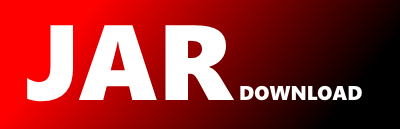
co.elastic.clients.elasticsearch.ml.DetectionRule Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.ml;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.JsonpUtils;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import co.elastic.clients.util.WithJsonObjectBuilderBase;
import jakarta.json.stream.JsonGenerator;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: ml._types.DetectionRule
/**
*
* @see API
* specification
*/
@JsonpDeserializable
public class DetectionRule implements JsonpSerializable {
private final List actions;
private final List conditions;
private final Map scope;
// ---------------------------------------------------------------------------------------------
private DetectionRule(Builder builder) {
this.actions = ApiTypeHelper.unmodifiable(builder.actions);
this.conditions = ApiTypeHelper.unmodifiable(builder.conditions);
this.scope = ApiTypeHelper.unmodifiable(builder.scope);
}
public static DetectionRule of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* The set of actions to be triggered when the rule applies. If more than one
* action is specified the effects of all actions are combined.
*
* API name: {@code actions}
*/
public final List actions() {
return this.actions;
}
/**
* An array of numeric conditions when the rule applies. A rule must either have
* a non-empty scope or at least one condition. Multiple conditions are combined
* together with a logical AND.
*
* API name: {@code conditions}
*/
public final List conditions() {
return this.conditions;
}
/**
* A scope of series where the rule applies. A rule must either have a non-empty
* scope or at least one condition. By default, the scope includes all series.
* Scoping is allowed for any of the fields that are also specified in
* by_field_name
, over_field_name
, or
* partition_field_name
.
*
* API name: {@code scope}
*/
public final Map scope() {
return this.scope;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (ApiTypeHelper.isDefined(this.actions)) {
generator.writeKey("actions");
generator.writeStartArray();
for (RuleAction item0 : this.actions) {
item0.serialize(generator, mapper);
}
generator.writeEnd();
}
if (ApiTypeHelper.isDefined(this.conditions)) {
generator.writeKey("conditions");
generator.writeStartArray();
for (RuleCondition item0 : this.conditions) {
item0.serialize(generator, mapper);
}
generator.writeEnd();
}
if (ApiTypeHelper.isDefined(this.scope)) {
generator.writeKey("scope");
generator.writeStartObject();
for (Map.Entry item0 : this.scope.entrySet()) {
generator.writeKey(item0.getKey());
item0.getValue().serialize(generator, mapper);
}
generator.writeEnd();
}
}
@Override
public String toString() {
return JsonpUtils.toString(this);
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link DetectionRule}.
*/
public static class Builder extends WithJsonObjectBuilderBase implements ObjectBuilder {
@Nullable
private List actions;
@Nullable
private List conditions;
@Nullable
private Map scope;
/**
* The set of actions to be triggered when the rule applies. If more than one
* action is specified the effects of all actions are combined.
*
* API name: {@code actions}
*
* Adds all elements of list
to actions
.
*/
public final Builder actions(List list) {
this.actions = _listAddAll(this.actions, list);
return this;
}
/**
* The set of actions to be triggered when the rule applies. If more than one
* action is specified the effects of all actions are combined.
*
* API name: {@code actions}
*
* Adds one or more values to actions
.
*/
public final Builder actions(RuleAction value, RuleAction... values) {
this.actions = _listAdd(this.actions, value, values);
return this;
}
/**
* An array of numeric conditions when the rule applies. A rule must either have
* a non-empty scope or at least one condition. Multiple conditions are combined
* together with a logical AND.
*
* API name: {@code conditions}
*
* Adds all elements of list
to conditions
.
*/
public final Builder conditions(List list) {
this.conditions = _listAddAll(this.conditions, list);
return this;
}
/**
* An array of numeric conditions when the rule applies. A rule must either have
* a non-empty scope or at least one condition. Multiple conditions are combined
* together with a logical AND.
*
* API name: {@code conditions}
*
* Adds one or more values to conditions
.
*/
public final Builder conditions(RuleCondition value, RuleCondition... values) {
this.conditions = _listAdd(this.conditions, value, values);
return this;
}
/**
* An array of numeric conditions when the rule applies. A rule must either have
* a non-empty scope or at least one condition. Multiple conditions are combined
* together with a logical AND.
*
* API name: {@code conditions}
*
* Adds a value to conditions
using a builder lambda.
*/
public final Builder conditions(Function> fn) {
return conditions(fn.apply(new RuleCondition.Builder()).build());
}
/**
* A scope of series where the rule applies. A rule must either have a non-empty
* scope or at least one condition. By default, the scope includes all series.
* Scoping is allowed for any of the fields that are also specified in
* by_field_name
, over_field_name
, or
* partition_field_name
.
*
* API name: {@code scope}
*
* Adds all entries of map
to scope
.
*/
public final Builder scope(Map map) {
this.scope = _mapPutAll(this.scope, map);
return this;
}
/**
* A scope of series where the rule applies. A rule must either have a non-empty
* scope or at least one condition. By default, the scope includes all series.
* Scoping is allowed for any of the fields that are also specified in
* by_field_name
, over_field_name
, or
* partition_field_name
.
*
* API name: {@code scope}
*
* Adds an entry to scope
.
*/
public final Builder scope(String key, FilterRef value) {
this.scope = _mapPut(this.scope, key, value);
return this;
}
/**
* A scope of series where the rule applies. A rule must either have a non-empty
* scope or at least one condition. By default, the scope includes all series.
* Scoping is allowed for any of the fields that are also specified in
* by_field_name
, over_field_name
, or
* partition_field_name
.
*
* API name: {@code scope}
*
* Adds an entry to scope
using a builder lambda.
*/
public final Builder scope(String key, Function> fn) {
return scope(key, fn.apply(new FilterRef.Builder()).build());
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link DetectionRule}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public DetectionRule build() {
_checkSingleUse();
return new DetectionRule(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link DetectionRule}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer.lazy(Builder::new,
DetectionRule::setupDetectionRuleDeserializer);
protected static void setupDetectionRuleDeserializer(ObjectDeserializer op) {
op.add(Builder::actions, JsonpDeserializer.arrayDeserializer(RuleAction._DESERIALIZER), "actions");
op.add(Builder::conditions, JsonpDeserializer.arrayDeserializer(RuleCondition._DESERIALIZER), "conditions");
op.add(Builder::scope, JsonpDeserializer.stringMapDeserializer(FilterRef._DESERIALIZER), "scope");
}
}