/**
* Update index settings. Changes dynamic index settings in real time. For data
* streams, index setting changes are applied to all backing indices by default.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putSettings() {
return this.transport.performRequestAsync(new PutIndicesSettingsRequest.Builder().build(),
PutIndicesSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: indices.put_template
/**
* Create or update an index template. Index templates define settings,
* mappings, and aliases that can be applied automatically to new indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putTemplate(PutTemplateRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTemplateRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create or update an index template. Index templates define settings,
* mappings, and aliases that can be applied automatically to new indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putTemplate(
Function> fn) {
return putTemplate(fn.apply(new PutTemplateRequest.Builder()).build());
}
// ----- Endpoint: indices.recovery
/**
* Returns information about ongoing and completed shard recoveries for one or
* more indices. For data streams, the API returns information for the stream’s
* backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery(RecoveryRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RecoveryRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about ongoing and completed shard recoveries for one or
* more indices. For data streams, the API returns information for the stream’s
* backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link RecoveryRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture recovery(
Function> fn) {
return recovery(fn.apply(new RecoveryRequest.Builder()).build());
}
/**
* Returns information about ongoing and completed shard recoveries for one or
* more indices. For data streams, the API returns information for the stream’s
* backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery() {
return this.transport.performRequestAsync(new RecoveryRequest.Builder().build(), RecoveryRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.refresh
/**
* Refresh an index. A refresh makes recent operations performed on one or more
* indices available for search. For data streams, the API runs the refresh
* operation on the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture refresh(RefreshRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RefreshRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Refresh an index. A refresh makes recent operations performed on one or more
* indices available for search. For data streams, the API runs the refresh
* operation on the stream’s backing indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link RefreshRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture refresh(
Function> fn) {
return refresh(fn.apply(new RefreshRequest.Builder()).build());
}
/**
* Refresh an index. A refresh makes recent operations performed on one or more
* indices available for search. For data streams, the API runs the refresh
* operation on the stream’s backing indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture refresh() {
return this.transport.performRequestAsync(new RefreshRequest.Builder().build(), RefreshRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: indices.reload_search_analyzers
/**
* Reloads an index's search analyzers and their resources.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture reloadSearchAnalyzers(
ReloadSearchAnalyzersRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ReloadSearchAnalyzersRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Reloads an index's search analyzers and their resources.
*
* @param fn
* a function that initializes a builder to create the
* {@link ReloadSearchAnalyzersRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture reloadSearchAnalyzers(
Function> fn) {
return reloadSearchAnalyzers(fn.apply(new ReloadSearchAnalyzersRequest.Builder()).build());
}
// ----- Endpoint: indices.resolve_cluster
/**
* Resolves the specified index expressions to return information about each
* cluster, including the local cluster, if included. Multiple patterns and
* remote clusters are supported.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture resolveCluster(ResolveClusterRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResolveClusterRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Resolves the specified index expressions to return information about each
* cluster, including the local cluster, if included. Multiple patterns and
* remote clusters are supported.
*
* @param fn
* a function that initializes a builder to create the
* {@link ResolveClusterRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture resolveCluster(
Function> fn) {
return resolveCluster(fn.apply(new ResolveClusterRequest.Builder()).build());
}
// ----- Endpoint: indices.resolve_index
/**
* Resolves the specified name(s) and/or index patterns for indices, aliases,
* and data streams. Multiple patterns and remote clusters are supported.
*
* @see