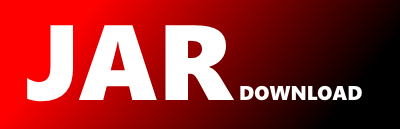
co.elastic.clients.elasticsearch.security.ElasticsearchSecurityClient Maven / Gradle / Ivy
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.security;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
/**
* Client for the security namespace.
*/
public class ElasticsearchSecurityClient extends ApiClient {
public ElasticsearchSecurityClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchSecurityClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchSecurityClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchSecurityClient(this.transport, transportOptions);
}
// ----- Endpoint: security.activate_user_profile
/**
* Activate a user profile.
*
* Create or update a user profile on behalf of another user.
*
* @see Documentation
* on elastic.co
*/
public ActivateUserProfileResponse activateUserProfile(ActivateUserProfileRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ActivateUserProfileRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Activate a user profile.
*
* Create or update a user profile on behalf of another user.
*
* @param fn
* a function that initializes a builder to create the
* {@link ActivateUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final ActivateUserProfileResponse activateUserProfile(
Function> fn)
throws IOException, ElasticsearchException {
return activateUserProfile(fn.apply(new ActivateUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.authenticate
/**
* Authenticate a user.
*
* Authenticates a user and returns information about the authenticated user.
* Include the user information in a
* basic
* auth header. A successful call returns a JSON structure that shows user
* information such as their username, the roles that are assigned to the user,
* any assigned metadata, and information about the realms that authenticated
* and authorized the user. If the user cannot be authenticated, this API
* returns a 401 status code.
*
* @see Documentation
* on elastic.co
*/
public AuthenticateResponse authenticate() throws IOException, ElasticsearchException {
return this.transport.performRequest(AuthenticateRequest._INSTANCE, AuthenticateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.bulk_delete_role
/**
* Bulk delete roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public BulkDeleteRoleResponse bulkDeleteRole(BulkDeleteRoleRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkDeleteRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Bulk delete roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk delete roles API
* cannot delete roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkDeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final BulkDeleteRoleResponse bulkDeleteRole(
Function> fn)
throws IOException, ElasticsearchException {
return bulkDeleteRole(fn.apply(new BulkDeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.bulk_put_role
/**
* Bulk create or update roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public BulkPutRoleResponse bulkPutRole(BulkPutRoleRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) BulkPutRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Bulk create or update roles.
*
* The role management APIs are generally the preferred way to manage roles,
* rather than using file-based role management. The bulk create or update roles
* API cannot update roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link BulkPutRoleRequest}
* @see Documentation
* on elastic.co
*/
public final BulkPutRoleResponse bulkPutRole(
Function> fn)
throws IOException, ElasticsearchException {
return bulkPutRole(fn.apply(new BulkPutRoleRequest.Builder()).build());
}
// ----- Endpoint: security.change_password
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public ChangePasswordResponse changePassword(ChangePasswordRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ChangePasswordRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ChangePasswordRequest}
* @see Documentation
* on elastic.co
*/
public final ChangePasswordResponse changePassword(
Function> fn)
throws IOException, ElasticsearchException {
return changePassword(fn.apply(new ChangePasswordRequest.Builder()).build());
}
/**
* Change passwords.
*
* Change the passwords of users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public ChangePasswordResponse changePassword() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ChangePasswordRequest.Builder().build(),
ChangePasswordRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.clear_api_key_cache
/**
* Clear the API key cache.
*
* Evict a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @see Documentation
* on elastic.co
*/
public ClearApiKeyCacheResponse clearApiKeyCache(ClearApiKeyCacheRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearApiKeyCacheRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear the API key cache.
*
* Evict a subset of all entries from the API key cache. The cache is also
* automatically cleared on state changes of the security index.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearApiKeyCacheRequest}
* @see Documentation
* on elastic.co
*/
public final ClearApiKeyCacheResponse clearApiKeyCache(
Function> fn)
throws IOException, ElasticsearchException {
return clearApiKeyCache(fn.apply(new ClearApiKeyCacheRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_privileges
/**
* Clear the privileges cache.
*
* Evict privileges from the native application privilege cache. The cache is
* also automatically cleared for applications that have their privileges
* updated.
*
* @see Documentation
* on elastic.co
*/
public ClearCachedPrivilegesResponse clearCachedPrivileges(ClearCachedPrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedPrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear the privileges cache.
*
* Evict privileges from the native application privilege cache. The cache is
* also automatically cleared for applications that have their privileges
* updated.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final ClearCachedPrivilegesResponse clearCachedPrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return clearCachedPrivileges(fn.apply(new ClearCachedPrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_realms
/**
* Clear the user cache.
*
* Evict users from the user cache. You can completely clear the cache or evict
* specific users.
*
* @see Documentation
* on elastic.co
*/
public ClearCachedRealmsResponse clearCachedRealms(ClearCachedRealmsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRealmsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear the user cache.
*
* Evict users from the user cache. You can completely clear the cache or evict
* specific users.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRealmsRequest}
* @see Documentation
* on elastic.co
*/
public final ClearCachedRealmsResponse clearCachedRealms(
Function> fn)
throws IOException, ElasticsearchException {
return clearCachedRealms(fn.apply(new ClearCachedRealmsRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_roles
/**
* Clear the roles cache.
*
* Evict roles from the native role cache.
*
* @see Documentation
* on elastic.co
*/
public ClearCachedRolesResponse clearCachedRoles(ClearCachedRolesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedRolesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear the roles cache.
*
* Evict roles from the native role cache.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedRolesRequest}
* @see Documentation
* on elastic.co
*/
public final ClearCachedRolesResponse clearCachedRoles(
Function> fn)
throws IOException, ElasticsearchException {
return clearCachedRoles(fn.apply(new ClearCachedRolesRequest.Builder()).build());
}
// ----- Endpoint: security.clear_cached_service_tokens
/**
* Clear service account token caches.
*
* Evict a subset of all entries from the service account token caches.
*
* @see Documentation
* on elastic.co
*/
public ClearCachedServiceTokensResponse clearCachedServiceTokens(ClearCachedServiceTokensRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClearCachedServiceTokensRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clear service account token caches.
*
* Evict a subset of all entries from the service account token caches.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClearCachedServiceTokensRequest}
* @see Documentation
* on elastic.co
*/
public final ClearCachedServiceTokensResponse clearCachedServiceTokens(
Function> fn)
throws IOException, ElasticsearchException {
return clearCachedServiceTokens(fn.apply(new ClearCachedServiceTokensRequest.Builder()).build());
}
// ----- Endpoint: security.create_api_key
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CreateApiKeyResponse createApiKey(CreateApiKeyRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CreateApiKeyResponse createApiKey(
Function> fn)
throws IOException, ElasticsearchException {
return createApiKey(fn.apply(new CreateApiKeyRequest.Builder()).build());
}
/**
* Create an API key.
*
* Create an API key for access without requiring basic authentication. A
* successful request returns a JSON structure that contains the API key, its
* unique id, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds. NOTE: By default, API keys never
* expire. You can specify expiration information when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public CreateApiKeyResponse createApiKey() throws IOException, ElasticsearchException {
return this.transport.performRequest(new CreateApiKeyRequest.Builder().build(), CreateApiKeyRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.create_cross_cluster_api_key
/**
* Create a cross-cluster API key.
*
* Create an API key of the cross_cluster
type for the API key
* based remote cluster access. A cross_cluster
API key cannot be
* used to authenticate through the REST interface.
*
* IMPORTANT: To authenticate this request you must use a credential that is not
* an API key. Even if you use an API key that has the required privilege, the
* API returns an error.
*
* Cross-cluster API keys are created by the Elasticsearch API key service,
* which is automatically enabled.
*
* NOTE: Unlike REST API keys, a cross-cluster API key does not capture
* permissions of the authenticated user. The API key’s effective permission is
* exactly as specified with the access
property.
*
* A successful request returns a JSON structure that contains the API key, its
* unique ID, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* Cross-cluster API keys can only be updated with the update cross-cluster API
* key API. Attempting to update them with the update REST API key API or the
* bulk update REST API keys API will result in an error.
*
* @see Documentation
* on elastic.co
*/
public CreateCrossClusterApiKeyResponse createCrossClusterApiKey(CreateCrossClusterApiKeyRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateCrossClusterApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a cross-cluster API key.
*
* Create an API key of the cross_cluster
type for the API key
* based remote cluster access. A cross_cluster
API key cannot be
* used to authenticate through the REST interface.
*
* IMPORTANT: To authenticate this request you must use a credential that is not
* an API key. Even if you use an API key that has the required privilege, the
* API returns an error.
*
* Cross-cluster API keys are created by the Elasticsearch API key service,
* which is automatically enabled.
*
* NOTE: Unlike REST API keys, a cross-cluster API key does not capture
* permissions of the authenticated user. The API key’s effective permission is
* exactly as specified with the access
property.
*
* A successful request returns a JSON structure that contains the API key, its
* unique ID, and its name. If applicable, it also returns expiration
* information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* Cross-cluster API keys can only be updated with the update cross-cluster API
* key API. Attempting to update them with the update REST API key API or the
* bulk update REST API keys API will result in an error.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateCrossClusterApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final CreateCrossClusterApiKeyResponse createCrossClusterApiKey(
Function> fn)
throws IOException, ElasticsearchException {
return createCrossClusterApiKey(fn.apply(new CreateCrossClusterApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.create_service_token
/**
* Create a service account token.
*
* Create a service accounts token for access without requiring basic
* authentication.
*
* @see Documentation
* on elastic.co
*/
public CreateServiceTokenResponse createServiceToken(CreateServiceTokenRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateServiceTokenRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create a service account token.
*
* Create a service accounts token for access without requiring basic
* authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final CreateServiceTokenResponse createServiceToken(
Function> fn)
throws IOException, ElasticsearchException {
return createServiceToken(fn.apply(new CreateServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_privileges
/**
* Delete application privileges.
*
* @see Documentation
* on elastic.co
*/
public DeletePrivilegesResponse deletePrivileges(DeletePrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeletePrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeletePrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final DeletePrivilegesResponse deletePrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return deletePrivileges(fn.apply(new DeletePrivilegesRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role
/**
* Delete roles.
*
* Delete roles in the native realm.
*
* @see Documentation
* on elastic.co
*/
public DeleteRoleResponse deleteRole(DeleteRoleRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete roles.
*
* Delete roles in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteRoleResponse deleteRole(Function> fn)
throws IOException, ElasticsearchException {
return deleteRole(fn.apply(new DeleteRoleRequest.Builder()).build());
}
// ----- Endpoint: security.delete_role_mapping
/**
* Delete role mappings.
*
* @see Documentation
* on elastic.co
*/
public DeleteRoleMappingResponse deleteRoleMapping(DeleteRoleMappingRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRoleMappingRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete role mappings.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteRoleMappingResponse deleteRoleMapping(
Function> fn)
throws IOException, ElasticsearchException {
return deleteRoleMapping(fn.apply(new DeleteRoleMappingRequest.Builder()).build());
}
// ----- Endpoint: security.delete_service_token
/**
* Delete service account tokens.
*
* Delete service account tokens for a service in a specified namespace.
*
* @see Documentation
* on elastic.co
*/
public DeleteServiceTokenResponse deleteServiceToken(DeleteServiceTokenRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteServiceTokenRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete service account tokens.
*
* Delete service account tokens for a service in a specified namespace.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteServiceTokenRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteServiceTokenResponse deleteServiceToken(
Function> fn)
throws IOException, ElasticsearchException {
return deleteServiceToken(fn.apply(new DeleteServiceTokenRequest.Builder()).build());
}
// ----- Endpoint: security.delete_user
/**
* Delete users.
*
* Delete users from the native realm.
*
* @see Documentation
* on elastic.co
*/
public DeleteUserResponse deleteUser(DeleteUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Delete users.
*
* Delete users from the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteUserRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteUserResponse deleteUser(Function> fn)
throws IOException, ElasticsearchException {
return deleteUser(fn.apply(new DeleteUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user
/**
* Disable users.
*
* Disable users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public DisableUserResponse disableUser(DisableUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Disable users.
*
* Disable users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserRequest}
* @see Documentation
* on elastic.co
*/
public final DisableUserResponse disableUser(
Function> fn)
throws IOException, ElasticsearchException {
return disableUser(fn.apply(new DisableUserRequest.Builder()).build());
}
// ----- Endpoint: security.disable_user_profile
/**
* Disable a user profile.
*
* Disable user profiles so that they are not visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public DisableUserProfileResponse disableUserProfile(DisableUserProfileRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DisableUserProfileRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Disable a user profile.
*
* Disable user profiles so that they are not visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link DisableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final DisableUserProfileResponse disableUserProfile(
Function> fn)
throws IOException, ElasticsearchException {
return disableUserProfile(fn.apply(new DisableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user
/**
* Enable users.
*
* Enable users in the native realm.
*
* @see Documentation
* on elastic.co
*/
public EnableUserResponse enableUser(EnableUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Enable users.
*
* Enable users in the native realm.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserRequest}
* @see Documentation
* on elastic.co
*/
public final EnableUserResponse enableUser(Function> fn)
throws IOException, ElasticsearchException {
return enableUser(fn.apply(new EnableUserRequest.Builder()).build());
}
// ----- Endpoint: security.enable_user_profile
/**
* Enable a user profile.
*
* Enable user profiles to make them visible in user profile searches.
*
* @see Documentation
* on elastic.co
*/
public EnableUserProfileResponse enableUserProfile(EnableUserProfileRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EnableUserProfileRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Enable a user profile.
*
* Enable user profiles to make them visible in user profile searches.
*
* @param fn
* a function that initializes a builder to create the
* {@link EnableUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final EnableUserProfileResponse enableUserProfile(
Function> fn)
throws IOException, ElasticsearchException {
return enableUserProfile(fn.apply(new EnableUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.enroll_kibana
/**
* Enroll Kibana.
*
* Enable a Kibana instance to configure itself for communication with a secured
* Elasticsearch cluster.
*
* @see Documentation
* on elastic.co
*/
public EnrollKibanaResponse enrollKibana() throws IOException, ElasticsearchException {
return this.transport.performRequest(EnrollKibanaRequest._INSTANCE, EnrollKibanaRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.enroll_node
/**
* Enroll a node.
*
* Enroll a new node to allow it to join an existing cluster with security
* features enabled.
*
* @see Documentation
* on elastic.co
*/
public EnrollNodeResponse enrollNode() throws IOException, ElasticsearchException {
return this.transport.performRequest(EnrollNodeRequest._INSTANCE, EnrollNodeRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_api_key
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public GetApiKeyResponse getApiKey(GetApiKeyRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final GetApiKeyResponse getApiKey(Function> fn)
throws IOException, ElasticsearchException {
return getApiKey(fn.apply(new GetApiKeyRequest.Builder()).build());
}
/**
* Get API key information.
*
* Retrieves information for one or more API keys. NOTE: If you have only the
* manage_own_api_key
privilege, this API returns only the API keys
* that you own. If you have read_security
,
* manage_api_key
or greater privileges (including
* manage_security
), this API returns all API keys regardless of
* ownership.
*
* @see Documentation
* on elastic.co
*/
public GetApiKeyResponse getApiKey() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetApiKeyRequest.Builder().build(), GetApiKeyRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_builtin_privileges
/**
* Get builtin privileges.
*
* Get the list of cluster privileges and index privileges that are available in
* this version of Elasticsearch.
*
* @see Documentation
* on elastic.co
*/
public GetBuiltinPrivilegesResponse getBuiltinPrivileges() throws IOException, ElasticsearchException {
return this.transport.performRequest(GetBuiltinPrivilegesRequest._INSTANCE,
GetBuiltinPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_privileges
/**
* Get application privileges.
*
* @see Documentation
* on elastic.co
*/
public GetPrivilegesResponse getPrivileges(GetPrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetPrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final GetPrivilegesResponse getPrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return getPrivileges(fn.apply(new GetPrivilegesRequest.Builder()).build());
}
/**
* Get application privileges.
*
* @see Documentation
* on elastic.co
*/
public GetPrivilegesResponse getPrivileges() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetPrivilegesRequest.Builder().build(), GetPrivilegesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_role
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public GetRoleResponse getRole(GetRoleRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleRequest}
* @see Documentation
* on elastic.co
*/
public final GetRoleResponse getRole(Function> fn)
throws IOException, ElasticsearchException {
return getRole(fn.apply(new GetRoleRequest.Builder()).build());
}
/**
* Get roles.
*
* Get roles in the native realm. The role management APIs are generally the
* preferred way to manage roles, rather than using file-based role management.
* The get roles API cannot retrieve roles that are defined in roles files.
*
* @see Documentation
* on elastic.co
*/
public GetRoleResponse getRole() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetRoleRequest.Builder().build(), GetRoleRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_role_mapping
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @see Documentation
* on elastic.co
*/
public GetRoleMappingResponse getRoleMapping(GetRoleMappingRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRoleMappingRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final GetRoleMappingResponse getRoleMapping(
Function> fn)
throws IOException, ElasticsearchException {
return getRoleMapping(fn.apply(new GetRoleMappingRequest.Builder()).build());
}
/**
* Get role mappings.
*
* Role mappings define which roles are assigned to each user. The role mapping
* APIs are generally the preferred way to manage role mappings rather than
* using role mapping files. The get role mappings API cannot retrieve role
* mappings that are defined in role mapping files.
*
* @see Documentation
* on elastic.co
*/
public GetRoleMappingResponse getRoleMapping() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetRoleMappingRequest.Builder().build(),
GetRoleMappingRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_accounts
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @see Documentation
* on elastic.co
*/
public GetServiceAccountsResponse getServiceAccounts(GetServiceAccountsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceAccountsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceAccountsRequest}
* @see Documentation
* on elastic.co
*/
public final GetServiceAccountsResponse getServiceAccounts(
Function> fn)
throws IOException, ElasticsearchException {
return getServiceAccounts(fn.apply(new GetServiceAccountsRequest.Builder()).build());
}
/**
* Get service accounts.
*
* Get a list of service accounts that match the provided path parameters.
*
* @see Documentation
* on elastic.co
*/
public GetServiceAccountsResponse getServiceAccounts() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetServiceAccountsRequest.Builder().build(),
GetServiceAccountsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_service_credentials
/**
* Get service account credentials.
*
* @see Documentation
* on elastic.co
*/
public GetServiceCredentialsResponse getServiceCredentials(GetServiceCredentialsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetServiceCredentialsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get service account credentials.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetServiceCredentialsRequest}
* @see Documentation
* on elastic.co
*/
public final GetServiceCredentialsResponse getServiceCredentials(
Function> fn)
throws IOException, ElasticsearchException {
return getServiceCredentials(fn.apply(new GetServiceCredentialsRequest.Builder()).build());
}
// ----- Endpoint: security.get_token
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public GetTokenResponse getToken(GetTokenRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTokenRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTokenRequest}
* @see Documentation
* on elastic.co
*/
public final GetTokenResponse getToken(Function> fn)
throws IOException, ElasticsearchException {
return getToken(fn.apply(new GetTokenRequest.Builder()).build());
}
/**
* Get a token.
*
* Create a bearer token for access without requiring basic authentication.
*
* @see Documentation
* on elastic.co
*/
public GetTokenResponse getToken() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetTokenRequest.Builder().build(), GetTokenRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public GetUserResponse getUser(GetUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserRequest}
* @see Documentation
* on elastic.co
*/
public final GetUserResponse getUser(Function> fn)
throws IOException, ElasticsearchException {
return getUser(fn.apply(new GetUserRequest.Builder()).build());
}
/**
* Get users.
*
* Get information about users in the native realm and built-in users.
*
* @see Documentation
* on elastic.co
*/
public GetUserResponse getUser() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetUserRequest.Builder().build(), GetUserRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.get_user_privileges
/**
* Get user privileges.
*
* @see Documentation
* on elastic.co
*/
public GetUserPrivilegesResponse getUserPrivileges(GetUserPrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserPrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get user privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final GetUserPrivilegesResponse getUserPrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return getUserPrivileges(fn.apply(new GetUserPrivilegesRequest.Builder()).build());
}
/**
* Get user privileges.
*
* @see Documentation
* on elastic.co
*/
public GetUserPrivilegesResponse getUserPrivileges() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetUserPrivilegesRequest.Builder().build(),
GetUserPrivilegesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.get_user_profile
/**
* Get a user profile.
*
* Get a user's profile using the unique profile ID.
*
* @see Documentation
* on elastic.co
*/
public GetUserProfileResponse getUserProfile(GetUserProfileRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetUserProfileRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Get a user profile.
*
* Get a user's profile using the unique profile ID.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final GetUserProfileResponse getUserProfile(
Function> fn)
throws IOException, ElasticsearchException {
return getUserProfile(fn.apply(new GetUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.grant_api_key
/**
* Grant an API key.
*
* Create an API key on behalf of another user. This API is similar to the
* create API keys API, however it creates the API key for a user that is
* different than the user that runs the API. The caller must have
* authentication credentials (either an access token, or a username and
* password) for the user on whose behalf the API key will be created. It is not
* possible to use this API to create an API key without that user’s
* credentials. The user, for whom the authentication credentials is provided,
* can optionally "run as" (impersonate) another user. In this case,
* the API key will be created on behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @see Documentation
* on elastic.co
*/
public GrantApiKeyResponse grantApiKey(GrantApiKeyRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GrantApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Grant an API key.
*
* Create an API key on behalf of another user. This API is similar to the
* create API keys API, however it creates the API key for a user that is
* different than the user that runs the API. The caller must have
* authentication credentials (either an access token, or a username and
* password) for the user on whose behalf the API key will be created. It is not
* possible to use this API to create an API key without that user’s
* credentials. The user, for whom the authentication credentials is provided,
* can optionally "run as" (impersonate) another user. In this case,
* the API key will be created on behalf of the impersonated user.
*
* This API is intended be used by applications that need to create and manage
* API keys for end users, but cannot guarantee that those users have permission
* to create API keys on their own behalf.
*
* A successful grant API key API call returns a JSON structure that contains
* the API key, its unique id, and its name. If applicable, it also returns
* expiration information for the API key in milliseconds.
*
* By default, API keys never expire. You can specify expiration information
* when you create the API keys.
*
* @param fn
* a function that initializes a builder to create the
* {@link GrantApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final GrantApiKeyResponse grantApiKey(
Function> fn)
throws IOException, ElasticsearchException {
return grantApiKey(fn.apply(new GrantApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.has_privileges
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public HasPrivilegesResponse hasPrivileges(HasPrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HasPrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link HasPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final HasPrivilegesResponse hasPrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return hasPrivileges(fn.apply(new HasPrivilegesRequest.Builder()).build());
}
/**
* Check user privileges.
*
* Determine whether the specified user has a specified list of privileges.
*
* @see Documentation
* on elastic.co
*/
public HasPrivilegesResponse hasPrivileges() throws IOException, ElasticsearchException {
return this.transport.performRequest(new HasPrivilegesRequest.Builder().build(), HasPrivilegesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.has_privileges_user_profile
/**
* Check user profile privileges.
*
* Determine whether the users associated with the specified user profile IDs
* have all the requested privileges.
*
* @see Documentation
* on elastic.co
*/
public HasPrivilegesUserProfileResponse hasPrivilegesUserProfile(HasPrivilegesUserProfileRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HasPrivilegesUserProfileRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Check user profile privileges.
*
* Determine whether the users associated with the specified user profile IDs
* have all the requested privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link HasPrivilegesUserProfileRequest}
* @see Documentation
* on elastic.co
*/
public final HasPrivilegesUserProfileResponse hasPrivilegesUserProfile(
Function> fn)
throws IOException, ElasticsearchException {
return hasPrivilegesUserProfile(fn.apply(new HasPrivilegesUserProfileRequest.Builder()).build());
}
// ----- Endpoint: security.invalidate_api_key
/**
* Invalidate API keys.
*
* This API invalidates API keys created by the create API key or grant API key
* APIs. Invalidated API keys fail authentication, but they can still be viewed
* using the get API key information and query API key information APIs, for at
* least the configured retention period, until they are automatically deleted.
* The manage_api_key
privilege allows deleting any API keys. The
* manage_own_api_key
only allows deleting API keys that are owned
* by the user. In addition, with the manage_own_api_key
privilege,
* an invalidation request must be issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, that is to say an API key
* invalidates itself, specify its ID in the
ids
field.
*
*
* @see Documentation
* on elastic.co
*/
public InvalidateApiKeyResponse invalidateApiKey(InvalidateApiKeyRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InvalidateApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Invalidate API keys.
*
* This API invalidates API keys created by the create API key or grant API key
* APIs. Invalidated API keys fail authentication, but they can still be viewed
* using the get API key information and query API key information APIs, for at
* least the configured retention period, until they are automatically deleted.
* The manage_api_key
privilege allows deleting any API keys. The
* manage_own_api_key
only allows deleting API keys that are owned
* by the user. In addition, with the manage_own_api_key
privilege,
* an invalidation request must be issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, that is to say an API key
* invalidates itself, specify its ID in the
ids
field.
*
*
* @param fn
* a function that initializes a builder to create the
* {@link InvalidateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final InvalidateApiKeyResponse invalidateApiKey(
Function> fn)
throws IOException, ElasticsearchException {
return invalidateApiKey(fn.apply(new InvalidateApiKeyRequest.Builder()).build());
}
/**
* Invalidate API keys.
*
* This API invalidates API keys created by the create API key or grant API key
* APIs. Invalidated API keys fail authentication, but they can still be viewed
* using the get API key information and query API key information APIs, for at
* least the configured retention period, until they are automatically deleted.
* The manage_api_key
privilege allows deleting any API keys. The
* manage_own_api_key
only allows deleting API keys that are owned
* by the user. In addition, with the manage_own_api_key
privilege,
* an invalidation request must be issued in one of the three formats:
*
* - Set the parameter
owner=true
.
* - Or, set both
username
and realm_name
to match
* the user’s identity.
* - Or, if the request is issued by an API key, that is to say an API key
* invalidates itself, specify its ID in the
ids
field.
*
*
* @see Documentation
* on elastic.co
*/
public InvalidateApiKeyResponse invalidateApiKey() throws IOException, ElasticsearchException {
return this.transport.performRequest(new InvalidateApiKeyRequest.Builder().build(),
InvalidateApiKeyRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.invalidate_token
/**
* Invalidate a token.
*
* The access tokens returned by the get token API have a finite period of time
* for which they are valid. After that time period, they can no longer be used.
* The time period is defined by the
* xpack.security.authc.token.timeout
setting.
*
* The refresh tokens returned by the get token API are only valid for 24 hours.
* They can also be used exactly once. If you want to invalidate one or more
* access or refresh tokens immediately, use this invalidate token API.
*
* @see Documentation
* on elastic.co
*/
public InvalidateTokenResponse invalidateToken(InvalidateTokenRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InvalidateTokenRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Invalidate a token.
*
* The access tokens returned by the get token API have a finite period of time
* for which they are valid. After that time period, they can no longer be used.
* The time period is defined by the
* xpack.security.authc.token.timeout
setting.
*
* The refresh tokens returned by the get token API are only valid for 24 hours.
* They can also be used exactly once. If you want to invalidate one or more
* access or refresh tokens immediately, use this invalidate token API.
*
* @param fn
* a function that initializes a builder to create the
* {@link InvalidateTokenRequest}
* @see Documentation
* on elastic.co
*/
public final InvalidateTokenResponse invalidateToken(
Function> fn)
throws IOException, ElasticsearchException {
return invalidateToken(fn.apply(new InvalidateTokenRequest.Builder()).build());
}
/**
* Invalidate a token.
*
* The access tokens returned by the get token API have a finite period of time
* for which they are valid. After that time period, they can no longer be used.
* The time period is defined by the
* xpack.security.authc.token.timeout
setting.
*
* The refresh tokens returned by the get token API are only valid for 24 hours.
* They can also be used exactly once. If you want to invalidate one or more
* access or refresh tokens immediately, use this invalidate token API.
*
* @see Documentation
* on elastic.co
*/
public InvalidateTokenResponse invalidateToken() throws IOException, ElasticsearchException {
return this.transport.performRequest(new InvalidateTokenRequest.Builder().build(),
InvalidateTokenRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.put_privileges
/**
* Create or update application privileges.
*
* @see Documentation
* on elastic.co
*/
public PutPrivilegesResponse putPrivileges(PutPrivilegesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutPrivilegesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create or update application privileges.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutPrivilegesRequest}
* @see Documentation
* on elastic.co
*/
public final PutPrivilegesResponse putPrivileges(
Function> fn)
throws IOException, ElasticsearchException {
return putPrivileges(fn.apply(new PutPrivilegesRequest.Builder()).build());
}
/**
* Create or update application privileges.
*
* @see Documentation
* on elastic.co
*/
public PutPrivilegesResponse putPrivileges() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PutPrivilegesRequest.Builder().build(), PutPrivilegesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.put_role
/**
* Create or update roles.
*
* The role management APIs are generally the preferred way to manage roles in
* the native realm, rather than using file-based role management. The create or
* update roles API cannot update roles that are defined in roles files.
* File-based role management is not available in Elastic Serverless.
*
* @see Documentation
* on elastic.co
*/
public PutRoleResponse putRole(PutRoleRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create or update roles.
*
* The role management APIs are generally the preferred way to manage roles in
* the native realm, rather than using file-based role management. The create or
* update roles API cannot update roles that are defined in roles files.
* File-based role management is not available in Elastic Serverless.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutRoleRequest}
* @see Documentation
* on elastic.co
*/
public final PutRoleResponse putRole(Function> fn)
throws IOException, ElasticsearchException {
return putRole(fn.apply(new PutRoleRequest.Builder()).build());
}
// ----- Endpoint: security.put_role_mapping
/**
* Create or update role mappings.
*
* Role mappings define which roles are assigned to each user. Each mapping has
* rules that identify users and a list of roles that are granted to those
* users. The role mapping APIs are generally the preferred way to manage role
* mappings rather than using role mapping files. The create or update role
* mappings API cannot update role mappings that are defined in role mapping
* files.
*
* This API does not create roles. Rather, it maps users to existing roles.
* Roles can be created by using the create or update roles API or roles files.
*
* @see Documentation
* on elastic.co
*/
public PutRoleMappingResponse putRoleMapping(PutRoleMappingRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutRoleMappingRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create or update role mappings.
*
* Role mappings define which roles are assigned to each user. Each mapping has
* rules that identify users and a list of roles that are granted to those
* users. The role mapping APIs are generally the preferred way to manage role
* mappings rather than using role mapping files. The create or update role
* mappings API cannot update role mappings that are defined in role mapping
* files.
*
* This API does not create roles. Rather, it maps users to existing roles.
* Roles can be created by using the create or update roles API or roles files.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutRoleMappingRequest}
* @see Documentation
* on elastic.co
*/
public final PutRoleMappingResponse putRoleMapping(
Function> fn)
throws IOException, ElasticsearchException {
return putRoleMapping(fn.apply(new PutRoleMappingRequest.Builder()).build());
}
// ----- Endpoint: security.put_user
/**
* Create or update users.
*
* A password is required for adding a new user but is optional when updating an
* existing user. To change a user’s password without updating any other fields,
* use the change password API.
*
* @see Documentation
* on elastic.co
*/
public PutUserResponse putUser(PutUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create or update users.
*
* A password is required for adding a new user but is optional when updating an
* existing user. To change a user’s password without updating any other fields,
* use the change password API.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutUserRequest}
* @see Documentation
* on elastic.co
*/
public final PutUserResponse putUser(Function> fn)
throws IOException, ElasticsearchException {
return putUser(fn.apply(new PutUserRequest.Builder()).build());
}
// ----- Endpoint: security.query_api_keys
/**
* Find API keys with a query.
*
* Get a paginated list of API keys and their information. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public QueryApiKeysResponse queryApiKeys(QueryApiKeysRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryApiKeysRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Find API keys with a query.
*
* Get a paginated list of API keys and their information. You can optionally
* filter the results with a query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryApiKeysRequest}
* @see Documentation
* on elastic.co
*/
public final QueryApiKeysResponse queryApiKeys(
Function> fn)
throws IOException, ElasticsearchException {
return queryApiKeys(fn.apply(new QueryApiKeysRequest.Builder()).build());
}
/**
* Find API keys with a query.
*
* Get a paginated list of API keys and their information. You can optionally
* filter the results with a query.
*
* @see Documentation
* on elastic.co
*/
public QueryApiKeysResponse queryApiKeys() throws IOException, ElasticsearchException {
return this.transport.performRequest(new QueryApiKeysRequest.Builder().build(), QueryApiKeysRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.query_role
/**
* Find roles with a query.
*
* Get roles in a paginated manner. You can optionally filter the results with a
* query.
*
* @see Documentation
* on elastic.co
*/
public QueryRoleResponse queryRole(QueryRoleRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryRoleRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Find roles with a query.
*
* Get roles in a paginated manner. You can optionally filter the results with a
* query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryRoleRequest}
* @see Documentation
* on elastic.co
*/
public final QueryRoleResponse queryRole(Function> fn)
throws IOException, ElasticsearchException {
return queryRole(fn.apply(new QueryRoleRequest.Builder()).build());
}
/**
* Find roles with a query.
*
* Get roles in a paginated manner. You can optionally filter the results with a
* query.
*
* @see Documentation
* on elastic.co
*/
public QueryRoleResponse queryRole() throws IOException, ElasticsearchException {
return this.transport.performRequest(new QueryRoleRequest.Builder().build(), QueryRoleRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.query_user
/**
* Find users with a query.
*
* Get information for users in a paginated manner. You can optionally filter
* the results with a query.
*
* @see Documentation
* on elastic.co
*/
public QueryUserResponse queryUser(QueryUserRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryUserRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Find users with a query.
*
* Get information for users in a paginated manner. You can optionally filter
* the results with a query.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryUserRequest}
* @see Documentation
* on elastic.co
*/
public final QueryUserResponse queryUser(Function> fn)
throws IOException, ElasticsearchException {
return queryUser(fn.apply(new QueryUserRequest.Builder()).build());
}
/**
* Find users with a query.
*
* Get information for users in a paginated manner. You can optionally filter
* the results with a query.
*
* @see Documentation
* on elastic.co
*/
public QueryUserResponse queryUser() throws IOException, ElasticsearchException {
return this.transport.performRequest(new QueryUserRequest.Builder().build(), QueryUserRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: security.saml_authenticate
/**
* Authenticate SAML.
*
* Submits a SAML response message to Elasticsearch for consumption.
*
* @see Documentation
* on elastic.co
*/
public SamlAuthenticateResponse samlAuthenticate(SamlAuthenticateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlAuthenticateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Authenticate SAML.
*
* Submits a SAML response message to Elasticsearch for consumption.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlAuthenticateRequest}
* @see Documentation
* on elastic.co
*/
public final SamlAuthenticateResponse samlAuthenticate(
Function> fn)
throws IOException, ElasticsearchException {
return samlAuthenticate(fn.apply(new SamlAuthenticateRequest.Builder()).build());
}
// ----- Endpoint: security.saml_complete_logout
/**
* Logout of SAML completely.
*
* Verifies the logout response sent from the SAML IdP.
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse samlCompleteLogout(SamlCompleteLogoutRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
Endpoint endpoint = (Endpoint) SamlCompleteLogoutRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Logout of SAML completely.
*
* Verifies the logout response sent from the SAML IdP.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlCompleteLogoutRequest}
* @see Documentation
* on elastic.co
*/
public final BooleanResponse samlCompleteLogout(
Function> fn)
throws IOException, ElasticsearchException {
return samlCompleteLogout(fn.apply(new SamlCompleteLogoutRequest.Builder()).build());
}
// ----- Endpoint: security.saml_invalidate
/**
* Invalidate SAML.
*
* Submits a SAML LogoutRequest message to Elasticsearch for consumption.
*
* @see Documentation
* on elastic.co
*/
public SamlInvalidateResponse samlInvalidate(SamlInvalidateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlInvalidateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Invalidate SAML.
*
* Submits a SAML LogoutRequest message to Elasticsearch for consumption.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlInvalidateRequest}
* @see Documentation
* on elastic.co
*/
public final SamlInvalidateResponse samlInvalidate(
Function> fn)
throws IOException, ElasticsearchException {
return samlInvalidate(fn.apply(new SamlInvalidateRequest.Builder()).build());
}
// ----- Endpoint: security.saml_logout
/**
* Logout of SAML.
*
* Submits a request to invalidate an access token and refresh token.
*
* @see Documentation
* on elastic.co
*/
public SamlLogoutResponse samlLogout(SamlLogoutRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlLogoutRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Logout of SAML.
*
* Submits a request to invalidate an access token and refresh token.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlLogoutRequest}
* @see Documentation
* on elastic.co
*/
public final SamlLogoutResponse samlLogout(Function> fn)
throws IOException, ElasticsearchException {
return samlLogout(fn.apply(new SamlLogoutRequest.Builder()).build());
}
// ----- Endpoint: security.saml_prepare_authentication
/**
* Prepare SAML authentication.
*
* Creates a SAML authentication request (<AuthnRequest>
) as
* a URL string, based on the configuration of the respective SAML realm in
* Elasticsearch.
*
* @see Documentation
* on elastic.co
*/
public SamlPrepareAuthenticationResponse samlPrepareAuthentication(SamlPrepareAuthenticationRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlPrepareAuthenticationRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Prepare SAML authentication.
*
* Creates a SAML authentication request (<AuthnRequest>
) as
* a URL string, based on the configuration of the respective SAML realm in
* Elasticsearch.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlPrepareAuthenticationRequest}
* @see Documentation
* on elastic.co
*/
public final SamlPrepareAuthenticationResponse samlPrepareAuthentication(
Function> fn)
throws IOException, ElasticsearchException {
return samlPrepareAuthentication(fn.apply(new SamlPrepareAuthenticationRequest.Builder()).build());
}
/**
* Prepare SAML authentication.
*
* Creates a SAML authentication request (<AuthnRequest>
) as
* a URL string, based on the configuration of the respective SAML realm in
* Elasticsearch.
*
* @see Documentation
* on elastic.co
*/
public SamlPrepareAuthenticationResponse samlPrepareAuthentication() throws IOException, ElasticsearchException {
return this.transport.performRequest(new SamlPrepareAuthenticationRequest.Builder().build(),
SamlPrepareAuthenticationRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.saml_service_provider_metadata
/**
* Create SAML service provider metadata.
*
* Generate SAML metadata for a SAML 2.0 Service Provider.
*
* @see Documentation
* on elastic.co
*/
public SamlServiceProviderMetadataResponse samlServiceProviderMetadata(SamlServiceProviderMetadataRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SamlServiceProviderMetadataRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Create SAML service provider metadata.
*
* Generate SAML metadata for a SAML 2.0 Service Provider.
*
* @param fn
* a function that initializes a builder to create the
* {@link SamlServiceProviderMetadataRequest}
* @see Documentation
* on elastic.co
*/
public final SamlServiceProviderMetadataResponse samlServiceProviderMetadata(
Function> fn)
throws IOException, ElasticsearchException {
return samlServiceProviderMetadata(fn.apply(new SamlServiceProviderMetadataRequest.Builder()).build());
}
// ----- Endpoint: security.suggest_user_profiles
/**
* Suggest a user profile.
*
* Get suggestions for user profiles that match specified search criteria.
*
* @see Documentation
* on elastic.co
*/
public SuggestUserProfilesResponse suggestUserProfiles(SuggestUserProfilesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SuggestUserProfilesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Suggest a user profile.
*
* Get suggestions for user profiles that match specified search criteria.
*
* @param fn
* a function that initializes a builder to create the
* {@link SuggestUserProfilesRequest}
* @see Documentation
* on elastic.co
*/
public final SuggestUserProfilesResponse suggestUserProfiles(
Function> fn)
throws IOException, ElasticsearchException {
return suggestUserProfiles(fn.apply(new SuggestUserProfilesRequest.Builder()).build());
}
/**
* Suggest a user profile.
*
* Get suggestions for user profiles that match specified search criteria.
*
* @see Documentation
* on elastic.co
*/
public SuggestUserProfilesResponse suggestUserProfiles() throws IOException, ElasticsearchException {
return this.transport.performRequest(new SuggestUserProfilesRequest.Builder().build(),
SuggestUserProfilesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: security.update_api_key
/**
* Update an API key.
*
* Updates attributes of an existing API key. Users can only update API keys
* that they created or that were granted to them. Use this API to update API
* keys created by the create API Key or grant API Key APIs. If you need to
* apply the same update to many API keys, you can use bulk update API Keys to
* reduce overhead. It’s not possible to update expired API keys, or API keys
* that have been invalidated by invalidate API Key. This API supports updates
* to an API key’s access scope and metadata. The access scope of an API key is
* derived from the role_descriptors
you specify in the request,
* and a snapshot of the owner user’s permissions at the time of the request.
* The snapshot of the owner’s permissions is updated automatically on every
* call. If you don’t specify role_descriptors
in the request, a
* call to this API might still change the API key’s access scope. This change
* can occur if the owner user’s permissions have changed since the API key was
* created or last modified. To update another user’s API key, use the
* run_as
feature to submit a request on behalf of another user.
* IMPORTANT: It’s not possible to use an API key as the authentication
* credential for this API. To update an API key, the owner user’s credentials
* are required.
*
* @see Documentation
* on elastic.co
*/
public UpdateApiKeyResponse updateApiKey(UpdateApiKeyRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update an API key.
*
* Updates attributes of an existing API key. Users can only update API keys
* that they created or that were granted to them. Use this API to update API
* keys created by the create API Key or grant API Key APIs. If you need to
* apply the same update to many API keys, you can use bulk update API Keys to
* reduce overhead. It’s not possible to update expired API keys, or API keys
* that have been invalidated by invalidate API Key. This API supports updates
* to an API key’s access scope and metadata. The access scope of an API key is
* derived from the role_descriptors
you specify in the request,
* and a snapshot of the owner user’s permissions at the time of the request.
* The snapshot of the owner’s permissions is updated automatically on every
* call. If you don’t specify role_descriptors
in the request, a
* call to this API might still change the API key’s access scope. This change
* can occur if the owner user’s permissions have changed since the API key was
* created or last modified. To update another user’s API key, use the
* run_as
feature to submit a request on behalf of another user.
* IMPORTANT: It’s not possible to use an API key as the authentication
* credential for this API. To update an API key, the owner user’s credentials
* are required.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateApiKeyRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateApiKeyResponse updateApiKey(
Function> fn)
throws IOException, ElasticsearchException {
return updateApiKey(fn.apply(new UpdateApiKeyRequest.Builder()).build());
}
// ----- Endpoint: security.update_cross_cluster_api_key
/**
* Update a cross-cluster API key.
*
* Update the attributes of an existing cross-cluster API key, which is used for
* API key based remote cluster access.
*
* @see Documentation
* on elastic.co
*/
public UpdateCrossClusterApiKeyResponse updateCrossClusterApiKey(UpdateCrossClusterApiKeyRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateCrossClusterApiKeyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Update a cross-cluster API key.
*
* Update the attributes of an existing cross-cluster API key, which is used for
* API key based remote cluster access.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateCrossClusterApiKeyRequest}
* @see