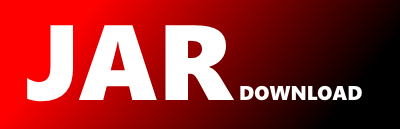
co.elastic.clients.elasticsearch.security.ElasticsearchSecurityAsyncClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.security;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the security namespace.
*/
public class ElasticsearchSecurityAsyncClient
extends
ApiClient {
public ElasticsearchSecurityAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchSecurityAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchSecurityAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchSecurityAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: security.activate_user_profile
/**
* Activate a user profile.
*
* Create or update a user profile on behalf of another user.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture activateUserProfile(ActivateUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ActivateUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Activate a user profile.
*
* Create or update a user profile on behalf of another user.
*
* @param fn
* a function that initializes a builder to create the
* {@link ActivateUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture activateUserProfile(
Function> fn) {
return activateUserProfile(fn.apply(new ActivateUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.authenticate
/**
* Authenticate a user.
*
* Authenticates a user and returns information about the authenticated user.
* Include the user information in a
* basic
* auth header. A successful call returns a JSON structure that shows user
* information such as their username, the roles that are assigned to the user,
* any assigned metadata, and information about the realms that authenticated
* and authorized the user. If the user cannot be authenticated, this API
* returns a 401 status code.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture authenticate() {
return this.transport.performRequestAsync(AuthenticateRequest._INSTANCE, AuthenticateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.bulk_delete_role
/**
* Bulk delete roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture bulkDeleteRole(BulkDeleteRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkDeleteRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Bulk delete roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkDeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture bulkDeleteRole(
Function> fn) {
return bulkDeleteRole(fn.apply(new BulkDeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.bulk_put_role
/**
* Bulk create or update roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture bulkPutRole(BulkPutRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkPutRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Bulk create or update roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkPutRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture bulkPutRole(
Function> fn) {
return bulkPutRole(fn.apply(new BulkPutRoleRequest.Builder()).build());
}
// ----- Endpoint: security.change_password
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture changePassword(ChangePasswordRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ChangePasswordRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ChangePasswordRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture changePassword(
Function> fn) {
return changePassword(fn.apply(new ChangePasswordRequest.Builder()).build());
}
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture changePassword() {
return this.transport.performRequestAsync(new ChangePasswordRequest.Builder().build(),
ChangePasswordRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.clear_api_key_cache
/**
* Clear the API key cache.
*
* Evict a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearApiKeyCache(ClearApiKeyCacheRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearApiKeyCacheRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear the API key cache.
*
* Evict a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearApiKeyCacheRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearApiKeyCache(
Function> fn) {
return clearApiKeyCache(fn.apply(new ClearApiKeyCacheRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_privileges
/**
* Clear the privileges cache.
*
* Evict privileges from the native application privilege cache. The cache is
* also automatically cleared for applications that have their privileges
* updated.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedPrivileges(
ClearCachedPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear the privileges cache.
*
* Evict privileges from the native application privilege cache. The cache is
* also automatically cleared for applications that have their privileges
* updated.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedPrivileges(
Function> fn) {
return clearCachedPrivileges(fn.apply(new ClearCachedPrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_realms
/**
* Clear the user cache.
*
* Evict users from the user cache. You can completely clear the cache or evict
* specific users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedRealms(ClearCachedRealmsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRealmsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear the user cache.
*
* Evict users from the user cache. You can completely clear the cache or evict
* specific users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRealmsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedRealms(
Function> fn) {
return clearCachedRealms(fn.apply(new ClearCachedRealmsRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_roles
/**
* Clear the roles cache.
*
* Evict roles from the native role cache.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedRoles(ClearCachedRolesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRolesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear the roles cache.
*
* Evict roles from the native role cache.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRolesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedRoles(
Function> fn) {
return clearCachedRoles(fn.apply(new ClearCachedRolesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_service_tokens
/**
* Clear service account token caches.
*
* Evict a subset of all entries from the service account token caches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture clearCachedServiceTokens(
ClearCachedServiceTokensRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedServiceTokensRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Clear service account token caches.
*
* Evict a subset of all entries from the service account token caches.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedServiceTokensRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture clearCachedServiceTokens(
Function> fn) {
return clearCachedServiceTokens(fn.apply(new ClearCachedServiceTokensRequest.Builder()).build());
}
// ----- Endpoint: security.create_api_key
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createApiKey(CreateApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createApiKey(
Function> fn) {
return createApiKey(fn.apply(new CreateApiKeyRequest.Builder()).build());
}
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createApiKey() {
return this.transport.performRequestAsync(new CreateApiKeyRequest.Builder().build(),
CreateApiKeyRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.create_cross_cluster_api_key
/**
* Create a cross-cluster API key.
*
* Create an API key of the cross_cluster
type for the API key
* based remote cluster access. A cross_cluster
API key cannot be
* used to authenticate through the REST interface.
*
* IMPORTANT: To authenticate this request you must use a credential that is not
* an API key. Even if you use an API key that has the required privilege, the
* API returns an error.
*
* Cross-cluster API keys are created by the Elasticsearch API key service,
* which is automatically enabled.
*
* NOTE: Unlike REST API keys, a cross-cluster API key does not capture
* permissions of the authenticated user. The API key’s effective permission is
* exactly as specified with the access
property.
*
* A successful request returns a JSON structure that contains the API key, its
* unique ID, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* Cross-cluster API keys can only be updated with the update cross-cluster API
* key API. Attempting to update them with the update REST API key API or the
* bulk update REST API keys API will result in an error.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createCrossClusterApiKey(
CreateCrossClusterApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateCrossClusterApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create a cross-cluster API key.
*
* Create an API key of the cross_cluster
type for the API key
* based remote cluster access. A cross_cluster
API key cannot be
* used to authenticate through the REST interface.
*
* IMPORTANT: To authenticate this request you must use a credential that is not
* an API key. Even if you use an API key that has the required privilege, the
* API returns an error.
*
* Cross-cluster API keys are created by the Elasticsearch API key service,
* which is automatically enabled.
*
* NOTE: Unlike REST API keys, a cross-cluster API key does not capture
* permissions of the authenticated user. The API key’s effective permission is
* exactly as specified with the access
property.
*
* A successful request returns a JSON structure that contains the API key, its
* unique ID, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* Cross-cluster API keys can only be updated with the update cross-cluster API
* key API. Attempting to update them with the update REST API key API or the
* bulk update REST API keys API will result in an error.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateCrossClusterApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createCrossClusterApiKey(
Function> fn) {
return createCrossClusterApiKey(fn.apply(new CreateCrossClusterApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.create_service_token
/**
* Create a service account token.
*
* Create a service accounts token for access without requiring basic
* authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture createServiceToken(CreateServiceTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateServiceTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Create a service account token.
*
* Create a service accounts token for access without requiring basic
* authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture createServiceToken(
Function> fn) {
return createServiceToken(fn.apply(new CreateServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_privileges
/**
* Delete application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deletePrivileges(DeletePrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeletePrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeletePrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deletePrivileges(
Function> fn) {
return deletePrivileges(fn.apply(new DeletePrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role
/**
* Delete roles.
*
* Delete roles in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteRole(DeleteRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete roles.
*
* Delete roles in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteRole(
Function> fn) {
return deleteRole(fn.apply(new DeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role_mapping
/**
* Delete role mappings.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteRoleMapping(DeleteRoleMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete role mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteRoleMapping(
Function> fn) {
return deleteRoleMapping(fn.apply(new DeleteRoleMappingRequest.Builder()).build());
}
// ----- Endpoint: security.delete_service_token
/**
* Delete service account tokens.
*
* Delete service account tokens for a service in a specified namespace.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteServiceToken(DeleteServiceTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteServiceTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete service account tokens.
*
* Delete service account tokens for a service in a specified namespace.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteServiceToken(
Function> fn) {
return deleteServiceToken(fn.apply(new DeleteServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_user
/**
* Delete users.
*
* Delete users from the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteUser(DeleteUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Delete users.
*
* Delete users from the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteUser(
Function> fn) {
return deleteUser(fn.apply(new DeleteUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user
/**
* Disable users.
*
* Disable users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture disableUser(DisableUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Disable users.
*
* Disable users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture disableUser(
Function> fn) {
return disableUser(fn.apply(new DisableUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user_profile
/**
* Disable a user profile.
*
* Disable user profiles so that they are not visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture disableUserProfile(DisableUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Disable a user profile.
*
* Disable user profiles so that they are not visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture disableUserProfile(
Function> fn) {
return disableUserProfile(fn.apply(new DisableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user
/**
* Enable users.
*
* Enable users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enableUser(EnableUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Enable users.
*
* Enable users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture enableUser(
Function> fn) {
return enableUser(fn.apply(new EnableUserRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user_profile
/**
* Enable a user profile.
*
* Enable user profiles to make them visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enableUserProfile(EnableUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Enable a user profile.
*
* Enable user profiles to make them visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture enableUserProfile(
Function> fn) {
return enableUserProfile(fn.apply(new EnableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enroll_kibana
/**
* Enroll Kibana.
*
* Enable a Kibana instance to configure itself for communication with a secured
* Elasticsearch cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enrollKibana() {
return this.transport.performRequestAsync(EnrollKibanaRequest._INSTANCE, EnrollKibanaRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.enroll_node
/**
* Enroll a node.
*
* Enroll a new node to allow it to join an existing cluster with security
* features enabled.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture enrollNode() {
return this.transport.performRequestAsync(EnrollNodeRequest._INSTANCE, EnrollNodeRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_api_key
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getApiKey(GetApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getApiKey(
Function> fn) {
return getApiKey(fn.apply(new GetApiKeyRequest.Builder()).build());
}
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getApiKey() {
return this.transport.performRequestAsync(new GetApiKeyRequest.Builder().build(), GetApiKeyRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_builtin_privileges
/**
* Get builtin privileges.
*
* Get the list of cluster privileges and index privileges that are available in
* this version of Elasticsearch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getBuiltinPrivileges() {
return this.transport.performRequestAsync(GetBuiltinPrivilegesRequest._INSTANCE,
GetBuiltinPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_privileges
/**
* Get application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getPrivileges(GetPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getPrivileges(
Function> fn) {
return getPrivileges(fn.apply(new GetPrivilegesRequest.Builder()).build());
}
/**
* Get application privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getPrivileges() {
return this.transport.performRequestAsync(new GetPrivilegesRequest.Builder().build(),
GetPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_role
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRole(GetRoleRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getRole(
Function> fn) {
return getRole(fn.apply(new GetRoleRequest.Builder()).build());
}
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRole() {
return this.transport.performRequestAsync(new GetRoleRequest.Builder().build(), GetRoleRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_role_mapping
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRoleMapping(GetRoleMappingRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleMappingRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getRoleMapping(
Function> fn) {
return getRoleMapping(fn.apply(new GetRoleMappingRequest.Builder()).build());
}
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getRoleMapping() {
return this.transport.performRequestAsync(new GetRoleMappingRequest.Builder().build(),
GetRoleMappingRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_accounts
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceAccounts(GetServiceAccountsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceAccountsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceAccountsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getServiceAccounts(
Function> fn) {
return getServiceAccounts(fn.apply(new GetServiceAccountsRequest.Builder()).build());
}
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceAccounts() {
return this.transport.performRequestAsync(new GetServiceAccountsRequest.Builder().build(),
GetServiceAccountsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_credentials
/**
* Get service account credentials.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getServiceCredentials(
GetServiceCredentialsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceCredentialsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get service account credentials.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceCredentialsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getServiceCredentials(
Function> fn) {
return getServiceCredentials(fn.apply(new GetServiceCredentialsRequest.Builder()).build());
}
// ----- Endpoint: security.get_token
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getToken(GetTokenRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTokenRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getToken(
Function> fn) {
return getToken(fn.apply(new GetTokenRequest.Builder()).build());
}
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getToken() {
return this.transport.performRequestAsync(new GetTokenRequest.Builder().build(), GetTokenRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUser(GetUserRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUser(
Function> fn) {
return getUser(fn.apply(new GetUserRequest.Builder()).build());
}
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUser() {
return this.transport.performRequestAsync(new GetUserRequest.Builder().build(), GetUserRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user_privileges
/**
* Get user privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserPrivileges(GetUserPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get user privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUserPrivileges(
Function> fn) {
return getUserPrivileges(fn.apply(new GetUserPrivilegesRequest.Builder()).build());
}
/**
* Get user privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserPrivileges() {
return this.transport.performRequestAsync(new GetUserPrivilegesRequest.Builder().build(),
GetUserPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_user_profile
/**
* Get a user profile.
*
* Get a user's profile using the unique profile ID.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getUserProfile(GetUserProfileRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserProfileRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Get a user profile.
*
* Get a user's profile using the unique profile ID.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getUserProfile(
Function> fn) {
return getUserProfile(fn.apply(new GetUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.grant_api_key
/**
* Grant an API key.
*
* Create an API key on behalf of another user. This API is similar to the
* create API keys API, however it creates the API key for a user that is
* different than the user that runs the API. The caller must have
* authentication credentials (either an access token, or a username and
* password) for the user on whose behalf the API key will be created. It is not
* possible to use this API to create an API key without that user’s
* credentials. The user, for whom the authentication credentials is provided,
* can optionally "run as" (impersonate) another user. In this case,
* the API key will be created on behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture grantApiKey(GrantApiKeyRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GrantApiKeyRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Grant an API key.
*
* Create an API key on behalf of another user. This API is similar to the
* create API keys API, however it creates the API key for a user that is
* different than the user that runs the API. The caller must have
* authentication credentials (either an access token, or a username and
* password) for the user on whose behalf the API key will be created. It is not
* possible to use this API to create an API key without that user’s
* credentials. The user, for whom the authentication credentials is provided,
* can optionally "run as" (impersonate) another user. In this case,
* the API key will be created on behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link GrantApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture grantApiKey(
Function> fn) {
return grantApiKey(fn.apply(new GrantApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.has_privileges
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture hasPrivileges(HasPrivilegesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HasPrivilegesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link HasPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture hasPrivileges(
Function> fn) {
return hasPrivileges(fn.apply(new HasPrivilegesRequest.Builder()).build());
}
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture hasPrivileges() {
return this.transport.performRequestAsync(new HasPrivilegesRequest.Builder().build(),
HasPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.has_privileges_user_profile
/**
* Check user profile privileges.
*