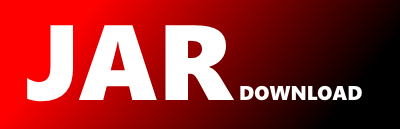
co.elastic.clients.elasticsearch.indices.ValidateQueryRequest Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.indices;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.elasticsearch._types.ExpandWildcard;
import co.elastic.clients.elasticsearch._types.RequestBase;
import co.elastic.clients.elasticsearch._types.query_dsl.Operator;
import co.elastic.clients.elasticsearch._types.query_dsl.Query;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.endpoints.SimpleEndpoint;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import jakarta.json.stream.JsonGenerator;
import java.lang.Boolean;
import java.lang.String;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: indices.validate_query.Request
/**
* Validate a query. Validates a query without running it.
*
* @see API
* specification
*/
@JsonpDeserializable
public class ValidateQueryRequest extends RequestBase implements JsonpSerializable {
@Nullable
private final Boolean allShards;
@Nullable
private final Boolean allowNoIndices;
@Nullable
private final Boolean analyzeWildcard;
@Nullable
private final String analyzer;
@Nullable
private final Operator defaultOperator;
@Nullable
private final String df;
private final List expandWildcards;
@Nullable
private final Boolean explain;
@Nullable
private final Boolean ignoreUnavailable;
private final List index;
@Nullable
private final Boolean lenient;
@Nullable
private final String q;
@Nullable
private final Query query;
@Nullable
private final Boolean rewrite;
// ---------------------------------------------------------------------------------------------
private ValidateQueryRequest(Builder builder) {
this.allShards = builder.allShards;
this.allowNoIndices = builder.allowNoIndices;
this.analyzeWildcard = builder.analyzeWildcard;
this.analyzer = builder.analyzer;
this.defaultOperator = builder.defaultOperator;
this.df = builder.df;
this.expandWildcards = ApiTypeHelper.unmodifiable(builder.expandWildcards);
this.explain = builder.explain;
this.ignoreUnavailable = builder.ignoreUnavailable;
this.index = ApiTypeHelper.unmodifiable(builder.index);
this.lenient = builder.lenient;
this.q = builder.q;
this.query = builder.query;
this.rewrite = builder.rewrite;
}
public static ValidateQueryRequest of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* If true
, the validation is executed on all shards instead of one
* random shard per index.
*
* API name: {@code all_shards}
*/
@Nullable
public final Boolean allShards() {
return this.allShards;
}
/**
* If false
, the request returns an error if any wildcard
* expression, index alias, or _all
value targets only missing or
* closed indices. This behavior applies even if the request targets other open
* indices.
*
* API name: {@code allow_no_indices}
*/
@Nullable
public final Boolean allowNoIndices() {
return this.allowNoIndices;
}
/**
* If true
, wildcard and prefix queries are analyzed.
*
* API name: {@code analyze_wildcard}
*/
@Nullable
public final Boolean analyzeWildcard() {
return this.analyzeWildcard;
}
/**
* Analyzer to use for the query string. This parameter can only be used when
* the q
query string parameter is specified.
*
* API name: {@code analyzer}
*/
@Nullable
public final String analyzer() {
return this.analyzer;
}
/**
* The default operator for query string query: AND
or
* OR
.
*
* API name: {@code default_operator}
*/
@Nullable
public final Operator defaultOperator() {
return this.defaultOperator;
}
/**
* Field to use as default where no field prefix is given in the query string.
* This parameter can only be used when the q
query string
* parameter is specified.
*
* API name: {@code df}
*/
@Nullable
public final String df() {
return this.df;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*/
public final List expandWildcards() {
return this.expandWildcards;
}
/**
* If true
, the response returns detailed information if an error
* has occurred.
*
* API name: {@code explain}
*/
@Nullable
public final Boolean explain() {
return this.explain;
}
/**
* If false
, the request returns an error if it targets a missing
* or closed index.
*
* API name: {@code ignore_unavailable}
*/
@Nullable
public final Boolean ignoreUnavailable() {
return this.ignoreUnavailable;
}
/**
* Comma-separated list of data streams, indices, and aliases to search.
* Supports wildcards (*
). To search all data streams or indices,
* omit this parameter or use *
or _all
.
*
* API name: {@code index}
*/
public final List index() {
return this.index;
}
/**
* If true
, format-based query failures (such as providing text to
* a numeric field) in the query string will be ignored.
*
* API name: {@code lenient}
*/
@Nullable
public final Boolean lenient() {
return this.lenient;
}
/**
* Query in the Lucene query string syntax.
*
* API name: {@code q}
*/
@Nullable
public final String q() {
return this.q;
}
/**
* Query in the Lucene query string syntax.
*
* API name: {@code query}
*/
@Nullable
public final Query query() {
return this.query;
}
/**
* If true
, returns a more detailed explanation showing the actual
* Lucene query that will be executed.
*
* API name: {@code rewrite}
*/
@Nullable
public final Boolean rewrite() {
return this.rewrite;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (this.query != null) {
generator.writeKey("query");
this.query.serialize(generator, mapper);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link ValidateQueryRequest}.
*/
public static class Builder extends RequestBase.AbstractBuilder
implements
ObjectBuilder {
@Nullable
private Boolean allShards;
@Nullable
private Boolean allowNoIndices;
@Nullable
private Boolean analyzeWildcard;
@Nullable
private String analyzer;
@Nullable
private Operator defaultOperator;
@Nullable
private String df;
@Nullable
private List expandWildcards;
@Nullable
private Boolean explain;
@Nullable
private Boolean ignoreUnavailable;
@Nullable
private List index;
@Nullable
private Boolean lenient;
@Nullable
private String q;
@Nullable
private Query query;
@Nullable
private Boolean rewrite;
/**
* If true
, the validation is executed on all shards instead of one
* random shard per index.
*
* API name: {@code all_shards}
*/
public final Builder allShards(@Nullable Boolean value) {
this.allShards = value;
return this;
}
/**
* If false
, the request returns an error if any wildcard
* expression, index alias, or _all
value targets only missing or
* closed indices. This behavior applies even if the request targets other open
* indices.
*
* API name: {@code allow_no_indices}
*/
public final Builder allowNoIndices(@Nullable Boolean value) {
this.allowNoIndices = value;
return this;
}
/**
* If true
, wildcard and prefix queries are analyzed.
*
* API name: {@code analyze_wildcard}
*/
public final Builder analyzeWildcard(@Nullable Boolean value) {
this.analyzeWildcard = value;
return this;
}
/**
* Analyzer to use for the query string. This parameter can only be used when
* the q
query string parameter is specified.
*
* API name: {@code analyzer}
*/
public final Builder analyzer(@Nullable String value) {
this.analyzer = value;
return this;
}
/**
* The default operator for query string query: AND
or
* OR
.
*
* API name: {@code default_operator}
*/
public final Builder defaultOperator(@Nullable Operator value) {
this.defaultOperator = value;
return this;
}
/**
* Field to use as default where no field prefix is given in the query string.
* This parameter can only be used when the q
query string
* parameter is specified.
*
* API name: {@code df}
*/
public final Builder df(@Nullable String value) {
this.df = value;
return this;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*
* Adds all elements of list
to expandWildcards
.
*/
public final Builder expandWildcards(List list) {
this.expandWildcards = _listAddAll(this.expandWildcards, list);
return this;
}
/**
* Type of index that wildcard patterns can match. If the request can target
* data streams, this argument determines whether wildcard expressions match
* hidden data streams. Supports comma-separated values, such as
* open,hidden
. Valid values are: all
,
* open
, closed
, hidden
,
* none
.
*
* API name: {@code expand_wildcards}
*
* Adds one or more values to expandWildcards
.
*/
public final Builder expandWildcards(ExpandWildcard value, ExpandWildcard... values) {
this.expandWildcards = _listAdd(this.expandWildcards, value, values);
return this;
}
/**
* If true
, the response returns detailed information if an error
* has occurred.
*
* API name: {@code explain}
*/
public final Builder explain(@Nullable Boolean value) {
this.explain = value;
return this;
}
/**
* If false
, the request returns an error if it targets a missing
* or closed index.
*
* API name: {@code ignore_unavailable}
*/
public final Builder ignoreUnavailable(@Nullable Boolean value) {
this.ignoreUnavailable = value;
return this;
}
/**
* Comma-separated list of data streams, indices, and aliases to search.
* Supports wildcards (*
). To search all data streams or indices,
* omit this parameter or use *
or _all
.
*
* API name: {@code index}
*
* Adds all elements of list
to index
.
*/
public final Builder index(List list) {
this.index = _listAddAll(this.index, list);
return this;
}
/**
* Comma-separated list of data streams, indices, and aliases to search.
* Supports wildcards (*
). To search all data streams or indices,
* omit this parameter or use *
or _all
.
*
* API name: {@code index}
*
* Adds one or more values to index
.
*/
public final Builder index(String value, String... values) {
this.index = _listAdd(this.index, value, values);
return this;
}
/**
* If true
, format-based query failures (such as providing text to
* a numeric field) in the query string will be ignored.
*
* API name: {@code lenient}
*/
public final Builder lenient(@Nullable Boolean value) {
this.lenient = value;
return this;
}
/**
* Query in the Lucene query string syntax.
*
* API name: {@code q}
*/
public final Builder q(@Nullable String value) {
this.q = value;
return this;
}
/**
* Query in the Lucene query string syntax.
*
* API name: {@code query}
*/
public final Builder query(@Nullable Query value) {
this.query = value;
return this;
}
/**
* Query in the Lucene query string syntax.
*
* API name: {@code query}
*/
public final Builder query(Function> fn) {
return this.query(fn.apply(new Query.Builder()).build());
}
/**
* If true
, returns a more detailed explanation showing the actual
* Lucene query that will be executed.
*
* API name: {@code rewrite}
*/
public final Builder rewrite(@Nullable Boolean value) {
this.rewrite = value;
return this;
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link ValidateQueryRequest}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public ValidateQueryRequest build() {
_checkSingleUse();
return new ValidateQueryRequest(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link ValidateQueryRequest}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer
.lazy(Builder::new, ValidateQueryRequest::setupValidateQueryRequestDeserializer);
protected static void setupValidateQueryRequestDeserializer(ObjectDeserializer op) {
op.add(Builder::query, Query._DESERIALIZER, "query");
}
// ---------------------------------------------------------------------------------------------
/**
* Endpoint "{@code indices.validate_query}".
*/
public static final Endpoint _ENDPOINT = new SimpleEndpoint<>(
"es/indices.validate_query",
// Request method
request -> {
return "POST";
},
// Request path
request -> {
final int _index = 1 << 0;
int propsSet = 0;
if (ApiTypeHelper.isDefined(request.index()))
propsSet |= _index;
if (propsSet == 0) {
StringBuilder buf = new StringBuilder();
buf.append("/_validate");
buf.append("/query");
return buf.toString();
}
if (propsSet == (_index)) {
StringBuilder buf = new StringBuilder();
buf.append("/");
SimpleEndpoint.pathEncode(request.index.stream().map(v -> v).collect(Collectors.joining(",")), buf);
buf.append("/_validate");
buf.append("/query");
return buf.toString();
}
throw SimpleEndpoint.noPathTemplateFound("path");
},
// Path parameters
request -> {
Map params = new HashMap<>();
final int _index = 1 << 0;
int propsSet = 0;
if (ApiTypeHelper.isDefined(request.index()))
propsSet |= _index;
if (propsSet == 0) {
}
if (propsSet == (_index)) {
params.put("index", request.index.stream().map(v -> v).collect(Collectors.joining(",")));
}
return params;
},
// Request parameters
request -> {
Map params = new HashMap<>();
if (request.explain != null) {
params.put("explain", String.valueOf(request.explain));
}
if (request.q != null) {
params.put("q", request.q);
}
if (request.df != null) {
params.put("df", request.df);
}
if (request.defaultOperator != null) {
params.put("default_operator", request.defaultOperator.jsonValue());
}
if (request.allShards != null) {
params.put("all_shards", String.valueOf(request.allShards));
}
if (ApiTypeHelper.isDefined(request.expandWildcards)) {
params.put("expand_wildcards",
request.expandWildcards.stream().map(v -> v.jsonValue()).collect(Collectors.joining(",")));
}
if (request.ignoreUnavailable != null) {
params.put("ignore_unavailable", String.valueOf(request.ignoreUnavailable));
}
if (request.allowNoIndices != null) {
params.put("allow_no_indices", String.valueOf(request.allowNoIndices));
}
if (request.analyzer != null) {
params.put("analyzer", request.analyzer);
}
if (request.analyzeWildcard != null) {
params.put("analyze_wildcard", String.valueOf(request.analyzeWildcard));
}
if (request.lenient != null) {
params.put("lenient", String.valueOf(request.lenient));
}
if (request.rewrite != null) {
params.put("rewrite", String.valueOf(request.rewrite));
}
return params;
}, SimpleEndpoint.emptyMap(), true, ValidateQueryResponse._DESERIALIZER);
}