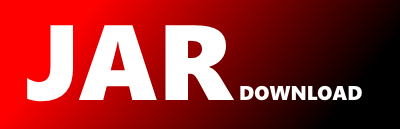
co.elastic.clients.elasticsearch.ml.ElasticsearchMlAsyncClient Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.ml;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.EndpointWithResponseMapperAttr;
import co.elastic.clients.util.ObjectBuilder;
import java.lang.reflect.Type;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the ml namespace.
*/
public class ElasticsearchMlAsyncClient extends ApiClient {
public ElasticsearchMlAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchMlAsyncClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchMlAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchMlAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: ml.clear_trained_model_deployment_cache
/**
* Clear trained model deployment cache. Cache will be cleared on all nodes
* where the trained model is assigned. A trained model deployment may have an
* inference cache enabled. As requests are handled by each allocated node,
* their responses may be cached on that individual node. Calling this API
* clears the caches without restarting the deployment.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearTrainedModelDeploymentCache(
ClearTrainedModelDeploymentCacheRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearTrainedModelDeploymentCacheRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear trained model deployment cache. Cache will be cleared on all nodes
* where the trained model is assigned. A trained model deployment may have an
* inference cache enabled. As requests are handled by each allocated node,
* their responses may be cached on that individual node. Calling this API
* clears the caches without restarting the deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearTrainedModelDeploymentCacheRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearTrainedModelDeploymentCache(
Function> fn) {
return clearTrainedModelDeploymentCache(
fn.apply(new ClearTrainedModelDeploymentCacheRequest.Builder()).build());
}
// ----- Endpoint: ml.close_job
/**
* Close anomaly detection jobs. A job can be opened and closed multiple times
* throughout its lifecycle. A closed job cannot receive data or perform
* analysis operations, but you can still explore and navigate results. When you
* close a job, it runs housekeeping tasks such as pruning the model history,
* flushing buffers, calculating final results and persisting the model
* snapshots. Depending upon the size of the job, it could take several minutes
* to close and the equivalent time to re-open. After it is closed, the job has
* a minimal overhead on the cluster except for maintaining its meta data.
* Therefore it is a best practice to close jobs that are no longer required to
* process data. If you close an anomaly detection job whose datafeed is
* running, the request first tries to stop the datafeed. This behavior is
* equivalent to calling stop datafeed API with the same timeout and force
* parameters as the close job request. When a datafeed that has a specified end
* date stops, it automatically closes its associated job.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture closeJob(CloseJobRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloseJobRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Close anomaly detection jobs. A job can be opened and closed multiple times
* throughout its lifecycle. A closed job cannot receive data or perform
* analysis operations, but you can still explore and navigate results. When you
* close a job, it runs housekeeping tasks such as pruning the model history,
* flushing buffers, calculating final results and persisting the model
* snapshots. Depending upon the size of the job, it could take several minutes
* to close and the equivalent time to re-open. After it is closed, the job has
* a minimal overhead on the cluster except for maintaining its meta data.
* Therefore it is a best practice to close jobs that are no longer required to
* process data. If you close an anomaly detection job whose datafeed is
* running, the request first tries to stop the datafeed. This behavior is
* equivalent to calling stop datafeed API with the same timeout and force
* parameters as the close job request. When a datafeed that has a specified end
* date stops, it automatically closes its associated job.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloseJobRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture closeJob(
Function> fn) {
return closeJob(fn.apply(new CloseJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar
/**
* Delete a calendar. Removes all scheduled events from a calendar, then deletes
* it.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteCalendar(DeleteCalendarRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a calendar. Removes all scheduled events from a calendar, then deletes
* it.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteCalendar(
Function> fn) {
return deleteCalendar(fn.apply(new DeleteCalendarRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_event
/**
* Delete events from a calendar.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteCalendarEvent(DeleteCalendarEventRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarEventRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete events from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarEventRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteCalendarEvent(
Function> fn) {
return deleteCalendarEvent(fn.apply(new DeleteCalendarEventRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_job
/**
* Delete anomaly jobs from a calendar.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteCalendarJob(DeleteCalendarJobRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarJobRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete anomaly jobs from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarJobRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteCalendarJob(
Function> fn) {
return deleteCalendarJob(fn.apply(new DeleteCalendarJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_data_frame_analytics
/**
* Delete a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteDataFrameAnalytics(
DeleteDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteDataFrameAnalytics(
Function> fn) {
return deleteDataFrameAnalytics(fn.apply(new DeleteDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_datafeed
/**
* Delete a datafeed.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteDatafeed(DeleteDatafeedRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDatafeedRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteDatafeed(
Function> fn) {
return deleteDatafeed(fn.apply(new DeleteDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_expired_data
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteExpiredData(DeleteExpiredDataRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteExpiredDataRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteExpiredDataRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteExpiredData(
Function> fn) {
return deleteExpiredData(fn.apply(new DeleteExpiredDataRequest.Builder()).build());
}
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteExpiredData() {
return this.transport.performRequestAsync(new DeleteExpiredDataRequest.Builder().build(),
DeleteExpiredDataRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.delete_filter
/**
* Delete a filter. If an anomaly detection job references the filter, you
* cannot delete the filter. You must update or delete the job before you can
* delete the filter.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteFilter(DeleteFilterRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteFilterRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a filter. If an anomaly detection job references the filter, you
* cannot delete the filter. You must update or delete the job before you can
* delete the filter.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteFilterRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteFilter(
Function> fn) {
return deleteFilter(fn.apply(new DeleteFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_forecast
/**
* Delete forecasts from a job. By default, forecasts are retained for 14 days.
* You can specify a different retention period with the expires_in
* parameter in the forecast jobs API. The delete forecast API enables you to
* delete one or more forecasts before they expire.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteForecast(DeleteForecastRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteForecastRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete forecasts from a job. By default, forecasts are retained for 14 days.
* You can specify a different retention period with the expires_in
* parameter in the forecast jobs API. The delete forecast API enables you to
* delete one or more forecasts before they expire.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteForecastRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteForecast(
Function> fn) {
return deleteForecast(fn.apply(new DeleteForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_job
/**
* Delete an anomaly detection job. All job configuration, model state and
* results are deleted. It is not currently possible to delete multiple jobs
* using wildcards or a comma separated list. If you delete a job that has a
* datafeed, the request first tries to delete the datafeed. This behavior is
* equivalent to calling the delete datafeed API with the same timeout and force
* parameters as the delete job request.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteJob(DeleteJobRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteJobRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete an anomaly detection job. All job configuration, model state and
* results are deleted. It is not currently possible to delete multiple jobs
* using wildcards or a comma separated list. If you delete a job that has a
* datafeed, the request first tries to delete the datafeed. This behavior is
* equivalent to calling the delete datafeed API with the same timeout and force
* parameters as the delete job request.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteJobRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteJob(
Function> fn) {
return deleteJob(fn.apply(new DeleteJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_model_snapshot
/**
* Delete a model snapshot. You cannot delete the active model snapshot. To
* delete that snapshot, first revert to a different one. To identify the active
* model snapshot, refer to the model_snapshot_id
in the results
* from the get jobs API.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteModelSnapshot(DeleteModelSnapshotRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteModelSnapshotRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a model snapshot. You cannot delete the active model snapshot. To
* delete that snapshot, first revert to a different one. To identify the active
* model snapshot, refer to the model_snapshot_id
in the results
* from the get jobs API.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteModelSnapshot(
Function> fn) {
return deleteModelSnapshot(fn.apply(new DeleteModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model
/**
* Delete an unreferenced trained model. The request deletes a trained inference
* model that is not referenced by an ingest pipeline.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteTrainedModel(DeleteTrainedModelRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete an unreferenced trained model. The request deletes a trained inference
* model that is not referenced by an ingest pipeline.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteTrainedModel(
Function> fn) {
return deleteTrainedModel(fn.apply(new DeleteTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model_alias
/**
* Delete a trained model alias. This API deletes an existing model alias that
* refers to a trained model. If the model alias is missing or refers to a model
* other than the one identified by the model_id
, this API returns
* an error.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteTrainedModelAlias(
DeleteTrainedModelAliasRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelAliasRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete a trained model alias. This API deletes an existing model alias that
* refers to a trained model. If the model alias is missing or refers to a model
* other than the one identified by the model_id
, this API returns
* an error.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelAliasRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteTrainedModelAlias(
Function> fn) {
return deleteTrainedModelAlias(fn.apply(new DeleteTrainedModelAliasRequest.Builder()).build());
}
// ----- Endpoint: ml.estimate_model_memory
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture estimateModelMemory(EstimateModelMemoryRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EstimateModelMemoryRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @param fn
* a function that initializes a builder to create the
* {@link EstimateModelMemoryRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture estimateModelMemory(
Function> fn) {
return estimateModelMemory(fn.apply(new EstimateModelMemoryRequest.Builder()).build());
}
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture estimateModelMemory() {
return this.transport.performRequestAsync(new EstimateModelMemoryRequest.Builder().build(),
EstimateModelMemoryRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.evaluate_data_frame
/**
* Evaluate data frame analytics. The API packages together commonly used
* evaluation metrics for various types of machine learning features. This has
* been designed for use on indexes created by data frame analytics. Evaluation
* requires both a ground truth field and an analytics result field to be
* present.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture evaluateDataFrame(EvaluateDataFrameRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EvaluateDataFrameRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evaluate data frame analytics. The API packages together commonly used
* evaluation metrics for various types of machine learning features. This has
* been designed for use on indexes created by data frame analytics. Evaluation
* requires both a ground truth field and an analytics result field to be
* present.
*
* @param fn
* a function that initializes a builder to create the
* {@link EvaluateDataFrameRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture evaluateDataFrame(
Function> fn) {
return evaluateDataFrame(fn.apply(new EvaluateDataFrameRequest.Builder()).build());
}
// ----- Endpoint: ml.explain_data_frame_analytics
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture explainDataFrameAnalytics(
ExplainDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExplainDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @param fn
* a function that initializes a builder to create the
* {@link ExplainDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture explainDataFrameAnalytics(
Function> fn) {
return explainDataFrameAnalytics(fn.apply(new ExplainDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture explainDataFrameAnalytics() {
return this.transport.performRequestAsync(new ExplainDataFrameAnalyticsRequest.Builder().build(),
ExplainDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.flush_job
/**
* Force buffered data to be processed. The flush jobs API is only applicable
* when sending data for analysis using the post data API. Depending on the
* content of the buffer, then it might additionally calculate new results. Both
* flush and close operations are similar, however the flush is more efficient
* if you are expecting to send more data for analysis. When flushing, the job
* remains open and is available to continue analyzing data. A close operation
* additionally prunes and persists the model state to disk and the job must be
* opened again before analyzing further data.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture flushJob(FlushJobRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FlushJobRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Force buffered data to be processed. The flush jobs API is only applicable
* when sending data for analysis using the post data API. Depending on the
* content of the buffer, then it might additionally calculate new results. Both
* flush and close operations are similar, however the flush is more efficient
* if you are expecting to send more data for analysis. When flushing, the job
* remains open and is available to continue analyzing data. A close operation
* additionally prunes and persists the model state to disk and the job must be
* opened again before analyzing further data.
*
* @param fn
* a function that initializes a builder to create the
* {@link FlushJobRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture flushJob(
Function> fn) {
return flushJob(fn.apply(new FlushJobRequest.Builder()).build());
}
// ----- Endpoint: ml.forecast
/**
* Predict future behavior of a time series.
*
* Forecasts are not supported for jobs that perform population analysis; an
* error occurs if you try to create a forecast for a job that has an
* over_field_name
in its configuration. Forcasts predict future
* behavior based on historical data.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture forecast(ForecastRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ForecastRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Predict future behavior of a time series.
*
* Forecasts are not supported for jobs that perform population analysis; an
* error occurs if you try to create a forecast for a job that has an
* over_field_name
in its configuration. Forcasts predict future
* behavior based on historical data.
*
* @param fn
* a function that initializes a builder to create the
* {@link ForecastRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture forecast(
Function> fn) {
return forecast(fn.apply(new ForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.get_buckets
/**
* Get anomaly detection job results for buckets. The API presents a
* chronological view of the records, grouped by bucket.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getBuckets(GetBucketsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetBucketsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for buckets. The API presents a
* chronological view of the records, grouped by bucket.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getBuckets(
Function> fn) {
return getBuckets(fn.apply(new GetBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendar_events
/**
* Get info about events in calendars.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getCalendarEvents(GetCalendarEventsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarEventsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get info about events in calendars.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getCalendarEvents(
Function> fn) {
return getCalendarEvents(fn.apply(new GetCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendars
/**
* Get calendar configuration info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getCalendars(GetCalendarsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get calendar configuration info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getCalendars(
Function> fn) {
return getCalendars(fn.apply(new GetCalendarsRequest.Builder()).build());
}
/**
* Get calendar configuration info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getCalendars() {
return this.transport.performRequestAsync(new GetCalendarsRequest.Builder().build(),
GetCalendarsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_categories
/**
* Get anomaly detection job results for categories.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getCategories(GetCategoriesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCategoriesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for categories.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCategoriesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getCategories(
Function> fn) {
return getCategories(fn.apply(new GetCategoriesRequest.Builder()).build());
}
// ----- Endpoint: ml.get_data_frame_analytics
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataFrameAnalytics(
GetDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDataFrameAnalytics(
Function> fn) {
return getDataFrameAnalytics(fn.apply(new GetDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataFrameAnalytics() {
return this.transport.performRequestAsync(new GetDataFrameAnalyticsRequest.Builder().build(),
GetDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_data_frame_analytics_stats
/**
* Get data frame analytics jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataFrameAnalyticsStats(
GetDataFrameAnalyticsStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get data frame analytics jobs usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDataFrameAnalyticsStats(
Function> fn) {
return getDataFrameAnalyticsStats(fn.apply(new GetDataFrameAnalyticsStatsRequest.Builder()).build());
}
/**
* Get data frame analytics jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDataFrameAnalyticsStats() {
return this.transport.performRequestAsync(new GetDataFrameAnalyticsStatsRequest.Builder().build(),
GetDataFrameAnalyticsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeed_stats
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDatafeedStats(GetDatafeedStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDatafeedStats(
Function> fn) {
return getDatafeedStats(fn.apply(new GetDatafeedStatsRequest.Builder()).build());
}
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDatafeedStats() {
return this.transport.performRequestAsync(new GetDatafeedStatsRequest.Builder().build(),
GetDatafeedStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeeds
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDatafeeds(GetDatafeedsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getDatafeeds(
Function> fn) {
return getDatafeeds(fn.apply(new GetDatafeedsRequest.Builder()).build());
}
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getDatafeeds() {
return this.transport.performRequestAsync(new GetDatafeedsRequest.Builder().build(),
GetDatafeedsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_filters
/**
* Get filters. You can get a single filter or all filters.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getFilters(GetFiltersRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetFiltersRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get filters. You can get a single filter or all filters.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetFiltersRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getFilters(
Function> fn) {
return getFilters(fn.apply(new GetFiltersRequest.Builder()).build());
}
/**
* Get filters. You can get a single filter or all filters.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getFilters() {
return this.transport.performRequestAsync(new GetFiltersRequest.Builder().build(), GetFiltersRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_influencers
/**
* Get anomaly detection job results for influencers. Influencers are the
* entities that have contributed to, or are to blame for, the anomalies.
* Influencer results are available only if an
* influencer_field_name
is specified in the job configuration.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getInfluencers(GetInfluencersRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetInfluencersRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for influencers. Influencers are the
* entities that have contributed to, or are to blame for, the anomalies.
* Influencer results are available only if an
* influencer_field_name
is specified in the job configuration.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetInfluencersRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getInfluencers(
Function> fn) {
return getInfluencers(fn.apply(new GetInfluencersRequest.Builder()).build());
}
// ----- Endpoint: ml.get_job_stats
/**
* Get anomaly detection jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getJobStats(GetJobStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection jobs usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getJobStats(
Function> fn) {
return getJobStats(fn.apply(new GetJobStatsRequest.Builder()).build());
}
/**
* Get anomaly detection jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getJobStats() {
return this.transport.performRequestAsync(new GetJobStatsRequest.Builder().build(),
GetJobStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_jobs
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getJobs(GetJobsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getJobs(
Function> fn) {
return getJobs(fn.apply(new GetJobsRequest.Builder()).build());
}
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getJobs() {
return this.transport.performRequestAsync(new GetJobsRequest.Builder().build(), GetJobsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_memory_stats
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getMemoryStats(GetMemoryStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetMemoryStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetMemoryStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getMemoryStats(
Function> fn) {
return getMemoryStats(fn.apply(new GetMemoryStatsRequest.Builder()).build());
}
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getMemoryStats() {
return this.transport.performRequestAsync(new GetMemoryStatsRequest.Builder().build(),
GetMemoryStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_model_snapshot_upgrade_stats
/**
* Get anomaly detection job model snapshot upgrade usage info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getModelSnapshotUpgradeStats(
GetModelSnapshotUpgradeStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetModelSnapshotUpgradeStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job model snapshot upgrade usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetModelSnapshotUpgradeStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getModelSnapshotUpgradeStats(
Function> fn) {
return getModelSnapshotUpgradeStats(fn.apply(new GetModelSnapshotUpgradeStatsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_model_snapshots
/**
* Get model snapshots info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getModelSnapshots(GetModelSnapshotsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetModelSnapshotsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get model snapshots info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetModelSnapshotsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getModelSnapshots(
Function> fn) {
return getModelSnapshots(fn.apply(new GetModelSnapshotsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_overall_buckets
/**
* Get overall bucket results.
*
* Retrievs overall bucket results that summarize the bucket results of multiple
* anomaly detection jobs.
*
* The overall_score
is calculated by combining the scores of all
* the buckets within the overall bucket span. First, the maximum
* anomaly_score
per anomaly detection job in the overall bucket is
* calculated. Then the top_n
of those scores are averaged to
* result in the overall_score
. This means that you can fine-tune
* the overall_score
so that it is more or less sensitive to the
* number of jobs that detect an anomaly at the same time. For example, if you
* set top_n
to 1
, the overall_score
is
* the maximum bucket score in the overall bucket. Alternatively, if you set
* top_n
to the number of jobs, the overall_score
is
* high only when all jobs detect anomalies in that overall bucket. If you set
* the bucket_span
parameter (to a value greater than its default),
* the overall_score
is the maximum overall_score
of
* the overall buckets that have a span equal to the jobs' largest bucket span.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getOverallBuckets(GetOverallBucketsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetOverallBucketsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get overall bucket results.
*
* Retrievs overall bucket results that summarize the bucket results of multiple
* anomaly detection jobs.
*
* The overall_score
is calculated by combining the scores of all
* the buckets within the overall bucket span. First, the maximum
* anomaly_score
per anomaly detection job in the overall bucket is
* calculated. Then the top_n
of those scores are averaged to
* result in the overall_score
. This means that you can fine-tune
* the overall_score
so that it is more or less sensitive to the
* number of jobs that detect an anomaly at the same time. For example, if you
* set top_n
to 1
, the overall_score
is
* the maximum bucket score in the overall bucket. Alternatively, if you set
* top_n
to the number of jobs, the overall_score
is
* high only when all jobs detect anomalies in that overall bucket. If you set
* the bucket_span
parameter (to a value greater than its default),
* the overall_score
is the maximum overall_score
of
* the overall buckets that have a span equal to the jobs' largest bucket span.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetOverallBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getOverallBuckets(
Function> fn) {
return getOverallBuckets(fn.apply(new GetOverallBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_records
/**
* Get anomaly records for an anomaly detection job. Records contain the
* detailed analytical results. They describe the anomalous activity that has
* been identified in the input data based on the detector configuration. There
* can be many anomaly records depending on the characteristics and size of the
* input data. In practice, there are often too many to be able to manually
* process them. The machine learning features therefore perform a sophisticated
* aggregation of the anomaly records into buckets. The number of record results
* depends on the number of anomalies found in each bucket, which relates to the
* number of time series being modeled and the number of detectors.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRecords(GetRecordsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRecordsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get anomaly records for an anomaly detection job. Records contain the
* detailed analytical results. They describe the anomalous activity that has
* been identified in the input data based on the detector configuration. There
* can be many anomaly records depending on the characteristics and size of the
* input data. In practice, there are often too many to be able to manually
* process them. The machine learning features therefore perform a sophisticated
* aggregation of the anomaly records into buckets. The number of record results
* depends on the number of anomalies found in each bucket, which relates to the
* number of time series being modeled and the number of detectors.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRecordsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getRecords(
Function> fn) {
return getRecords(fn.apply(new GetRecordsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_trained_models
/**
* Get trained model configuration info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTrainedModels(GetTrainedModelsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get trained model configuration info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getTrainedModels(
Function> fn) {
return getTrainedModels(fn.apply(new GetTrainedModelsRequest.Builder()).build());
}
/**
* Get trained model configuration info.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTrainedModels() {
return this.transport.performRequestAsync(new GetTrainedModelsRequest.Builder().build(),
GetTrainedModelsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_trained_models_stats
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTrainedModelsStats(
GetTrainedModelsStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getTrainedModelsStats(
Function> fn) {
return getTrainedModelsStats(fn.apply(new GetTrainedModelsStatsRequest.Builder()).build());
}
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getTrainedModelsStats() {
return this.transport.performRequestAsync(new GetTrainedModelsStatsRequest.Builder().build(),
GetTrainedModelsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.infer_trained_model
/**
* Evaluate a trained model.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture inferTrainedModel(InferTrainedModelRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InferTrainedModelRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Evaluate a trained model.
*
* @param fn
* a function that initializes a builder to create the
* {@link InferTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture inferTrainedModel(
Function> fn) {
return inferTrainedModel(fn.apply(new InferTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.info
/**
* Return ML defaults and limits. Returns defaults and limits used by machine
* learning. This endpoint is designed to be used by a user interface that needs
* to fully understand machine learning configurations where some options are
* not specified, meaning that the defaults should be used. This endpoint may be
* used to find out what those defaults are. It also provides information about
* the maximum size of machine learning jobs that could run in the current
* cluster configuration.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture info() {
return this.transport.performRequestAsync(MlInfoRequest._INSTANCE, MlInfoRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.open_job
/**
* Open anomaly detection jobs. An anomaly detection job must be opened to be
* ready to receive and analyze data. It can be opened and closed multiple times
* throughout its lifecycle. When you open a new job, it starts with an empty
* model. When you open an existing job, the most recent model state is
* automatically loaded. The job is ready to resume its analysis from where it
* left off, once new data is received.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture openJob(OpenJobRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) OpenJobRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Open anomaly detection jobs. An anomaly detection job must be opened to be
* ready to receive and analyze data. It can be opened and closed multiple times
* throughout its lifecycle. When you open a new job, it starts with an empty
* model. When you open an existing job, the most recent model state is
* automatically loaded. The job is ready to resume its analysis from where it
* left off, once new data is received.
*
* @param fn
* a function that initializes a builder to create the
* {@link OpenJobRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture openJob(
Function> fn) {
return openJob(fn.apply(new OpenJobRequest.Builder()).build());
}
// ----- Endpoint: ml.post_calendar_events
/**
* Add scheduled events to the calendar.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture postCalendarEvents(PostCalendarEventsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PostCalendarEventsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Add scheduled events to the calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture postCalendarEvents(
Function> fn) {
return postCalendarEvents(fn.apply(new PostCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.post_data
/**
* Send data to an anomaly detection job for analysis.
*
* IMPORTANT: For each job, data can be accepted from only a single connection
* at a time. It is not currently possible to post data to multiple jobs using
* wildcards or a comma-separated list.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture postData(PostDataRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint, PostDataResponse, ErrorResponse> endpoint = (JsonEndpoint, PostDataResponse, ErrorResponse>) PostDataRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Send data to an anomaly detection job for analysis.
*
* IMPORTANT: For each job, data can be accepted from only a single connection
* at a time. It is not currently possible to post data to multiple jobs using
* wildcards or a comma-separated list.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostDataRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture postData(
Function, ObjectBuilder>> fn) {
return postData(fn.apply(new PostDataRequest.Builder()).build());
}
// ----- Endpoint: ml.preview_data_frame_analytics
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture previewDataFrameAnalytics(
PreviewDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PreviewDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture previewDataFrameAnalytics(
Function> fn) {
return previewDataFrameAnalytics(fn.apply(new PreviewDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture previewDataFrameAnalytics() {
return this.transport.performRequestAsync(new PreviewDataFrameAnalyticsRequest.Builder().build(),
PreviewDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.preview_datafeed
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture> previewDatafeed(
PreviewDatafeedRequest request, Class tDocumentClass) {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewDatafeedRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:ml.preview_datafeed.Response.TDocument",
getDeserializer(tDocumentClass));
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture> previewDatafeed(
Function