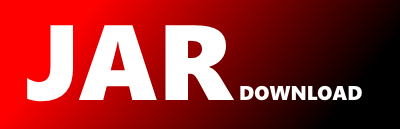
co.elastic.clients.elasticsearch.cat.ElasticsearchCatAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.cat;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the cat namespace.
*/
public class ElasticsearchCatAsyncClient extends ApiClient {
public ElasticsearchCatAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchCatAsyncClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchCatAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchCatAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: cat.aliases
/**
* Shows information about currently configured aliases to indices including
* filter and routing infos.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture aliases(AliasesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AliasesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Shows information about currently configured aliases to indices including
* filter and routing infos.
*
* @param fn
* a function that initializes a builder to create the
* {@link AliasesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture aliases(
Function> fn) {
return aliases(fn.apply(new AliasesRequest.Builder()).build());
}
/**
* Shows information about currently configured aliases to indices including
* filter and routing infos.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture aliases() {
return this.transport.performRequestAsync(new AliasesRequest.Builder().build(), AliasesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.allocation
/**
* Provides a snapshot of how many shards are allocated to each data node and
* how much disk space they are using.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocation(AllocationRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AllocationRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides a snapshot of how many shards are allocated to each data node and
* how much disk space they are using.
*
* @param fn
* a function that initializes a builder to create the
* {@link AllocationRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture allocation(
Function> fn) {
return allocation(fn.apply(new AllocationRequest.Builder()).build());
}
/**
* Provides a snapshot of how many shards are allocated to each data node and
* how much disk space they are using.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture allocation() {
return this.transport.performRequestAsync(new AllocationRequest.Builder().build(), AllocationRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.count
/**
* Provides quick access to the document count of the entire cluster, or
* individual indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture count(CountRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CountRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides quick access to the document count of the entire cluster, or
* individual indices.
*
* @param fn
* a function that initializes a builder to create the
* {@link CountRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture count(
Function> fn) {
return count(fn.apply(new CountRequest.Builder()).build());
}
/**
* Provides quick access to the document count of the entire cluster, or
* individual indices.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture count() {
return this.transport.performRequestAsync(new CountRequest.Builder().build(), CountRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.fielddata
/**
* Shows how much heap memory is currently being used by fielddata on every data
* node in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture fielddata(FielddataRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FielddataRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Shows how much heap memory is currently being used by fielddata on every data
* node in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link FielddataRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture fielddata(
Function> fn) {
return fielddata(fn.apply(new FielddataRequest.Builder()).build());
}
/**
* Shows how much heap memory is currently being used by fielddata on every data
* node in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture fielddata() {
return this.transport.performRequestAsync(new FielddataRequest.Builder().build(), FielddataRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.health
/**
* Returns a concise representation of the cluster health.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health(HealthRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HealthRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns a concise representation of the cluster health.
*
* @param fn
* a function that initializes a builder to create the
* {@link HealthRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture health(
Function> fn) {
return health(fn.apply(new HealthRequest.Builder()).build());
}
/**
* Returns a concise representation of the cluster health.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture health() {
return this.transport.performRequestAsync(new HealthRequest.Builder().build(), HealthRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.help
/**
* Returns help for the Cat APIs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture help() {
return this.transport.performRequestAsync(HelpRequest._INSTANCE, HelpRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.indices
/**
* Returns information about indices: number of primaries and replicas, document
* counts, disk size, ...
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture indices(IndicesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) IndicesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about indices: number of primaries and replicas, document
* counts, disk size, ...
*
* @param fn
* a function that initializes a builder to create the
* {@link IndicesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture indices(
Function> fn) {
return indices(fn.apply(new IndicesRequest.Builder()).build());
}
/**
* Returns information about indices: number of primaries and replicas, document
* counts, disk size, ...
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture indices() {
return this.transport.performRequestAsync(new IndicesRequest.Builder().build(), IndicesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.master
/**
* Returns information about the master node.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture master() {
return this.transport.performRequestAsync(MasterRequest._INSTANCE, MasterRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.ml_data_frame_analytics
/**
* Gets configuration and usage information about data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDataFrameAnalytics(MlDataFrameAnalyticsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Gets configuration and usage information about data frame analytics jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlDataFrameAnalytics(
Function> fn) {
return mlDataFrameAnalytics(fn.apply(new MlDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Gets configuration and usage information about data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDataFrameAnalytics() {
return this.transport.performRequestAsync(new MlDataFrameAnalyticsRequest.Builder().build(),
MlDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.ml_datafeeds
/**
* Gets configuration and usage information about datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDatafeeds(MlDatafeedsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlDatafeedsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Gets configuration and usage information about datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlDatafeedsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlDatafeeds(
Function> fn) {
return mlDatafeeds(fn.apply(new MlDatafeedsRequest.Builder()).build());
}
/**
* Gets configuration and usage information about datafeeds.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlDatafeeds() {
return this.transport.performRequestAsync(new MlDatafeedsRequest.Builder().build(),
MlDatafeedsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.ml_jobs
/**
* Gets configuration and usage information about anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlJobs(MlJobsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlJobsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Gets configuration and usage information about anomaly detection jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlJobsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlJobs(
Function> fn) {
return mlJobs(fn.apply(new MlJobsRequest.Builder()).build());
}
/**
* Gets configuration and usage information about anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlJobs() {
return this.transport.performRequestAsync(new MlJobsRequest.Builder().build(), MlJobsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.ml_trained_models
/**
* Gets configuration and usage information about inference trained models.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlTrainedModels(MlTrainedModelsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) MlTrainedModelsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Gets configuration and usage information about inference trained models.
*
* @param fn
* a function that initializes a builder to create the
* {@link MlTrainedModelsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture mlTrainedModels(
Function> fn) {
return mlTrainedModels(fn.apply(new MlTrainedModelsRequest.Builder()).build());
}
/**
* Gets configuration and usage information about inference trained models.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture mlTrainedModels() {
return this.transport.performRequestAsync(new MlTrainedModelsRequest.Builder().build(),
MlTrainedModelsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cat.nodeattrs
/**
* Returns information about custom node attributes.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodeattrs() {
return this.transport.performRequestAsync(NodeattrsRequest._INSTANCE, NodeattrsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.nodes
/**
* Returns basic statistics about performance of cluster nodes.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodes(NodesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) NodesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns basic statistics about performance of cluster nodes.
*
* @param fn
* a function that initializes a builder to create the
* {@link NodesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture nodes(
Function> fn) {
return nodes(fn.apply(new NodesRequest.Builder()).build());
}
/**
* Returns basic statistics about performance of cluster nodes.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture nodes() {
return this.transport.performRequestAsync(new NodesRequest.Builder().build(), NodesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.pending_tasks
/**
* Returns a concise representation of the cluster pending tasks.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture pendingTasks() {
return this.transport.performRequestAsync(PendingTasksRequest._INSTANCE, PendingTasksRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.plugins
/**
* Returns information about installed plugins across nodes node.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture plugins() {
return this.transport.performRequestAsync(PluginsRequest._INSTANCE, PluginsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.recovery
/**
* Returns information about index shard recoveries, both on-going completed.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery(RecoveryRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RecoveryRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about index shard recoveries, both on-going completed.
*
* @param fn
* a function that initializes a builder to create the
* {@link RecoveryRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture recovery(
Function> fn) {
return recovery(fn.apply(new RecoveryRequest.Builder()).build());
}
/**
* Returns information about index shard recoveries, both on-going completed.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture recovery() {
return this.transport.performRequestAsync(new RecoveryRequest.Builder().build(), RecoveryRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.repositories
/**
* Returns information about snapshot repositories registered in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture repositories() {
return this.transport.performRequestAsync(RepositoriesRequest._INSTANCE, RepositoriesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.segments
/**
* Provides low-level information about the segments in the shards of an index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture segments(SegmentsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SegmentsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides low-level information about the segments in the shards of an index.
*
* @param fn
* a function that initializes a builder to create the
* {@link SegmentsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture segments(
Function> fn) {
return segments(fn.apply(new SegmentsRequest.Builder()).build());
}
/**
* Provides low-level information about the segments in the shards of an index.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture segments() {
return this.transport.performRequestAsync(new SegmentsRequest.Builder().build(), SegmentsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.shards
/**
* Provides a detailed view of shard allocation on nodes.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture shards(ShardsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ShardsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Provides a detailed view of shard allocation on nodes.
*
* @param fn
* a function that initializes a builder to create the
* {@link ShardsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture shards(
Function> fn) {
return shards(fn.apply(new ShardsRequest.Builder()).build());
}
/**
* Provides a detailed view of shard allocation on nodes.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture shards() {
return this.transport.performRequestAsync(new ShardsRequest.Builder().build(), ShardsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.snapshots
/**
* Returns all snapshots in a specific repository.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture snapshots(SnapshotsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SnapshotsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns all snapshots in a specific repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link SnapshotsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture snapshots(
Function> fn) {
return snapshots(fn.apply(new SnapshotsRequest.Builder()).build());
}
/**
* Returns all snapshots in a specific repository.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture snapshots() {
return this.transport.performRequestAsync(new SnapshotsRequest.Builder().build(), SnapshotsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.tasks
/**
* Returns information about the tasks currently executing on one or more nodes
* in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture tasks(TasksRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TasksRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about the tasks currently executing on one or more nodes
* in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link TasksRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture tasks(
Function> fn) {
return tasks(fn.apply(new TasksRequest.Builder()).build());
}
/**
* Returns information about the tasks currently executing on one or more nodes
* in the cluster.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture tasks() {
return this.transport.performRequestAsync(new TasksRequest.Builder().build(), TasksRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.templates
/**
* Returns information about existing templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture templates(TemplatesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TemplatesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns information about existing templates.
*
* @param fn
* a function that initializes a builder to create the
* {@link TemplatesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture templates(
Function> fn) {
return templates(fn.apply(new TemplatesRequest.Builder()).build());
}
/**
* Returns information about existing templates.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture templates() {
return this.transport.performRequestAsync(new TemplatesRequest.Builder().build(), TemplatesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.thread_pool
/**
* Returns cluster-wide thread pool statistics per node. By default the active,
* queue and rejected statistics are returned for all thread pools.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture threadPool(ThreadPoolRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ThreadPoolRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Returns cluster-wide thread pool statistics per node. By default the active,
* queue and rejected statistics are returned for all thread pools.
*
* @param fn
* a function that initializes a builder to create the
* {@link ThreadPoolRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture threadPool(
Function> fn) {
return threadPool(fn.apply(new ThreadPoolRequest.Builder()).build());
}
/**
* Returns cluster-wide thread pool statistics per node. By default the active,
* queue and rejected statistics are returned for all thread pools.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture threadPool() {
return this.transport.performRequestAsync(new ThreadPoolRequest.Builder().build(), ThreadPoolRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cat.transforms
/**
* Gets configuration and usage information about transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture transforms(TransformsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) TransformsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Gets configuration and usage information about transforms.
*
* @param fn
* a function that initializes a builder to create the
* {@link TransformsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture transforms(
Function> fn) {
return transforms(fn.apply(new TransformsRequest.Builder()).build());
}
/**
* Gets configuration and usage information about transforms.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture transforms() {
return this.transport.performRequestAsync(new TransformsRequest.Builder().build(), TransformsRequest._ENDPOINT,
this.transportOptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy