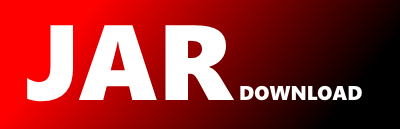
co.elastic.clients.elasticsearch.cluster.ElasticsearchClusterClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.cluster;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.BooleanResponse;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the cluster namespace.
*/
public class ElasticsearchClusterClient extends ApiClient {
public ElasticsearchClusterClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchClusterClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchClusterClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchClusterClient(this.transport, transportOptions);
}
// ----- Endpoint: cluster.allocation_explain
/**
* Provides explanations for shard allocations in the cluster.
*
* @see Documentation
* on elastic.co
*/
public AllocationExplainResponse allocationExplain(AllocationExplainRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AllocationExplainRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Provides explanations for shard allocations in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link AllocationExplainRequest}
* @see Documentation
* on elastic.co
*/
public final AllocationExplainResponse allocationExplain(
Function> fn)
throws IOException, ElasticsearchException {
return allocationExplain(fn.apply(new AllocationExplainRequest.Builder()).build());
}
/**
* Provides explanations for shard allocations in the cluster.
*
* @see Documentation
* on elastic.co
*/
public AllocationExplainResponse allocationExplain() throws IOException, ElasticsearchException {
return this.transport.performRequest(new AllocationExplainRequest.Builder().build(),
AllocationExplainRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.delete_component_template
/**
* Deletes a component template
*
* @see Documentation
* on elastic.co
*/
public DeleteComponentTemplateResponse deleteComponentTemplate(DeleteComponentTemplateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteComponentTemplateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes a component template
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteComponentTemplateResponse deleteComponentTemplate(
Function> fn)
throws IOException, ElasticsearchException {
return deleteComponentTemplate(fn.apply(new DeleteComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.delete_voting_config_exclusions
/**
* Clears cluster voting config exclusions.
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse deleteVotingConfigExclusions(DeleteVotingConfigExclusionsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteVotingConfigExclusionsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clears cluster voting config exclusions.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteVotingConfigExclusionsRequest}
* @see Documentation
* on elastic.co
*/
public final BooleanResponse deleteVotingConfigExclusions(
Function> fn)
throws IOException, ElasticsearchException {
return deleteVotingConfigExclusions(fn.apply(new DeleteVotingConfigExclusionsRequest.Builder()).build());
}
/**
* Clears cluster voting config exclusions.
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse deleteVotingConfigExclusions() throws IOException, ElasticsearchException {
return this.transport.performRequest(new DeleteVotingConfigExclusionsRequest.Builder().build(),
DeleteVotingConfigExclusionsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.exists_component_template
/**
* Returns information about whether a particular component template exist
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse existsComponentTemplate(ExistsComponentTemplateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExistsComponentTemplateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns information about whether a particular component template exist
*
* @param fn
* a function that initializes a builder to create the
* {@link ExistsComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final BooleanResponse existsComponentTemplate(
Function> fn)
throws IOException, ElasticsearchException {
return existsComponentTemplate(fn.apply(new ExistsComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.get_component_template
/**
* Returns one or more component templates
*
* @see Documentation
* on elastic.co
*/
public GetComponentTemplateResponse getComponentTemplate(GetComponentTemplateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetComponentTemplateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns one or more component templates
*
* @param fn
* a function that initializes a builder to create the
* {@link GetComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final GetComponentTemplateResponse getComponentTemplate(
Function> fn)
throws IOException, ElasticsearchException {
return getComponentTemplate(fn.apply(new GetComponentTemplateRequest.Builder()).build());
}
/**
* Returns one or more component templates
*
* @see Documentation
* on elastic.co
*/
public GetComponentTemplateResponse getComponentTemplate() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetComponentTemplateRequest.Builder().build(),
GetComponentTemplateRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.get_settings
/**
* Returns cluster settings.
*
* @see Documentation
* on elastic.co
*/
public GetClusterSettingsResponse getSettings(GetClusterSettingsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetClusterSettingsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns cluster settings.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetClusterSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final GetClusterSettingsResponse getSettings(
Function> fn)
throws IOException, ElasticsearchException {
return getSettings(fn.apply(new GetClusterSettingsRequest.Builder()).build());
}
/**
* Returns cluster settings.
*
* @see Documentation
* on elastic.co
*/
public GetClusterSettingsResponse getSettings() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetClusterSettingsRequest.Builder().build(),
GetClusterSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.health
/**
* Returns basic information about the health of the cluster.
*
* @see Documentation
* on elastic.co
*/
public HealthResponse health(HealthRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) HealthRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns basic information about the health of the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link HealthRequest}
* @see Documentation
* on elastic.co
*/
public final HealthResponse health(Function> fn)
throws IOException, ElasticsearchException {
return health(fn.apply(new HealthRequest.Builder()).build());
}
/**
* Returns basic information about the health of the cluster.
*
* @see Documentation
* on elastic.co
*/
public HealthResponse health() throws IOException, ElasticsearchException {
return this.transport.performRequest(new HealthRequest.Builder().build(), HealthRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.pending_tasks
/**
* Returns a list of any cluster-level changes (e.g. create index, update
* mapping, allocate or fail shard) which have not yet been executed.
*
* @see Documentation
* on elastic.co
*/
public PendingTasksResponse pendingTasks(PendingTasksRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PendingTasksRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns a list of any cluster-level changes (e.g. create index, update
* mapping, allocate or fail shard) which have not yet been executed.
*
* @param fn
* a function that initializes a builder to create the
* {@link PendingTasksRequest}
* @see Documentation
* on elastic.co
*/
public final PendingTasksResponse pendingTasks(
Function> fn)
throws IOException, ElasticsearchException {
return pendingTasks(fn.apply(new PendingTasksRequest.Builder()).build());
}
/**
* Returns a list of any cluster-level changes (e.g. create index, update
* mapping, allocate or fail shard) which have not yet been executed.
*
* @see Documentation
* on elastic.co
*/
public PendingTasksResponse pendingTasks() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PendingTasksRequest.Builder().build(), PendingTasksRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.post_voting_config_exclusions
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse postVotingConfigExclusions(PostVotingConfigExclusionsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PostVotingConfigExclusionsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostVotingConfigExclusionsRequest}
* @see Documentation
* on elastic.co
*/
public final BooleanResponse postVotingConfigExclusions(
Function> fn)
throws IOException, ElasticsearchException {
return postVotingConfigExclusions(fn.apply(new PostVotingConfigExclusionsRequest.Builder()).build());
}
/**
* Updates the cluster voting config exclusions by node ids or node names.
*
* @see Documentation
* on elastic.co
*/
public BooleanResponse postVotingConfigExclusions() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PostVotingConfigExclusionsRequest.Builder().build(),
PostVotingConfigExclusionsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.put_component_template
/**
* Creates or updates a component template
*
* @see Documentation
* on elastic.co
*/
public PutComponentTemplateResponse putComponentTemplate(PutComponentTemplateRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutComponentTemplateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates or updates a component template
*
* @param fn
* a function that initializes a builder to create the
* {@link PutComponentTemplateRequest}
* @see Documentation
* on elastic.co
*/
public final PutComponentTemplateResponse putComponentTemplate(
Function> fn)
throws IOException, ElasticsearchException {
return putComponentTemplate(fn.apply(new PutComponentTemplateRequest.Builder()).build());
}
// ----- Endpoint: cluster.put_settings
/**
* Updates the cluster settings.
*
* @see Documentation
* on elastic.co
*/
public PutClusterSettingsResponse putSettings(PutClusterSettingsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutClusterSettingsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates the cluster settings.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutClusterSettingsRequest}
* @see Documentation
* on elastic.co
*/
public final PutClusterSettingsResponse putSettings(
Function> fn)
throws IOException, ElasticsearchException {
return putSettings(fn.apply(new PutClusterSettingsRequest.Builder()).build());
}
/**
* Updates the cluster settings.
*
* @see Documentation
* on elastic.co
*/
public PutClusterSettingsResponse putSettings() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PutClusterSettingsRequest.Builder().build(),
PutClusterSettingsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: cluster.remote_info
/**
* Returns the information about configured remote clusters.
*
* @see Documentation
* on elastic.co
*/
public RemoteInfoResponse remoteInfo() throws IOException, ElasticsearchException {
return this.transport.performRequest(RemoteInfoRequest._INSTANCE, RemoteInfoRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.reroute
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @see Documentation
* on elastic.co
*/
public RerouteResponse reroute(RerouteRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RerouteRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link RerouteRequest}
* @see Documentation
* on elastic.co
*/
public final RerouteResponse reroute(Function> fn)
throws IOException, ElasticsearchException {
return reroute(fn.apply(new RerouteRequest.Builder()).build());
}
/**
* Allows to manually change the allocation of individual shards in the cluster.
*
* @see Documentation
* on elastic.co
*/
public RerouteResponse reroute() throws IOException, ElasticsearchException {
return this.transport.performRequest(new RerouteRequest.Builder().build(), RerouteRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.state
/**
* Returns a comprehensive information about the state of the cluster.
*
* @see Documentation
* on elastic.co
*/
public StateResponse state(StateRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns a comprehensive information about the state of the cluster.
*
* @param fn
* a function that initializes a builder to create the
* {@link StateRequest}
* @see Documentation
* on elastic.co
*/
public final StateResponse state(Function> fn)
throws IOException, ElasticsearchException {
return state(fn.apply(new StateRequest.Builder()).build());
}
/**
* Returns a comprehensive information about the state of the cluster.
*
* @see Documentation
* on elastic.co
*/
public StateResponse state() throws IOException, ElasticsearchException {
return this.transport.performRequest(new StateRequest.Builder().build(), StateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: cluster.stats
/**
* Returns high-level overview of cluster statistics.
*
* @see Documentation
* on elastic.co
*/
public ClusterStatsResponse stats(ClusterStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ClusterStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns high-level overview of cluster statistics.
*
* @param fn
* a function that initializes a builder to create the
* {@link ClusterStatsRequest}
* @see Documentation
* on elastic.co
*/
public final ClusterStatsResponse stats(
Function> fn)
throws IOException, ElasticsearchException {
return stats(fn.apply(new ClusterStatsRequest.Builder()).build());
}
/**
* Returns high-level overview of cluster statistics.
*
* @see Documentation
* on elastic.co
*/
public ClusterStatsResponse stats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ClusterStatsRequest.Builder().build(), ClusterStatsRequest._ENDPOINT,
this.transportOptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy