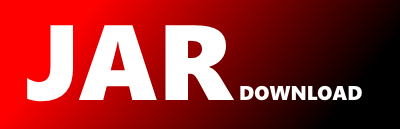
co.elastic.clients.elasticsearch.ml.ElasticsearchMlClient Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.ml;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.EndpointWithResponseMapperAttr;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the ml namespace.
*/
public class ElasticsearchMlClient extends ApiClient {
public ElasticsearchMlClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchMlClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchMlClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchMlClient(this.transport, transportOptions);
}
// ----- Endpoint: ml.clear_trained_model_deployment_cache
/**
* Clear trained model deployment cache. Cache will be cleared on all nodes
* where the trained model is assigned. A trained model deployment may have an
* inference cache enabled. As requests are handled by each allocated node,
* their responses may be cached on that individual node. Calling this API
* clears the caches without restarting the deployment.
*
* @see Documentation
* on elastic.co
*/
public ClearTrainedModelDeploymentCacheResponse clearTrainedModelDeploymentCache(
ClearTrainedModelDeploymentCacheRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearTrainedModelDeploymentCacheRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear trained model deployment cache. Cache will be cleared on all nodes
* where the trained model is assigned. A trained model deployment may have an
* inference cache enabled. As requests are handled by each allocated node,
* their responses may be cached on that individual node. Calling this API
* clears the caches without restarting the deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearTrainedModelDeploymentCacheRequest}
* @see Documentation
* on elastic.co
*/
public final ClearTrainedModelDeploymentCacheResponse clearTrainedModelDeploymentCache(
Function> fn)
throws IOException, ElasticsearchException {
return clearTrainedModelDeploymentCache(
fn.apply(new ClearTrainedModelDeploymentCacheRequest.Builder()).build());
}
// ----- Endpoint: ml.close_job
/**
* Close anomaly detection jobs. A job can be opened and closed multiple times
* throughout its lifecycle. A closed job cannot receive data or perform
* analysis operations, but you can still explore and navigate results. When you
* close a job, it runs housekeeping tasks such as pruning the model history,
* flushing buffers, calculating final results and persisting the model
* snapshots. Depending upon the size of the job, it could take several minutes
* to close and the equivalent time to re-open. After it is closed, the job has
* a minimal overhead on the cluster except for maintaining its meta data.
* Therefore it is a best practice to close jobs that are no longer required to
* process data. If you close an anomaly detection job whose datafeed is
* running, the request first tries to stop the datafeed. This behavior is
* equivalent to calling stop datafeed API with the same timeout and force
* parameters as the close job request. When a datafeed that has a specified end
* date stops, it automatically closes its associated job.
*
* @see Documentation
* on elastic.co
*/
public CloseJobResponse closeJob(CloseJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloseJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Close anomaly detection jobs. A job can be opened and closed multiple times
* throughout its lifecycle. A closed job cannot receive data or perform
* analysis operations, but you can still explore and navigate results. When you
* close a job, it runs housekeeping tasks such as pruning the model history,
* flushing buffers, calculating final results and persisting the model
* snapshots. Depending upon the size of the job, it could take several minutes
* to close and the equivalent time to re-open. After it is closed, the job has
* a minimal overhead on the cluster except for maintaining its meta data.
* Therefore it is a best practice to close jobs that are no longer required to
* process data. If you close an anomaly detection job whose datafeed is
* running, the request first tries to stop the datafeed. This behavior is
* equivalent to calling stop datafeed API with the same timeout and force
* parameters as the close job request. When a datafeed that has a specified end
* date stops, it automatically closes its associated job.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloseJobRequest}
* @see Documentation
* on elastic.co
*/
public final CloseJobResponse closeJob(Function> fn)
throws IOException, ElasticsearchException {
return closeJob(fn.apply(new CloseJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar
/**
* Delete a calendar. Removes all scheduled events from a calendar, then deletes
* it.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarResponse deleteCalendar(DeleteCalendarRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a calendar. Removes all scheduled events from a calendar, then deletes
* it.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarResponse deleteCalendar(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendar(fn.apply(new DeleteCalendarRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_event
/**
* Delete events from a calendar.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarEventResponse deleteCalendarEvent(DeleteCalendarEventRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarEventRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete events from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarEventRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarEventResponse deleteCalendarEvent(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendarEvent(fn.apply(new DeleteCalendarEventRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_job
/**
* Delete anomaly jobs from a calendar.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarJobResponse deleteCalendarJob(DeleteCalendarJobRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete anomaly jobs from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarJobRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarJobResponse deleteCalendarJob(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendarJob(fn.apply(new DeleteCalendarJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_data_frame_analytics
/**
* Delete a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public DeleteDataFrameAnalyticsResponse deleteDataFrameAnalytics(DeleteDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteDataFrameAnalyticsResponse deleteDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return deleteDataFrameAnalytics(fn.apply(new DeleteDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_datafeed
/**
* Delete a datafeed.
*
* @see Documentation
* on elastic.co
*/
public DeleteDatafeedResponse deleteDatafeed(DeleteDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteDatafeedResponse deleteDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return deleteDatafeed(fn.apply(new DeleteDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_expired_data
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @see Documentation
* on elastic.co
*/
public DeleteExpiredDataResponse deleteExpiredData(DeleteExpiredDataRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteExpiredDataRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteExpiredDataRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteExpiredDataResponse deleteExpiredData(
Function> fn)
throws IOException, ElasticsearchException {
return deleteExpiredData(fn.apply(new DeleteExpiredDataRequest.Builder()).build());
}
/**
* Delete expired ML data. Deletes all job results, model snapshots and forecast
* data that have exceeded their retention days period. Machine learning state
* documents that are not associated with any job are also deleted. You can
* limit the request to a single or set of anomaly detection jobs by using a job
* identifier, a group name, a comma-separated list of jobs, or a wildcard
* expression. You can delete expired data for all anomaly detection jobs by
* using _all, by specifying * as the <job_id>, or by omitting the
* <job_id>.
*
* @see Documentation
* on elastic.co
*/
public DeleteExpiredDataResponse deleteExpiredData() throws IOException, ElasticsearchException {
return this.transport.performRequest(new DeleteExpiredDataRequest.Builder().build(),
DeleteExpiredDataRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.delete_filter
/**
* Delete a filter. If an anomaly detection job references the filter, you
* cannot delete the filter. You must update or delete the job before you can
* delete the filter.
*
* @see Documentation
* on elastic.co
*/
public DeleteFilterResponse deleteFilter(DeleteFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a filter. If an anomaly detection job references the filter, you
* cannot delete the filter. You must update or delete the job before you can
* delete the filter.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteFilterRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteFilterResponse deleteFilter(
Function> fn)
throws IOException, ElasticsearchException {
return deleteFilter(fn.apply(new DeleteFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_forecast
/**
* Delete forecasts from a job. By default, forecasts are retained for 14 days.
* You can specify a different retention period with the expires_in
* parameter in the forecast jobs API. The delete forecast API enables you to
* delete one or more forecasts before they expire.
*
* @see Documentation
* on elastic.co
*/
public DeleteForecastResponse deleteForecast(DeleteForecastRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteForecastRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete forecasts from a job. By default, forecasts are retained for 14 days.
* You can specify a different retention period with the expires_in
* parameter in the forecast jobs API. The delete forecast API enables you to
* delete one or more forecasts before they expire.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteForecastRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteForecastResponse deleteForecast(
Function> fn)
throws IOException, ElasticsearchException {
return deleteForecast(fn.apply(new DeleteForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_job
/**
* Delete an anomaly detection job. All job configuration, model state and
* results are deleted. It is not currently possible to delete multiple jobs
* using wildcards or a comma separated list. If you delete a job that has a
* datafeed, the request first tries to delete the datafeed. This behavior is
* equivalent to calling the delete datafeed API with the same timeout and force
* parameters as the delete job request.
*
* @see Documentation
* on elastic.co
*/
public DeleteJobResponse deleteJob(DeleteJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete an anomaly detection job. All job configuration, model state and
* results are deleted. It is not currently possible to delete multiple jobs
* using wildcards or a comma separated list. If you delete a job that has a
* datafeed, the request first tries to delete the datafeed. This behavior is
* equivalent to calling the delete datafeed API with the same timeout and force
* parameters as the delete job request.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteJobRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteJobResponse deleteJob(Function> fn)
throws IOException, ElasticsearchException {
return deleteJob(fn.apply(new DeleteJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_model_snapshot
/**
* Delete a model snapshot. You cannot delete the active model snapshot. To
* delete that snapshot, first revert to a different one. To identify the active
* model snapshot, refer to the model_snapshot_id
in the results
* from the get jobs API.
*
* @see Documentation
* on elastic.co
*/
public DeleteModelSnapshotResponse deleteModelSnapshot(DeleteModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a model snapshot. You cannot delete the active model snapshot. To
* delete that snapshot, first revert to a different one. To identify the active
* model snapshot, refer to the model_snapshot_id
in the results
* from the get jobs API.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteModelSnapshotResponse deleteModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return deleteModelSnapshot(fn.apply(new DeleteModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model
/**
* Delete an unreferenced trained model. The request deletes a trained inference
* model that is not referenced by an ingest pipeline.
*
* @see Documentation
* on elastic.co
*/
public DeleteTrainedModelResponse deleteTrainedModel(DeleteTrainedModelRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete an unreferenced trained model. The request deletes a trained inference
* model that is not referenced by an ingest pipeline.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteTrainedModelResponse deleteTrainedModel(
Function> fn)
throws IOException, ElasticsearchException {
return deleteTrainedModel(fn.apply(new DeleteTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model_alias
/**
* Delete a trained model alias. This API deletes an existing model alias that
* refers to a trained model. If the model alias is missing or refers to a model
* other than the one identified by the model_id
, this API returns
* an error.
*
* @see Documentation
* on elastic.co
*/
public DeleteTrainedModelAliasResponse deleteTrainedModelAlias(DeleteTrainedModelAliasRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelAliasRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete a trained model alias. This API deletes an existing model alias that
* refers to a trained model. If the model alias is missing or refers to a model
* other than the one identified by the model_id
, this API returns
* an error.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelAliasRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteTrainedModelAliasResponse deleteTrainedModelAlias(
Function> fn)
throws IOException, ElasticsearchException {
return deleteTrainedModelAlias(fn.apply(new DeleteTrainedModelAliasRequest.Builder()).build());
}
// ----- Endpoint: ml.estimate_model_memory
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @see Documentation
* on elastic.co
*/
public EstimateModelMemoryResponse estimateModelMemory(EstimateModelMemoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EstimateModelMemoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @param fn
* a function that initializes a builder to create the
* {@link EstimateModelMemoryRequest}
* @see Documentation
* on elastic.co
*/
public final EstimateModelMemoryResponse estimateModelMemory(
Function> fn)
throws IOException, ElasticsearchException {
return estimateModelMemory(fn.apply(new EstimateModelMemoryRequest.Builder()).build());
}
/**
* Estimate job model memory usage. Makes an estimation of the memory usage for
* an anomaly detection job model. It is based on analysis configuration details
* for the job and cardinality estimates for the fields it references.
*
* @see Documentation
* on elastic.co
*/
public EstimateModelMemoryResponse estimateModelMemory() throws IOException, ElasticsearchException {
return this.transport.performRequest(new EstimateModelMemoryRequest.Builder().build(),
EstimateModelMemoryRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.evaluate_data_frame
/**
* Evaluate data frame analytics. The API packages together commonly used
* evaluation metrics for various types of machine learning features. This has
* been designed for use on indexes created by data frame analytics. Evaluation
* requires both a ground truth field and an analytics result field to be
* present.
*
* @see Documentation
* on elastic.co
*/
public EvaluateDataFrameResponse evaluateDataFrame(EvaluateDataFrameRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EvaluateDataFrameRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Evaluate data frame analytics. The API packages together commonly used
* evaluation metrics for various types of machine learning features. This has
* been designed for use on indexes created by data frame analytics. Evaluation
* requires both a ground truth field and an analytics result field to be
* present.
*
* @param fn
* a function that initializes a builder to create the
* {@link EvaluateDataFrameRequest}
* @see Documentation
* on elastic.co
*/
public final EvaluateDataFrameResponse evaluateDataFrame(
Function> fn)
throws IOException, ElasticsearchException {
return evaluateDataFrame(fn.apply(new EvaluateDataFrameRequest.Builder()).build());
}
// ----- Endpoint: ml.explain_data_frame_analytics
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @see Documentation
* on elastic.co
*/
public ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics(ExplainDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExplainDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @param fn
* a function that initializes a builder to create the
* {@link ExplainDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return explainDataFrameAnalytics(fn.apply(new ExplainDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Explain data frame analytics config. This API provides explanations for a
* data frame analytics config that either exists already or one that has not
* been created yet. The following explanations are provided:
*
* - which fields are included or not in the analysis and why,
* - how much memory is estimated to be required. The estimate can be used
* when deciding the appropriate value for model_memory_limit setting later on.
* If you have object fields or fields that are excluded via source filtering,
* they are not included in the explanation.
*
*
* @see Documentation
* on elastic.co
*/
public ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ExplainDataFrameAnalyticsRequest.Builder().build(),
ExplainDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.flush_job
/**
* Force buffered data to be processed. The flush jobs API is only applicable
* when sending data for analysis using the post data API. Depending on the
* content of the buffer, then it might additionally calculate new results. Both
* flush and close operations are similar, however the flush is more efficient
* if you are expecting to send more data for analysis. When flushing, the job
* remains open and is available to continue analyzing data. A close operation
* additionally prunes and persists the model state to disk and the job must be
* opened again before analyzing further data.
*
* @see Documentation
* on elastic.co
*/
public FlushJobResponse flushJob(FlushJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FlushJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Force buffered data to be processed. The flush jobs API is only applicable
* when sending data for analysis using the post data API. Depending on the
* content of the buffer, then it might additionally calculate new results. Both
* flush and close operations are similar, however the flush is more efficient
* if you are expecting to send more data for analysis. When flushing, the job
* remains open and is available to continue analyzing data. A close operation
* additionally prunes and persists the model state to disk and the job must be
* opened again before analyzing further data.
*
* @param fn
* a function that initializes a builder to create the
* {@link FlushJobRequest}
* @see Documentation
* on elastic.co
*/
public final FlushJobResponse flushJob(Function> fn)
throws IOException, ElasticsearchException {
return flushJob(fn.apply(new FlushJobRequest.Builder()).build());
}
// ----- Endpoint: ml.forecast
/**
* Predict future behavior of a time series.
*
* Forecasts are not supported for jobs that perform population analysis; an
* error occurs if you try to create a forecast for a job that has an
* over_field_name
in its configuration. Forcasts predict future
* behavior based on historical data.
*
* @see Documentation
* on elastic.co
*/
public ForecastResponse forecast(ForecastRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ForecastRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Predict future behavior of a time series.
*
* Forecasts are not supported for jobs that perform population analysis; an
* error occurs if you try to create a forecast for a job that has an
* over_field_name
in its configuration. Forcasts predict future
* behavior based on historical data.
*
* @param fn
* a function that initializes a builder to create the
* {@link ForecastRequest}
* @see Documentation
* on elastic.co
*/
public final ForecastResponse forecast(Function> fn)
throws IOException, ElasticsearchException {
return forecast(fn.apply(new ForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.get_buckets
/**
* Get anomaly detection job results for buckets. The API presents a
* chronological view of the records, grouped by bucket.
*
* @see Documentation
* on elastic.co
*/
public GetBucketsResponse getBuckets(GetBucketsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetBucketsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for buckets. The API presents a
* chronological view of the records, grouped by bucket.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final GetBucketsResponse getBuckets(Function> fn)
throws IOException, ElasticsearchException {
return getBuckets(fn.apply(new GetBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendar_events
/**
* Get info about events in calendars.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarEventsResponse getCalendarEvents(GetCalendarEventsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarEventsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get info about events in calendars.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final GetCalendarEventsResponse getCalendarEvents(
Function> fn)
throws IOException, ElasticsearchException {
return getCalendarEvents(fn.apply(new GetCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendars
/**
* Get calendar configuration info.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarsResponse getCalendars(GetCalendarsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get calendar configuration info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarsRequest}
* @see Documentation
* on elastic.co
*/
public final GetCalendarsResponse getCalendars(
Function> fn)
throws IOException, ElasticsearchException {
return getCalendars(fn.apply(new GetCalendarsRequest.Builder()).build());
}
/**
* Get calendar configuration info.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarsResponse getCalendars() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetCalendarsRequest.Builder().build(), GetCalendarsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_categories
/**
* Get anomaly detection job results for categories.
*
* @see Documentation
* on elastic.co
*/
public GetCategoriesResponse getCategories(GetCategoriesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCategoriesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for categories.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCategoriesRequest}
* @see Documentation
* on elastic.co
*/
public final GetCategoriesResponse getCategories(
Function> fn)
throws IOException, ElasticsearchException {
return getCategories(fn.apply(new GetCategoriesRequest.Builder()).build());
}
// ----- Endpoint: ml.get_data_frame_analytics
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsResponse getDataFrameAnalytics(GetDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDataFrameAnalyticsResponse getDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return getDataFrameAnalytics(fn.apply(new GetDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Get data frame analytics job configuration info. You can get information for
* multiple data frame analytics jobs in a single API request by using a
* comma-separated list of data frame analytics jobs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsResponse getDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDataFrameAnalyticsRequest.Builder().build(),
GetDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_data_frame_analytics_stats
/**
* Get data frame analytics jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats(GetDataFrameAnalyticsStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get data frame analytics jobs usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats(
Function> fn)
throws IOException, ElasticsearchException {
return getDataFrameAnalyticsStats(fn.apply(new GetDataFrameAnalyticsStatsRequest.Builder()).build());
}
/**
* Get data frame analytics jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDataFrameAnalyticsStatsRequest.Builder().build(),
GetDataFrameAnalyticsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeed_stats
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedStatsResponse getDatafeedStats(GetDatafeedStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDatafeedStatsResponse getDatafeedStats(
Function> fn)
throws IOException, ElasticsearchException {
return getDatafeedStats(fn.apply(new GetDatafeedStatsRequest.Builder()).build());
}
/**
* Get datafeeds usage info. You can get statistics for multiple datafeeds in a
* single API request by using a comma-separated list of datafeeds or a wildcard
* expression. You can get statistics for all datafeeds by using
* _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. If the datafeed is stopped, the only
* information you receive is the datafeed_id
and the
* state
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedStatsResponse getDatafeedStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDatafeedStatsRequest.Builder().build(),
GetDatafeedStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeeds
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedsResponse getDatafeeds(GetDatafeedsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDatafeedsResponse getDatafeeds(
Function> fn)
throws IOException, ElasticsearchException {
return getDatafeeds(fn.apply(new GetDatafeedsRequest.Builder()).build());
}
/**
* Get datafeeds configuration info. You can get information for multiple
* datafeeds in a single API request by using a comma-separated list of
* datafeeds or a wildcard expression. You can get information for all datafeeds
* by using _all
, by specifying *
as the
* <feed_id>
, or by omitting the
* <feed_id>
. This API returns a maximum of 10,000 datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedsResponse getDatafeeds() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDatafeedsRequest.Builder().build(), GetDatafeedsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_filters
/**
* Get filters. You can get a single filter or all filters.
*
* @see Documentation
* on elastic.co
*/
public GetFiltersResponse getFilters(GetFiltersRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetFiltersRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get filters. You can get a single filter or all filters.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetFiltersRequest}
* @see Documentation
* on elastic.co
*/
public final GetFiltersResponse getFilters(Function> fn)
throws IOException, ElasticsearchException {
return getFilters(fn.apply(new GetFiltersRequest.Builder()).build());
}
/**
* Get filters. You can get a single filter or all filters.
*
* @see Documentation
* on elastic.co
*/
public GetFiltersResponse getFilters() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetFiltersRequest.Builder().build(), GetFiltersRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_influencers
/**
* Get anomaly detection job results for influencers. Influencers are the
* entities that have contributed to, or are to blame for, the anomalies.
* Influencer results are available only if an
* influencer_field_name
is specified in the job configuration.
*
* @see Documentation
* on elastic.co
*/
public GetInfluencersResponse getInfluencers(GetInfluencersRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetInfluencersRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job results for influencers. Influencers are the
* entities that have contributed to, or are to blame for, the anomalies.
* Influencer results are available only if an
* influencer_field_name
is specified in the job configuration.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetInfluencersRequest}
* @see Documentation
* on elastic.co
*/
public final GetInfluencersResponse getInfluencers(
Function> fn)
throws IOException, ElasticsearchException {
return getInfluencers(fn.apply(new GetInfluencersRequest.Builder()).build());
}
// ----- Endpoint: ml.get_job_stats
/**
* Get anomaly detection jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public GetJobStatsResponse getJobStats(GetJobStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection jobs usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetJobStatsResponse getJobStats(
Function> fn)
throws IOException, ElasticsearchException {
return getJobStats(fn.apply(new GetJobStatsRequest.Builder()).build());
}
/**
* Get anomaly detection jobs usage info.
*
* @see Documentation
* on elastic.co
*/
public GetJobStatsResponse getJobStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetJobStatsRequest.Builder().build(), GetJobStatsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_jobs
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @see Documentation
* on elastic.co
*/
public GetJobsResponse getJobs(GetJobsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobsRequest}
* @see Documentation
* on elastic.co
*/
public final GetJobsResponse getJobs(Function> fn)
throws IOException, ElasticsearchException {
return getJobs(fn.apply(new GetJobsRequest.Builder()).build());
}
/**
* Get anomaly detection jobs configuration info. You can get information for
* multiple anomaly detection jobs in a single API request by using a group
* name, a comma-separated list of jobs, or a wildcard expression. You can get
* information for all anomaly detection jobs by using _all
, by
* specifying *
as the <job_id>
, or by omitting
* the <job_id>
.
*
* @see Documentation
* on elastic.co
*/
public GetJobsResponse getJobs() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetJobsRequest.Builder().build(), GetJobsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_memory_stats
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @see Documentation
* on elastic.co
*/
public GetMemoryStatsResponse getMemoryStats(GetMemoryStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetMemoryStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetMemoryStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetMemoryStatsResponse getMemoryStats(
Function> fn)
throws IOException, ElasticsearchException {
return getMemoryStats(fn.apply(new GetMemoryStatsRequest.Builder()).build());
}
/**
* Get machine learning memory usage info. Get information about how machine
* learning jobs and trained models are using memory, on each node, both within
* the JVM heap, and natively, outside of the JVM.
*
* @see Documentation
* on elastic.co
*/
public GetMemoryStatsResponse getMemoryStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetMemoryStatsRequest.Builder().build(),
GetMemoryStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_model_snapshot_upgrade_stats
/**
* Get anomaly detection job model snapshot upgrade usage info.
*
* @see Documentation
* on elastic.co
*/
public GetModelSnapshotUpgradeStatsResponse getModelSnapshotUpgradeStats(
GetModelSnapshotUpgradeStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetModelSnapshotUpgradeStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly detection job model snapshot upgrade usage info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetModelSnapshotUpgradeStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetModelSnapshotUpgradeStatsResponse getModelSnapshotUpgradeStats(
Function> fn)
throws IOException, ElasticsearchException {
return getModelSnapshotUpgradeStats(fn.apply(new GetModelSnapshotUpgradeStatsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_model_snapshots
/**
* Get model snapshots info.
*
* @see Documentation
* on elastic.co
*/
public GetModelSnapshotsResponse getModelSnapshots(GetModelSnapshotsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetModelSnapshotsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get model snapshots info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetModelSnapshotsRequest}
* @see Documentation
* on elastic.co
*/
public final GetModelSnapshotsResponse getModelSnapshots(
Function> fn)
throws IOException, ElasticsearchException {
return getModelSnapshots(fn.apply(new GetModelSnapshotsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_overall_buckets
/**
* Get overall bucket results.
*
* Retrievs overall bucket results that summarize the bucket results of multiple
* anomaly detection jobs.
*
* The overall_score
is calculated by combining the scores of all
* the buckets within the overall bucket span. First, the maximum
* anomaly_score
per anomaly detection job in the overall bucket is
* calculated. Then the top_n
of those scores are averaged to
* result in the overall_score
. This means that you can fine-tune
* the overall_score
so that it is more or less sensitive to the
* number of jobs that detect an anomaly at the same time. For example, if you
* set top_n
to 1
, the overall_score
is
* the maximum bucket score in the overall bucket. Alternatively, if you set
* top_n
to the number of jobs, the overall_score
is
* high only when all jobs detect anomalies in that overall bucket. If you set
* the bucket_span
parameter (to a value greater than its default),
* the overall_score
is the maximum overall_score
of
* the overall buckets that have a span equal to the jobs' largest bucket span.
*
* @see Documentation
* on elastic.co
*/
public GetOverallBucketsResponse getOverallBuckets(GetOverallBucketsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetOverallBucketsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get overall bucket results.
*
* Retrievs overall bucket results that summarize the bucket results of multiple
* anomaly detection jobs.
*
* The overall_score
is calculated by combining the scores of all
* the buckets within the overall bucket span. First, the maximum
* anomaly_score
per anomaly detection job in the overall bucket is
* calculated. Then the top_n
of those scores are averaged to
* result in the overall_score
. This means that you can fine-tune
* the overall_score
so that it is more or less sensitive to the
* number of jobs that detect an anomaly at the same time. For example, if you
* set top_n
to 1
, the overall_score
is
* the maximum bucket score in the overall bucket. Alternatively, if you set
* top_n
to the number of jobs, the overall_score
is
* high only when all jobs detect anomalies in that overall bucket. If you set
* the bucket_span
parameter (to a value greater than its default),
* the overall_score
is the maximum overall_score
of
* the overall buckets that have a span equal to the jobs' largest bucket span.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetOverallBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final GetOverallBucketsResponse getOverallBuckets(
Function> fn)
throws IOException, ElasticsearchException {
return getOverallBuckets(fn.apply(new GetOverallBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_records
/**
* Get anomaly records for an anomaly detection job. Records contain the
* detailed analytical results. They describe the anomalous activity that has
* been identified in the input data based on the detector configuration. There
* can be many anomaly records depending on the characteristics and size of the
* input data. In practice, there are often too many to be able to manually
* process them. The machine learning features therefore perform a sophisticated
* aggregation of the anomaly records into buckets. The number of record results
* depends on the number of anomalies found in each bucket, which relates to the
* number of time series being modeled and the number of detectors.
*
* @see Documentation
* on elastic.co
*/
public GetRecordsResponse getRecords(GetRecordsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRecordsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get anomaly records for an anomaly detection job. Records contain the
* detailed analytical results. They describe the anomalous activity that has
* been identified in the input data based on the detector configuration. There
* can be many anomaly records depending on the characteristics and size of the
* input data. In practice, there are often too many to be able to manually
* process them. The machine learning features therefore perform a sophisticated
* aggregation of the anomaly records into buckets. The number of record results
* depends on the number of anomalies found in each bucket, which relates to the
* number of time series being modeled and the number of detectors.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRecordsRequest}
* @see Documentation
* on elastic.co
*/
public final GetRecordsResponse getRecords(Function> fn)
throws IOException, ElasticsearchException {
return getRecords(fn.apply(new GetRecordsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_trained_models
/**
* Get trained model configuration info.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsResponse getTrainedModels(GetTrainedModelsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get trained model configuration info.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsRequest}
* @see Documentation
* on elastic.co
*/
public final GetTrainedModelsResponse getTrainedModels(
Function> fn)
throws IOException, ElasticsearchException {
return getTrainedModels(fn.apply(new GetTrainedModelsRequest.Builder()).build());
}
/**
* Get trained model configuration info.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsResponse getTrainedModels() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetTrainedModelsRequest.Builder().build(),
GetTrainedModelsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_trained_models_stats
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsStatsResponse getTrainedModelsStats(GetTrainedModelsStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetTrainedModelsStatsResponse getTrainedModelsStats(
Function> fn)
throws IOException, ElasticsearchException {
return getTrainedModelsStats(fn.apply(new GetTrainedModelsStatsRequest.Builder()).build());
}
/**
* Get trained models usage info. You can get usage information for multiple
* trained models in a single API request by using a comma-separated list of
* model IDs or a wildcard expression.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsStatsResponse getTrainedModelsStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetTrainedModelsStatsRequest.Builder().build(),
GetTrainedModelsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.infer_trained_model
/**
* Evaluate a trained model.
*
* @see Documentation
* on elastic.co
*/
public InferTrainedModelResponse inferTrainedModel(InferTrainedModelRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InferTrainedModelRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Evaluate a trained model.
*
* @param fn
* a function that initializes a builder to create the
* {@link InferTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final InferTrainedModelResponse inferTrainedModel(
Function> fn)
throws IOException, ElasticsearchException {
return inferTrainedModel(fn.apply(new InferTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.info
/**
* Return ML defaults and limits. Returns defaults and limits used by machine
* learning. This endpoint is designed to be used by a user interface that needs
* to fully understand machine learning configurations where some options are
* not specified, meaning that the defaults should be used. This endpoint may be
* used to find out what those defaults are. It also provides information about
* the maximum size of machine learning jobs that could run in the current
* cluster configuration.
*
* @see Documentation
* on elastic.co
*/
public MlInfoResponse info() throws IOException, ElasticsearchException {
return this.transport.performRequest(MlInfoRequest._INSTANCE, MlInfoRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.open_job
/**
* Open anomaly detection jobs. An anomaly detection job must be opened to be
* ready to receive and analyze data. It can be opened and closed multiple times
* throughout its lifecycle. When you open a new job, it starts with an empty
* model. When you open an existing job, the most recent model state is
* automatically loaded. The job is ready to resume its analysis from where it
* left off, once new data is received.
*
* @see Documentation
* on elastic.co
*/
public OpenJobResponse openJob(OpenJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) OpenJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Open anomaly detection jobs. An anomaly detection job must be opened to be
* ready to receive and analyze data. It can be opened and closed multiple times
* throughout its lifecycle. When you open a new job, it starts with an empty
* model. When you open an existing job, the most recent model state is
* automatically loaded. The job is ready to resume its analysis from where it
* left off, once new data is received.
*
* @param fn
* a function that initializes a builder to create the
* {@link OpenJobRequest}
* @see Documentation
* on elastic.co
*/
public final OpenJobResponse openJob(Function> fn)
throws IOException, ElasticsearchException {
return openJob(fn.apply(new OpenJobRequest.Builder()).build());
}
// ----- Endpoint: ml.post_calendar_events
/**
* Add scheduled events to the calendar.
*
* @see Documentation
* on elastic.co
*/
public PostCalendarEventsResponse postCalendarEvents(PostCalendarEventsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PostCalendarEventsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Add scheduled events to the calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final PostCalendarEventsResponse postCalendarEvents(
Function> fn)
throws IOException, ElasticsearchException {
return postCalendarEvents(fn.apply(new PostCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.post_data
/**
* Send data to an anomaly detection job for analysis.
*
* IMPORTANT: For each job, data can be accepted from only a single connection
* at a time. It is not currently possible to post data to multiple jobs using
* wildcards or a comma-separated list.
*
* @see Documentation
* on elastic.co
*/
public PostDataResponse postData(PostDataRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint, PostDataResponse, ErrorResponse> endpoint = (JsonEndpoint, PostDataResponse, ErrorResponse>) PostDataRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Send data to an anomaly detection job for analysis.
*
* IMPORTANT: For each job, data can be accepted from only a single connection
* at a time. It is not currently possible to post data to multiple jobs using
* wildcards or a comma-separated list.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostDataRequest}
* @see Documentation
* on elastic.co
*/
public final PostDataResponse postData(
Function, ObjectBuilder>> fn)
throws IOException, ElasticsearchException {
return postData(fn.apply(new PostDataRequest.Builder()).build());
}
// ----- Endpoint: ml.preview_data_frame_analytics
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics(PreviewDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PreviewDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return previewDataFrameAnalytics(fn.apply(new PreviewDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Preview features used by data frame analytics. Previews the extracted
* features used by a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PreviewDataFrameAnalyticsRequest.Builder().build(),
PreviewDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.preview_datafeed
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @see Documentation
* on elastic.co
*/
public PreviewDatafeedResponse previewDatafeed(PreviewDatafeedRequest request,
Class tDocumentClass) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewDatafeedRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:ml.preview_datafeed.Response.TDocument",
getDeserializer(tDocumentClass));
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final PreviewDatafeedResponse previewDatafeed(
Function> fn,
Class tDocumentClass) throws IOException, ElasticsearchException {
return previewDatafeed(fn.apply(new PreviewDatafeedRequest.Builder()).build(), tDocumentClass);
}
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @see Documentation
* on elastic.co
*/
public PreviewDatafeedResponse previewDatafeed(PreviewDatafeedRequest request,
Type tDocumentType) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewDatafeedRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:ml.preview_datafeed.Response.TDocument",
getDeserializer(tDocumentType));
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Preview a datafeed. This API returns the first "page" of search
* results from a datafeed. You can preview an existing datafeed or provide
* configuration details for a datafeed and anomaly detection job in the API.
* The preview shows the structure of the data that will be passed to the
* anomaly detection engine. IMPORTANT: When Elasticsearch security features are
* enabled, the preview uses the credentials of the user that called the API.
* However, when the datafeed starts it uses the roles of the last user that
* created or updated the datafeed. To get a preview that accurately reflects
* the behavior of the datafeed, use the appropriate credentials. You can also
* use secondary authorization headers to supply the credentials.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final PreviewDatafeedResponse previewDatafeed(
Function> fn, Type tDocumentType)
throws IOException, ElasticsearchException {
return previewDatafeed(fn.apply(new PreviewDatafeedRequest.Builder()).build(), tDocumentType);
}
// ----- Endpoint: ml.put_calendar
/**
* Create a calendar.
*
* @see Documentation
* on elastic.co
*/
public PutCalendarResponse putCalendar(PutCalendarRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutCalendarRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutCalendarRequest}
* @see Documentation
* on elastic.co
*/
public final PutCalendarResponse putCalendar(
Function> fn)
throws IOException, ElasticsearchException {
return putCalendar(fn.apply(new PutCalendarRequest.Builder()).build());
}
// ----- Endpoint: ml.put_calendar_job
/**
* Add anomaly detection job to calendar.
*
* @see Documentation
* on elastic.co
*/
public PutCalendarJobResponse putCalendarJob(PutCalendarJobRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutCalendarJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Add anomaly detection job to calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutCalendarJobRequest}
* @see Documentation
* on elastic.co
*/
public final PutCalendarJobResponse putCalendarJob(
Function> fn)
throws IOException, ElasticsearchException {
return putCalendarJob(fn.apply(new PutCalendarJobRequest.Builder()).build());
}
// ----- Endpoint: ml.put_data_frame_analytics
/**
* Create a data frame analytics job. This API creates a data frame analytics
* job that performs an analysis on the source indices and stores the outcome in
* a destination index.
*
* @see Documentation
* on elastic.co
*/
public PutDataFrameAnalyticsResponse putDataFrameAnalytics(PutDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a data frame analytics job. This API creates a data frame analytics
* job that performs an analysis on the source indices and stores the outcome in
* a destination index.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final PutDataFrameAnalyticsResponse putDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return putDataFrameAnalytics(fn.apply(new PutDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.put_datafeed
/**
* Create a datafeed. Datafeeds retrieve data from Elasticsearch for analysis by
* an anomaly detection job. You can associate only one datafeed with each
* anomaly detection job. The datafeed contains a query that runs at a defined
* interval (frequency
). If you are concerned about delayed data,
* you can add a delay
* (query_delay') at each interval. When Elasticsearch security features are enabled, your datafeed remembers which roles the user who created it had at the time of creation and runs the query using those same roles. If you provide secondary authorization headers, those credentials are used instead. You must use Kibana, this API, or the create anomaly detection jobs API to create a datafeed. Do not add a datafeed directly to the
.ml-configindex. Do not give users
writeprivileges on the
.ml-config`
* index.
*
* @see Documentation
* on elastic.co
*/
public PutDatafeedResponse putDatafeed(PutDatafeedRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a datafeed. Datafeeds retrieve data from Elasticsearch for analysis by
* an anomaly detection job. You can associate only one datafeed with each
* anomaly detection job. The datafeed contains a query that runs at a defined
* interval (frequency
). If you are concerned about delayed data,
* you can add a delay
* (query_delay') at each interval. When Elasticsearch security features are enabled, your datafeed remembers which roles the user who created it had at the time of creation and runs the query using those same roles. If you provide secondary authorization headers, those credentials are used instead. You must use Kibana, this API, or the create anomaly detection jobs API to create a datafeed. Do not add a datafeed directly to the
.ml-configindex. Do not give users
writeprivileges on the
.ml-config`
* index.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final PutDatafeedResponse putDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return putDatafeed(fn.apply(new PutDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.put_filter
/**
* Create a filter. A filter contains a list of strings. It can be used by one
* or more anomaly detection jobs. Specifically, filters are referenced in the
* custom_rules
property of detector configuration objects.
*
* @see Documentation
* on elastic.co
*/
public PutFilterResponse putFilter(PutFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a filter. A filter contains a list of strings. It can be used by one
* or more anomaly detection jobs. Specifically, filters are referenced in the
* custom_rules
property of detector configuration objects.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutFilterRequest}
* @see Documentation
* on elastic.co
*/
public final PutFilterResponse putFilter(Function> fn)
throws IOException, ElasticsearchException {
return putFilter(fn.apply(new PutFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.put_job
/**
* Create an anomaly detection job. If you include a
* datafeed_config
, you must have read index privileges on the
* source index.
*
* @see Documentation
* on elastic.co
*/
public PutJobResponse putJob(PutJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create an anomaly detection job. If you include a
* datafeed_config
, you must have read index privileges on the
* source index.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutJobRequest}
* @see Documentation
* on elastic.co
*/
public final PutJobResponse putJob(Function> fn)
throws IOException, ElasticsearchException {
return putJob(fn.apply(new PutJobRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model
/**
* Create a trained model. Enable you to supply a trained model that is not
* created by data frame analytics.
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelResponse putTrainedModel(PutTrainedModelRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a trained model. Enable you to supply a trained model that is not
* created by data frame analytics.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelResponse putTrainedModel(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModel(fn.apply(new PutTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_alias
/**
* Create or update a trained model alias. A trained model alias is a logical
* name used to reference a single trained model. You can use aliases instead of
* trained model identifiers to make it easier to reference your models. For
* example, you can use aliases in inference aggregations and processors. An
* alias must be unique and refer to only a single trained model. However, you
* can have multiple aliases for each trained model. If you use this API to
* update an alias such that it references a different trained model ID and the
* model uses a different type of data frame analytics, an error occurs. For
* example, this situation occurs if you have a trained model for regression
* analysis and a trained model for classification analysis; you cannot reassign
* an alias from one type of trained model to another. If you use this API to
* update an alias and there are very few input fields in common between the old
* and new trained models for the model alias, the API returns a warning.
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelAliasResponse putTrainedModelAlias(PutTrainedModelAliasRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelAliasRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create or update a trained model alias. A trained model alias is a logical
* name used to reference a single trained model. You can use aliases instead of
* trained model identifiers to make it easier to reference your models. For
* example, you can use aliases in inference aggregations and processors. An
* alias must be unique and refer to only a single trained model. However, you
* can have multiple aliases for each trained model. If you use this API to
* update an alias such that it references a different trained model ID and the
* model uses a different type of data frame analytics, an error occurs. For
* example, this situation occurs if you have a trained model for regression
* analysis and a trained model for classification analysis; you cannot reassign
* an alias from one type of trained model to another. If you use this API to
* update an alias and there are very few input fields in common between the old
* and new trained models for the model alias, the API returns a warning.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelAliasRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelAliasResponse putTrainedModelAlias(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelAlias(fn.apply(new PutTrainedModelAliasRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_definition_part
/**
* Create part of a trained model definition.
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelDefinitionPartResponse putTrainedModelDefinitionPart(
PutTrainedModelDefinitionPartRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelDefinitionPartRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create part of a trained model definition.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelDefinitionPartRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelDefinitionPartResponse putTrainedModelDefinitionPart(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelDefinitionPart(fn.apply(new PutTrainedModelDefinitionPartRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_vocabulary
/**
* Create a trained model vocabulary. This API is supported only for natural
* language processing (NLP) models. The vocabulary is stored in the index as
* described in inference_config.*.vocabulary
of the trained model
* definition.
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelVocabularyResponse putTrainedModelVocabulary(PutTrainedModelVocabularyRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelVocabularyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a trained model vocabulary. This API is supported only for natural
* language processing (NLP) models. The vocabulary is stored in the index as
* described in inference_config.*.vocabulary
of the trained model
* definition.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelVocabularyRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelVocabularyResponse putTrainedModelVocabulary(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelVocabulary(fn.apply(new PutTrainedModelVocabularyRequest.Builder()).build());
}
// ----- Endpoint: ml.reset_job
/**
* Reset an anomaly detection job. All model state and results are deleted. The
* job is ready to start over as if it had just been created. It is not
* currently possible to reset multiple jobs using wildcards or a comma
* separated list.
*
* @see Documentation
* on elastic.co
*/
public ResetJobResponse resetJob(ResetJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResetJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Reset an anomaly detection job. All model state and results are deleted. The
* job is ready to start over as if it had just been created. It is not
* currently possible to reset multiple jobs using wildcards or a comma
* separated list.
*
* @param fn
* a function that initializes a builder to create the
* {@link ResetJobRequest}
* @see Documentation
* on elastic.co
*/
public final ResetJobResponse resetJob(Function> fn)
throws IOException, ElasticsearchException {
return resetJob(fn.apply(new ResetJobRequest.Builder()).build());
}
// ----- Endpoint: ml.revert_model_snapshot
/**
* Revert to a snapshot. The machine learning features react quickly to
* anomalous input, learning new behaviors in data. Highly anomalous input
* increases the variance in the models whilst the system learns whether this is
* a new step-change in behavior or a one-off event. In the case where this
* anomalous input is known to be a one-off, then it might be appropriate to
* reset the model state to a time before this event. For example, you might
* consider reverting to a saved snapshot after Black Friday or a critical
* system failure.
*
* @see Documentation
* on elastic.co
*/
public RevertModelSnapshotResponse revertModelSnapshot(RevertModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RevertModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Revert to a snapshot. The machine learning features react quickly to
* anomalous input, learning new behaviors in data. Highly anomalous input
* increases the variance in the models whilst the system learns whether this is
* a new step-change in behavior or a one-off event. In the case where this
* anomalous input is known to be a one-off, then it might be appropriate to
* reset the model state to a time before this event. For example, you might
* consider reverting to a saved snapshot after Black Friday or a critical
* system failure.
*
* @param fn
* a function that initializes a builder to create the
* {@link RevertModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final RevertModelSnapshotResponse revertModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return revertModelSnapshot(fn.apply(new RevertModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.set_upgrade_mode
/**
* Set upgrade_mode for ML indices. Sets a cluster wide upgrade_mode setting
* that prepares machine learning indices for an upgrade. When upgrading your
* cluster, in some circumstances you must restart your nodes and reindex your
* machine learning indices. In those circumstances, there must be no machine
* learning jobs running. You can close the machine learning jobs, do the
* upgrade, then open all the jobs again. Alternatively, you can use this API to
* temporarily halt tasks associated with the jobs and datafeeds and prevent new
* jobs from opening. You can also use this API during upgrades that do not
* require you to reindex your machine learning indices, though stopping jobs is
* not a requirement in that case. You can see the current value for the
* upgrade_mode setting by using the get machine learning info API.
*
* @see Documentation
* on elastic.co
*/
public SetUpgradeModeResponse setUpgradeMode(SetUpgradeModeRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SetUpgradeModeRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Set upgrade_mode for ML indices. Sets a cluster wide upgrade_mode setting
* that prepares machine learning indices for an upgrade. When upgrading your
* cluster, in some circumstances you must restart your nodes and reindex your
* machine learning indices. In those circumstances, there must be no machine
* learning jobs running. You can close the machine learning jobs, do the
* upgrade, then open all the jobs again. Alternatively, you can use this API to
* temporarily halt tasks associated with the jobs and datafeeds and prevent new
* jobs from opening. You can also use this API during upgrades that do not
* require you to reindex your machine learning indices, though stopping jobs is
* not a requirement in that case. You can see the current value for the
* upgrade_mode setting by using the get machine learning info API.
*
* @param fn
* a function that initializes a builder to create the
* {@link SetUpgradeModeRequest}
* @see Documentation
* on elastic.co
*/
public final SetUpgradeModeResponse setUpgradeMode(
Function> fn)
throws IOException, ElasticsearchException {
return setUpgradeMode(fn.apply(new SetUpgradeModeRequest.Builder()).build());
}
/**
* Set upgrade_mode for ML indices. Sets a cluster wide upgrade_mode setting
* that prepares machine learning indices for an upgrade. When upgrading your
* cluster, in some circumstances you must restart your nodes and reindex your
* machine learning indices. In those circumstances, there must be no machine
* learning jobs running. You can close the machine learning jobs, do the
* upgrade, then open all the jobs again. Alternatively, you can use this API to
* temporarily halt tasks associated with the jobs and datafeeds and prevent new
* jobs from opening. You can also use this API during upgrades that do not
* require you to reindex your machine learning indices, though stopping jobs is
* not a requirement in that case. You can see the current value for the
* upgrade_mode setting by using the get machine learning info API.
*
* @see Documentation
* on elastic.co
*/
public SetUpgradeModeResponse setUpgradeMode() throws IOException, ElasticsearchException {
return this.transport.performRequest(new SetUpgradeModeRequest.Builder().build(),
SetUpgradeModeRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.start_data_frame_analytics
/**
* Start a data frame analytics job. A data frame analytics job can be started
* and stopped multiple times throughout its lifecycle. If the destination index
* does not exist, it is created automatically the first time you start the data
* frame analytics job. The index.number_of_shards
and
* index.number_of_replicas
settings for the destination index are
* copied from the source index. If there are multiple source indices, the
* destination index copies the highest setting values. The mappings for the
* destination index are also copied from the source indices. If there are any
* mapping conflicts, the job fails to start. If the destination index exists,
* it is used as is. You can therefore set up the destination index in advance
* with custom settings and mappings.
*
* @see Documentation
* on elastic.co
*/
public StartDataFrameAnalyticsResponse startDataFrameAnalytics(StartDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Start a data frame analytics job. A data frame analytics job can be started
* and stopped multiple times throughout its lifecycle. If the destination index
* does not exist, it is created automatically the first time you start the data
* frame analytics job. The index.number_of_shards
and
* index.number_of_replicas
settings for the destination index are
* copied from the source index. If there are multiple source indices, the
* destination index copies the highest setting values. The mappings for the
* destination index are also copied from the source indices. If there are any
* mapping conflicts, the job fails to start. If the destination index exists,
* it is used as is. You can therefore set up the destination index in advance
* with custom settings and mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final StartDataFrameAnalyticsResponse startDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return startDataFrameAnalytics(fn.apply(new StartDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.start_datafeed
/**
* Start datafeeds.
*
* A datafeed must be started in order to retrieve data from Elasticsearch. A
* datafeed can be started and stopped multiple times throughout its lifecycle.
*
* Before you can start a datafeed, the anomaly detection job must be open.
* Otherwise, an error occurs.
*
* If you restart a stopped datafeed, it continues processing input data from
* the next millisecond after it was stopped. If new data was indexed for that
* exact millisecond between stopping and starting, it will be ignored.
*
* When Elasticsearch security features are enabled, your datafeed remembers
* which roles the last user to create or update it had at the time of creation
* or update and runs the query using those same roles. If you provided
* secondary authorization headers when you created or updated the datafeed,
* those credentials are used instead.
*
* @see Documentation
* on elastic.co
*/
public StartDatafeedResponse startDatafeed(StartDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Start datafeeds.
*
* A datafeed must be started in order to retrieve data from Elasticsearch. A
* datafeed can be started and stopped multiple times throughout its lifecycle.
*
* Before you can start a datafeed, the anomaly detection job must be open.
* Otherwise, an error occurs.
*
* If you restart a stopped datafeed, it continues processing input data from
* the next millisecond after it was stopped. If new data was indexed for that
* exact millisecond between stopping and starting, it will be ignored.
*
* When Elasticsearch security features are enabled, your datafeed remembers
* which roles the last user to create or update it had at the time of creation
* or update and runs the query using those same roles. If you provided
* secondary authorization headers when you created or updated the datafeed,
* those credentials are used instead.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final StartDatafeedResponse startDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return startDatafeed(fn.apply(new StartDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.start_trained_model_deployment
/**
* Start a trained model deployment. It allocates the model to every machine
* learning node.
*
* @see Documentation
* on elastic.co
*/
public StartTrainedModelDeploymentResponse startTrainedModelDeployment(StartTrainedModelDeploymentRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Start a trained model deployment. It allocates the model to every machine
* learning node.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final StartTrainedModelDeploymentResponse startTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return startTrainedModelDeployment(fn.apply(new StartTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_data_frame_analytics
/**
* Stop data frame analytics jobs. A data frame analytics job can be started and
* stopped multiple times throughout its lifecycle.
*
* @see Documentation
* on elastic.co
*/
public StopDataFrameAnalyticsResponse stopDataFrameAnalytics(StopDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stop data frame analytics jobs. A data frame analytics job can be started and
* stopped multiple times throughout its lifecycle.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final StopDataFrameAnalyticsResponse stopDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return stopDataFrameAnalytics(fn.apply(new StopDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_datafeed
/**
* Stop datafeeds. A datafeed that is stopped ceases to retrieve data from
* Elasticsearch. A datafeed can be started and stopped multiple times
* throughout its lifecycle.
*
* @see Documentation
* on elastic.co
*/
public StopDatafeedResponse stopDatafeed(StopDatafeedRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stop datafeeds. A datafeed that is stopped ceases to retrieve data from
* Elasticsearch. A datafeed can be started and stopped multiple times
* throughout its lifecycle.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final StopDatafeedResponse stopDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return stopDatafeed(fn.apply(new StopDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_trained_model_deployment
/**
* Stop a trained model deployment.
*
* @see Documentation
* on elastic.co
*/
public StopTrainedModelDeploymentResponse stopTrainedModelDeployment(StopTrainedModelDeploymentRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stop a trained model deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final StopTrainedModelDeploymentResponse stopTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return stopTrainedModelDeployment(fn.apply(new StopTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.update_data_frame_analytics
/**
* Update a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public UpdateDataFrameAnalyticsResponse updateDataFrameAnalytics(UpdateDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateDataFrameAnalyticsResponse updateDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return updateDataFrameAnalytics(fn.apply(new UpdateDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.update_datafeed
/**
* Update a datafeed. You must stop and start the datafeed for the changes to be
* applied. When Elasticsearch security features are enabled, your datafeed
* remembers which roles the user who updated it had at the time of the update
* and runs the query using those same roles. If you provide secondary
* authorization headers, those credentials are used instead.
*
* @see Documentation
* on elastic.co
*/
public UpdateDatafeedResponse updateDatafeed(UpdateDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a datafeed. You must stop and start the datafeed for the changes to be
* applied. When Elasticsearch security features are enabled, your datafeed
* remembers which roles the user who updated it had at the time of the update
* and runs the query using those same roles. If you provide secondary
* authorization headers, those credentials are used instead.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateDatafeedResponse updateDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return updateDatafeed(fn.apply(new UpdateDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.update_filter
/**
* Update a filter. Updates the description of a filter, adds items, or removes
* items from the list.
*
* @see Documentation
* on elastic.co
*/
public UpdateFilterResponse updateFilter(UpdateFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a filter. Updates the description of a filter, adds items, or removes
* items from the list.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateFilterRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateFilterResponse updateFilter(
Function> fn)
throws IOException, ElasticsearchException {
return updateFilter(fn.apply(new UpdateFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.update_job
/**
* Update an anomaly detection job. Updates certain properties of an anomaly
* detection job.
*
* @see Documentation
* on elastic.co
*/
public UpdateJobResponse updateJob(UpdateJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update an anomaly detection job. Updates certain properties of an anomaly
* detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateJobRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateJobResponse updateJob(Function> fn)
throws IOException, ElasticsearchException {
return updateJob(fn.apply(new UpdateJobRequest.Builder()).build());
}
// ----- Endpoint: ml.update_model_snapshot
/**
* Update a snapshot. Updates certain properties of a snapshot.
*
* @see Documentation
* on elastic.co
*/
public UpdateModelSnapshotResponse updateModelSnapshot(UpdateModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a snapshot. Updates certain properties of a snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateModelSnapshotResponse updateModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return updateModelSnapshot(fn.apply(new UpdateModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.update_trained_model_deployment
/**
* Update a trained model deployment.
*
* @see Documentation
* on elastic.co
*/
public UpdateTrainedModelDeploymentResponse updateTrainedModelDeployment(
UpdateTrainedModelDeploymentRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a trained model deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateTrainedModelDeploymentResponse updateTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return updateTrainedModelDeployment(fn.apply(new UpdateTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.upgrade_job_snapshot
/**
* Upgrade a snapshot. Upgrades an anomaly detection model snapshot to the
* latest major version. Over time, older snapshot formats are deprecated and
* removed. Anomaly detection jobs support only snapshots that are from the
* current or previous major version. This API provides a means to upgrade a
* snapshot to the current major version. This aids in preparing the cluster for
* an upgrade to the next major version. Only one snapshot per anomaly detection
* job can be upgraded at a time and the upgraded snapshot cannot be the current
* snapshot of the anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public UpgradeJobSnapshotResponse upgradeJobSnapshot(UpgradeJobSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpgradeJobSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Upgrade a snapshot. Upgrades an anomaly detection model snapshot to the
* latest major version. Over time, older snapshot formats are deprecated and
* removed. Anomaly detection jobs support only snapshots that are from the
* current or previous major version. This API provides a means to upgrade a
* snapshot to the current major version. This aids in preparing the cluster for
* an upgrade to the next major version. Only one snapshot per anomaly detection
* job can be upgraded at a time and the upgraded snapshot cannot be the current
* snapshot of the anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpgradeJobSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final UpgradeJobSnapshotResponse upgradeJobSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return upgradeJobSnapshot(fn.apply(new UpgradeJobSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.validate
/**
* Validates an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public ValidateResponse validate(ValidateRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ValidateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Validates an anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link ValidateRequest}
* @see Documentation
* on elastic.co
*/
public final ValidateResponse validate(Function> fn)
throws IOException, ElasticsearchException {
return validate(fn.apply(new ValidateRequest.Builder()).build());
}
/**
* Validates an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public ValidateResponse validate() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ValidateRequest.Builder().build(), ValidateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.validate_detector
/**
* Validates an anomaly detection detector.
*
* @see Documentation
* on elastic.co
*/
public ValidateDetectorResponse validateDetector(ValidateDetectorRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ValidateDetectorRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Validates an anomaly detection detector.
*
* @param fn
* a function that initializes a builder to create the
* {@link ValidateDetectorRequest}
* @see Documentation
* on elastic.co
*/
public final ValidateDetectorResponse validateDetector(
Function> fn)
throws IOException, ElasticsearchException {
return validateDetector(fn.apply(new ValidateDetectorRequest.Builder()).build());
}
/**
* Validates an anomaly detection detector.
*
* @see Documentation
* on elastic.co
*/
public ValidateDetectorResponse validateDetector() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ValidateDetectorRequest.Builder().build(),
ValidateDetectorRequest._ENDPOINT, this.transportOptions);
}
}