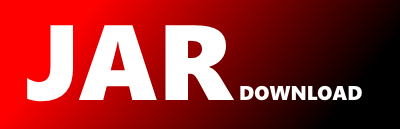
co.elastic.clients.elasticsearch.transform.UpdateTransformRequest Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.elasticsearch.transform;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.elasticsearch._types.RequestBase;
import co.elastic.clients.elasticsearch._types.Time;
import co.elastic.clients.json.JsonData;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.endpoints.SimpleEndpoint;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import jakarta.json.stream.JsonGenerator;
import java.lang.Boolean;
import java.lang.String;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import javax.annotation.Nullable;
//----------------------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------------------
//
// This code is generated from the Elasticsearch API specification
// at https://github.com/elastic/elasticsearch-specification
//
// Manual updates to this file will be lost when the code is
// re-generated.
//
// If you find a property that is missing or wrongly typed, please
// open an issue or a PR on the API specification repository.
//
//----------------------------------------------------------------
// typedef: transform.update_transform.Request
/**
* Update a transform. Updates certain properties of a transform.
*
* All updated properties except description
do not take effect
* until after the transform starts the next checkpoint, thus there is data
* consistency in each checkpoint. To use this API, you must have
* read
and view_index_metadata
privileges for the
* source indices. You must also have index
and read
* privileges for the destination index. When Elasticsearch security features
* are enabled, the transform remembers which roles the user who updated it had
* at the time of update and runs with those privileges.
*
* @see API
* specification
*/
@JsonpDeserializable
public class UpdateTransformRequest extends RequestBase implements JsonpSerializable {
private final Map meta;
@Nullable
private final Boolean deferValidation;
@Nullable
private final String description;
@Nullable
private final Destination dest;
@Nullable
private final Time frequency;
@Nullable
private final RetentionPolicy retentionPolicy;
@Nullable
private final Settings settings;
@Nullable
private final Source source;
@Nullable
private final Sync sync;
@Nullable
private final Time timeout;
private final String transformId;
// ---------------------------------------------------------------------------------------------
private UpdateTransformRequest(Builder builder) {
this.meta = ApiTypeHelper.unmodifiable(builder.meta);
this.deferValidation = builder.deferValidation;
this.description = builder.description;
this.dest = builder.dest;
this.frequency = builder.frequency;
this.retentionPolicy = builder.retentionPolicy;
this.settings = builder.settings;
this.source = builder.source;
this.sync = builder.sync;
this.timeout = builder.timeout;
this.transformId = ApiTypeHelper.requireNonNull(builder.transformId, this, "transformId");
}
public static UpdateTransformRequest of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* Defines optional transform metadata.
*
* API name: {@code _meta}
*/
public final Map meta() {
return this.meta;
}
/**
* When true, deferrable validations are not run. This behavior may be desired
* if the source index does not exist until after the transform is created.
*
* API name: {@code defer_validation}
*/
@Nullable
public final Boolean deferValidation() {
return this.deferValidation;
}
/**
* Free text description of the transform.
*
* API name: {@code description}
*/
@Nullable
public final String description() {
return this.description;
}
/**
* The destination for the transform.
*
* API name: {@code dest}
*/
@Nullable
public final Destination dest() {
return this.dest;
}
/**
* The interval between checks for changes in the source indices when the
* transform is running continuously. Also determines the retry interval in the
* event of transient failures while the transform is searching or indexing. The
* minimum value is 1s and the maximum is 1h.
*
* API name: {@code frequency}
*/
@Nullable
public final Time frequency() {
return this.frequency;
}
/**
* Defines a retention policy for the transform. Data that meets the defined
* criteria is deleted from the destination index.
*
* API name: {@code retention_policy}
*/
@Nullable
public final RetentionPolicy retentionPolicy() {
return this.retentionPolicy;
}
/**
* Defines optional transform settings.
*
* API name: {@code settings}
*/
@Nullable
public final Settings settings() {
return this.settings;
}
/**
* The source of the data for the transform.
*
* API name: {@code source}
*/
@Nullable
public final Source source() {
return this.source;
}
/**
* Defines the properties transforms require to run continuously.
*
* API name: {@code sync}
*/
@Nullable
public final Sync sync() {
return this.sync;
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
@Nullable
public final Time timeout() {
return this.timeout;
}
/**
* Required - Identifier for the transform.
*
* API name: {@code transform_id}
*/
public final String transformId() {
return this.transformId;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (ApiTypeHelper.isDefined(this.meta)) {
generator.writeKey("_meta");
generator.writeStartObject();
for (Map.Entry item0 : this.meta.entrySet()) {
generator.writeKey(item0.getKey());
item0.getValue().serialize(generator, mapper);
}
generator.writeEnd();
}
if (this.description != null) {
generator.writeKey("description");
generator.write(this.description);
}
if (this.dest != null) {
generator.writeKey("dest");
this.dest.serialize(generator, mapper);
}
if (this.frequency != null) {
generator.writeKey("frequency");
this.frequency.serialize(generator, mapper);
}
if (this.retentionPolicy != null) {
generator.writeKey("retention_policy");
this.retentionPolicy.serialize(generator, mapper);
}
if (this.settings != null) {
generator.writeKey("settings");
this.settings.serialize(generator, mapper);
}
if (this.source != null) {
generator.writeKey("source");
this.source.serialize(generator, mapper);
}
if (this.sync != null) {
generator.writeKey("sync");
this.sync.serialize(generator, mapper);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link UpdateTransformRequest}.
*/
public static class Builder extends RequestBase.AbstractBuilder
implements
ObjectBuilder {
@Nullable
private Map meta;
@Nullable
private Boolean deferValidation;
@Nullable
private String description;
@Nullable
private Destination dest;
@Nullable
private Time frequency;
@Nullable
private RetentionPolicy retentionPolicy;
@Nullable
private Settings settings;
@Nullable
private Source source;
@Nullable
private Sync sync;
@Nullable
private Time timeout;
private String transformId;
/**
* Defines optional transform metadata.
*
* API name: {@code _meta}
*
* Adds all entries of map
to meta
.
*/
public final Builder meta(Map map) {
this.meta = _mapPutAll(this.meta, map);
return this;
}
/**
* Defines optional transform metadata.
*
* API name: {@code _meta}
*
* Adds an entry to meta
.
*/
public final Builder meta(String key, JsonData value) {
this.meta = _mapPut(this.meta, key, value);
return this;
}
/**
* When true, deferrable validations are not run. This behavior may be desired
* if the source index does not exist until after the transform is created.
*
* API name: {@code defer_validation}
*/
public final Builder deferValidation(@Nullable Boolean value) {
this.deferValidation = value;
return this;
}
/**
* Free text description of the transform.
*
* API name: {@code description}
*/
public final Builder description(@Nullable String value) {
this.description = value;
return this;
}
/**
* The destination for the transform.
*
* API name: {@code dest}
*/
public final Builder dest(@Nullable Destination value) {
this.dest = value;
return this;
}
/**
* The destination for the transform.
*
* API name: {@code dest}
*/
public final Builder dest(Function> fn) {
return this.dest(fn.apply(new Destination.Builder()).build());
}
/**
* The interval between checks for changes in the source indices when the
* transform is running continuously. Also determines the retry interval in the
* event of transient failures while the transform is searching or indexing. The
* minimum value is 1s and the maximum is 1h.
*
* API name: {@code frequency}
*/
public final Builder frequency(@Nullable Time value) {
this.frequency = value;
return this;
}
/**
* The interval between checks for changes in the source indices when the
* transform is running continuously. Also determines the retry interval in the
* event of transient failures while the transform is searching or indexing. The
* minimum value is 1s and the maximum is 1h.
*
* API name: {@code frequency}
*/
public final Builder frequency(Function> fn) {
return this.frequency(fn.apply(new Time.Builder()).build());
}
/**
* Defines a retention policy for the transform. Data that meets the defined
* criteria is deleted from the destination index.
*
* API name: {@code retention_policy}
*/
public final Builder retentionPolicy(@Nullable RetentionPolicy value) {
this.retentionPolicy = value;
return this;
}
/**
* Defines a retention policy for the transform. Data that meets the defined
* criteria is deleted from the destination index.
*
* API name: {@code retention_policy}
*/
public final Builder retentionPolicy(Function> fn) {
return this.retentionPolicy(fn.apply(new RetentionPolicy.Builder()).build());
}
/**
* Defines optional transform settings.
*
* API name: {@code settings}
*/
public final Builder settings(@Nullable Settings value) {
this.settings = value;
return this;
}
/**
* Defines optional transform settings.
*
* API name: {@code settings}
*/
public final Builder settings(Function> fn) {
return this.settings(fn.apply(new Settings.Builder()).build());
}
/**
* The source of the data for the transform.
*
* API name: {@code source}
*/
public final Builder source(@Nullable Source value) {
this.source = value;
return this;
}
/**
* The source of the data for the transform.
*
* API name: {@code source}
*/
public final Builder source(Function> fn) {
return this.source(fn.apply(new Source.Builder()).build());
}
/**
* Defines the properties transforms require to run continuously.
*
* API name: {@code sync}
*/
public final Builder sync(@Nullable Sync value) {
this.sync = value;
return this;
}
/**
* Defines the properties transforms require to run continuously.
*
* API name: {@code sync}
*/
public final Builder sync(Function> fn) {
return this.sync(fn.apply(new Sync.Builder()).build());
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
public final Builder timeout(@Nullable Time value) {
this.timeout = value;
return this;
}
/**
* Period to wait for a response. If no response is received before the timeout
* expires, the request fails and returns an error.
*
* API name: {@code timeout}
*/
public final Builder timeout(Function> fn) {
return this.timeout(fn.apply(new Time.Builder()).build());
}
/**
* Required - Identifier for the transform.
*
* API name: {@code transform_id}
*/
public final Builder transformId(String value) {
this.transformId = value;
return this;
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link UpdateTransformRequest}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public UpdateTransformRequest build() {
_checkSingleUse();
return new UpdateTransformRequest(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link UpdateTransformRequest}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer
.lazy(Builder::new, UpdateTransformRequest::setupUpdateTransformRequestDeserializer);
protected static void setupUpdateTransformRequestDeserializer(
ObjectDeserializer op) {
op.add(Builder::meta, JsonpDeserializer.stringMapDeserializer(JsonData._DESERIALIZER), "_meta");
op.add(Builder::description, JsonpDeserializer.stringDeserializer(), "description");
op.add(Builder::dest, Destination._DESERIALIZER, "dest");
op.add(Builder::frequency, Time._DESERIALIZER, "frequency");
op.add(Builder::retentionPolicy, RetentionPolicy._DESERIALIZER, "retention_policy");
op.add(Builder::settings, Settings._DESERIALIZER, "settings");
op.add(Builder::source, Source._DESERIALIZER, "source");
op.add(Builder::sync, Sync._DESERIALIZER, "sync");
}
// ---------------------------------------------------------------------------------------------
/**
* Endpoint "{@code transform.update_transform}".
*/
public static final Endpoint _ENDPOINT = new SimpleEndpoint<>(
"es/transform.update_transform",
// Request method
request -> {
return "POST";
},
// Request path
request -> {
final int _transformId = 1 << 0;
int propsSet = 0;
propsSet |= _transformId;
if (propsSet == (_transformId)) {
StringBuilder buf = new StringBuilder();
buf.append("/_transform");
buf.append("/");
SimpleEndpoint.pathEncode(request.transformId, buf);
buf.append("/_update");
return buf.toString();
}
throw SimpleEndpoint.noPathTemplateFound("path");
},
// Path parameters
request -> {
Map params = new HashMap<>();
final int _transformId = 1 << 0;
int propsSet = 0;
propsSet |= _transformId;
if (propsSet == (_transformId)) {
params.put("transformId", request.transformId);
}
return params;
},
// Request parameters
request -> {
Map params = new HashMap<>();
if (request.deferValidation != null) {
params.put("defer_validation", String.valueOf(request.deferValidation));
}
if (request.timeout != null) {
params.put("timeout", request.timeout._toJsonString());
}
return params;
}, SimpleEndpoint.emptyMap(), true, UpdateTransformResponse._DESERIALIZER);
}