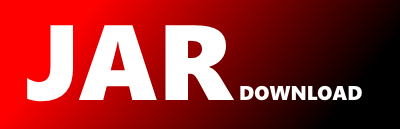
co.elastic.clients.elasticsearch.ml.ElasticsearchMlClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.ml;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.transport.endpoints.EndpointWithResponseMapperAttr;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the ml namespace.
*/
public class ElasticsearchMlClient extends ApiClient {
public ElasticsearchMlClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchMlClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchMlClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchMlClient(this.transport, transportOptions);
}
// ----- Endpoint: ml.close_job
/**
* Closes one or more anomaly detection jobs. A job can be opened and closed
* multiple times throughout its lifecycle.
*
* @see Documentation
* on elastic.co
*/
public CloseJobResponse closeJob(CloseJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloseJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Closes one or more anomaly detection jobs. A job can be opened and closed
* multiple times throughout its lifecycle.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloseJobRequest}
* @see Documentation
* on elastic.co
*/
public final CloseJobResponse closeJob(Function> fn)
throws IOException, ElasticsearchException {
return closeJob(fn.apply(new CloseJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar
/**
* Deletes a calendar.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarResponse deleteCalendar(DeleteCalendarRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarResponse deleteCalendar(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendar(fn.apply(new DeleteCalendarRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_event
/**
* Deletes scheduled events from a calendar.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarEventResponse deleteCalendarEvent(DeleteCalendarEventRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarEventRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes scheduled events from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarEventRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarEventResponse deleteCalendarEvent(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendarEvent(fn.apply(new DeleteCalendarEventRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_calendar_job
/**
* Deletes anomaly detection jobs from a calendar.
*
* @see Documentation
* on elastic.co
*/
public DeleteCalendarJobResponse deleteCalendarJob(DeleteCalendarJobRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteCalendarJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes anomaly detection jobs from a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteCalendarJobRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteCalendarJobResponse deleteCalendarJob(
Function> fn)
throws IOException, ElasticsearchException {
return deleteCalendarJob(fn.apply(new DeleteCalendarJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_data_frame_analytics
/**
* Deletes an existing data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public DeleteDataFrameAnalyticsResponse deleteDataFrameAnalytics(DeleteDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes an existing data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteDataFrameAnalyticsResponse deleteDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return deleteDataFrameAnalytics(fn.apply(new DeleteDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_datafeed
/**
* Deletes an existing datafeed.
*
* @see Documentation
* on elastic.co
*/
public DeleteDatafeedResponse deleteDatafeed(DeleteDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes an existing datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteDatafeedResponse deleteDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return deleteDatafeed(fn.apply(new DeleteDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_expired_data
/**
* Deletes expired and unused machine learning data.
*
* @see Documentation
* on elastic.co
*/
public DeleteExpiredDataResponse deleteExpiredData(DeleteExpiredDataRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteExpiredDataRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes expired and unused machine learning data.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteExpiredDataRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteExpiredDataResponse deleteExpiredData(
Function> fn)
throws IOException, ElasticsearchException {
return deleteExpiredData(fn.apply(new DeleteExpiredDataRequest.Builder()).build());
}
/**
* Deletes expired and unused machine learning data.
*
* @see Documentation
* on elastic.co
*/
public DeleteExpiredDataResponse deleteExpiredData() throws IOException, ElasticsearchException {
return this.transport.performRequest(new DeleteExpiredDataRequest.Builder().build(),
DeleteExpiredDataRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.delete_filter
/**
* Deletes a filter.
*
* @see Documentation
* on elastic.co
*/
public DeleteFilterResponse deleteFilter(DeleteFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes a filter.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteFilterRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteFilterResponse deleteFilter(
Function> fn)
throws IOException, ElasticsearchException {
return deleteFilter(fn.apply(new DeleteFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_forecast
/**
* Deletes forecasts from a machine learning job.
*
* @see Documentation
* on elastic.co
*/
public DeleteForecastResponse deleteForecast(DeleteForecastRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteForecastRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes forecasts from a machine learning job.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteForecastRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteForecastResponse deleteForecast(
Function> fn)
throws IOException, ElasticsearchException {
return deleteForecast(fn.apply(new DeleteForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_job
/**
* Deletes an existing anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public DeleteJobResponse deleteJob(DeleteJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes an existing anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteJobRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteJobResponse deleteJob(Function> fn)
throws IOException, ElasticsearchException {
return deleteJob(fn.apply(new DeleteJobRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_model_snapshot
/**
* Deletes an existing model snapshot.
*
* @see Documentation
* on elastic.co
*/
public DeleteModelSnapshotResponse deleteModelSnapshot(DeleteModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes an existing model snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteModelSnapshotResponse deleteModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return deleteModelSnapshot(fn.apply(new DeleteModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model
/**
* Deletes an existing trained inference model that is currently not referenced
* by an ingest pipeline.
*
* @see Documentation
* on elastic.co
*/
public DeleteTrainedModelResponse deleteTrainedModel(DeleteTrainedModelRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes an existing trained inference model that is currently not referenced
* by an ingest pipeline.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteTrainedModelResponse deleteTrainedModel(
Function> fn)
throws IOException, ElasticsearchException {
return deleteTrainedModel(fn.apply(new DeleteTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.delete_trained_model_alias
/**
* Deletes a model alias that refers to the trained model
*
* @see Documentation
* on elastic.co
*/
public DeleteTrainedModelAliasResponse deleteTrainedModelAlias(DeleteTrainedModelAliasRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteTrainedModelAliasRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes a model alias that refers to the trained model
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteTrainedModelAliasRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteTrainedModelAliasResponse deleteTrainedModelAlias(
Function> fn)
throws IOException, ElasticsearchException {
return deleteTrainedModelAlias(fn.apply(new DeleteTrainedModelAliasRequest.Builder()).build());
}
// ----- Endpoint: ml.estimate_model_memory
/**
* Estimates the model memory
*
* @see Documentation
* on elastic.co
*/
public EstimateModelMemoryResponse estimateModelMemory(EstimateModelMemoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EstimateModelMemoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Estimates the model memory
*
* @param fn
* a function that initializes a builder to create the
* {@link EstimateModelMemoryRequest}
* @see Documentation
* on elastic.co
*/
public final EstimateModelMemoryResponse estimateModelMemory(
Function> fn)
throws IOException, ElasticsearchException {
return estimateModelMemory(fn.apply(new EstimateModelMemoryRequest.Builder()).build());
}
/**
* Estimates the model memory
*
* @see Documentation
* on elastic.co
*/
public EstimateModelMemoryResponse estimateModelMemory() throws IOException, ElasticsearchException {
return this.transport.performRequest(new EstimateModelMemoryRequest.Builder().build(),
EstimateModelMemoryRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.evaluate_data_frame
/**
* Evaluates the data frame analytics for an annotated index.
*
* @see Documentation
* on elastic.co
*/
public EvaluateDataFrameResponse evaluateDataFrame(EvaluateDataFrameRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) EvaluateDataFrameRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Evaluates the data frame analytics for an annotated index.
*
* @param fn
* a function that initializes a builder to create the
* {@link EvaluateDataFrameRequest}
* @see Documentation
* on elastic.co
*/
public final EvaluateDataFrameResponse evaluateDataFrame(
Function> fn)
throws IOException, ElasticsearchException {
return evaluateDataFrame(fn.apply(new EvaluateDataFrameRequest.Builder()).build());
}
// ----- Endpoint: ml.explain_data_frame_analytics
/**
* Explains a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics(ExplainDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExplainDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Explains a data frame analytics config.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExplainDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return explainDataFrameAnalytics(fn.apply(new ExplainDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Explains a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public ExplainDataFrameAnalyticsResponse explainDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ExplainDataFrameAnalyticsRequest.Builder().build(),
ExplainDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.flush_job
/**
* Forces any buffered data to be processed by the job.
*
* @see Documentation
* on elastic.co
*/
public FlushJobResponse flushJob(FlushJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) FlushJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Forces any buffered data to be processed by the job.
*
* @param fn
* a function that initializes a builder to create the
* {@link FlushJobRequest}
* @see Documentation
* on elastic.co
*/
public final FlushJobResponse flushJob(Function> fn)
throws IOException, ElasticsearchException {
return flushJob(fn.apply(new FlushJobRequest.Builder()).build());
}
// ----- Endpoint: ml.forecast
/**
* Predicts the future behavior of a time series by using its historical
* behavior.
*
* @see Documentation
* on elastic.co
*/
public ForecastResponse forecast(ForecastRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ForecastRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Predicts the future behavior of a time series by using its historical
* behavior.
*
* @param fn
* a function that initializes a builder to create the
* {@link ForecastRequest}
* @see Documentation
* on elastic.co
*/
public final ForecastResponse forecast(Function> fn)
throws IOException, ElasticsearchException {
return forecast(fn.apply(new ForecastRequest.Builder()).build());
}
// ----- Endpoint: ml.get_buckets
/**
* Retrieves anomaly detection job results for one or more buckets.
*
* @see Documentation
* on elastic.co
*/
public GetBucketsResponse getBuckets(GetBucketsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetBucketsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves anomaly detection job results for one or more buckets.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final GetBucketsResponse getBuckets(Function> fn)
throws IOException, ElasticsearchException {
return getBuckets(fn.apply(new GetBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendar_events
/**
* Retrieves information about the scheduled events in calendars.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarEventsResponse getCalendarEvents(GetCalendarEventsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarEventsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves information about the scheduled events in calendars.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final GetCalendarEventsResponse getCalendarEvents(
Function> fn)
throws IOException, ElasticsearchException {
return getCalendarEvents(fn.apply(new GetCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_calendars
/**
* Retrieves configuration information for calendars.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarsResponse getCalendars(GetCalendarsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCalendarsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for calendars.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCalendarsRequest}
* @see Documentation
* on elastic.co
*/
public final GetCalendarsResponse getCalendars(
Function> fn)
throws IOException, ElasticsearchException {
return getCalendars(fn.apply(new GetCalendarsRequest.Builder()).build());
}
/**
* Retrieves configuration information for calendars.
*
* @see Documentation
* on elastic.co
*/
public GetCalendarsResponse getCalendars() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetCalendarsRequest.Builder().build(), GetCalendarsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_categories
/**
* Retrieves anomaly detection job results for one or more categories.
*
* @see Documentation
* on elastic.co
*/
public GetCategoriesResponse getCategories(GetCategoriesRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetCategoriesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves anomaly detection job results for one or more categories.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetCategoriesRequest}
* @see Documentation
* on elastic.co
*/
public final GetCategoriesResponse getCategories(
Function> fn)
throws IOException, ElasticsearchException {
return getCategories(fn.apply(new GetCategoriesRequest.Builder()).build());
}
// ----- Endpoint: ml.get_data_frame_analytics
/**
* Retrieves configuration information for data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsResponse getDataFrameAnalytics(GetDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for data frame analytics jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDataFrameAnalyticsResponse getDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return getDataFrameAnalytics(fn.apply(new GetDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Retrieves configuration information for data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsResponse getDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDataFrameAnalyticsRequest.Builder().build(),
GetDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_data_frame_analytics_stats
/**
* Retrieves usage information for data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats(GetDataFrameAnalyticsStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDataFrameAnalyticsStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves usage information for data frame analytics jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDataFrameAnalyticsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats(
Function> fn)
throws IOException, ElasticsearchException {
return getDataFrameAnalyticsStats(fn.apply(new GetDataFrameAnalyticsStatsRequest.Builder()).build());
}
/**
* Retrieves usage information for data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public GetDataFrameAnalyticsStatsResponse getDataFrameAnalyticsStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDataFrameAnalyticsStatsRequest.Builder().build(),
GetDataFrameAnalyticsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeed_stats
/**
* Retrieves usage information for datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedStatsResponse getDatafeedStats(GetDatafeedStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves usage information for datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDatafeedStatsResponse getDatafeedStats(
Function> fn)
throws IOException, ElasticsearchException {
return getDatafeedStats(fn.apply(new GetDatafeedStatsRequest.Builder()).build());
}
/**
* Retrieves usage information for datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedStatsResponse getDatafeedStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDatafeedStatsRequest.Builder().build(),
GetDatafeedStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_datafeeds
/**
* Retrieves configuration information for datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedsResponse getDatafeeds(GetDatafeedsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetDatafeedsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetDatafeedsRequest}
* @see Documentation
* on elastic.co
*/
public final GetDatafeedsResponse getDatafeeds(
Function> fn)
throws IOException, ElasticsearchException {
return getDatafeeds(fn.apply(new GetDatafeedsRequest.Builder()).build());
}
/**
* Retrieves configuration information for datafeeds.
*
* @see Documentation
* on elastic.co
*/
public GetDatafeedsResponse getDatafeeds() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetDatafeedsRequest.Builder().build(), GetDatafeedsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_filters
/**
* Retrieves filters.
*
* @see Documentation
* on elastic.co
*/
public GetFiltersResponse getFilters(GetFiltersRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetFiltersRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves filters.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetFiltersRequest}
* @see Documentation
* on elastic.co
*/
public final GetFiltersResponse getFilters(Function> fn)
throws IOException, ElasticsearchException {
return getFilters(fn.apply(new GetFiltersRequest.Builder()).build());
}
/**
* Retrieves filters.
*
* @see Documentation
* on elastic.co
*/
public GetFiltersResponse getFilters() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetFiltersRequest.Builder().build(), GetFiltersRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_influencers
/**
* Retrieves anomaly detection job results for one or more influencers.
*
* @see Documentation
* on elastic.co
*/
public GetInfluencersResponse getInfluencers(GetInfluencersRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetInfluencersRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves anomaly detection job results for one or more influencers.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetInfluencersRequest}
* @see Documentation
* on elastic.co
*/
public final GetInfluencersResponse getInfluencers(
Function> fn)
throws IOException, ElasticsearchException {
return getInfluencers(fn.apply(new GetInfluencersRequest.Builder()).build());
}
// ----- Endpoint: ml.get_job_stats
/**
* Retrieves usage information for anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public GetJobStatsResponse getJobStats(GetJobStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves usage information for anomaly detection jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetJobStatsResponse getJobStats(
Function> fn)
throws IOException, ElasticsearchException {
return getJobStats(fn.apply(new GetJobStatsRequest.Builder()).build());
}
/**
* Retrieves usage information for anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public GetJobStatsResponse getJobStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetJobStatsRequest.Builder().build(), GetJobStatsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_jobs
/**
* Retrieves configuration information for anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public GetJobsResponse getJobs(GetJobsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetJobsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for anomaly detection jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetJobsRequest}
* @see Documentation
* on elastic.co
*/
public final GetJobsResponse getJobs(Function> fn)
throws IOException, ElasticsearchException {
return getJobs(fn.apply(new GetJobsRequest.Builder()).build());
}
/**
* Retrieves configuration information for anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public GetJobsResponse getJobs() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetJobsRequest.Builder().build(), GetJobsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.get_memory_stats
/**
* Returns information on how ML is using memory.
*
* @see Documentation
* on elastic.co
*/
public GetMemoryStatsResponse getMemoryStats(GetMemoryStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetMemoryStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns information on how ML is using memory.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetMemoryStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetMemoryStatsResponse getMemoryStats(
Function> fn)
throws IOException, ElasticsearchException {
return getMemoryStats(fn.apply(new GetMemoryStatsRequest.Builder()).build());
}
/**
* Returns information on how ML is using memory.
*
* @see Documentation
* on elastic.co
*/
public GetMemoryStatsResponse getMemoryStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetMemoryStatsRequest.Builder().build(),
GetMemoryStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_model_snapshots
/**
* Retrieves information about model snapshots.
*
* @see Documentation
* on elastic.co
*/
public GetModelSnapshotsResponse getModelSnapshots(GetModelSnapshotsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetModelSnapshotsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves information about model snapshots.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetModelSnapshotsRequest}
* @see Documentation
* on elastic.co
*/
public final GetModelSnapshotsResponse getModelSnapshots(
Function> fn)
throws IOException, ElasticsearchException {
return getModelSnapshots(fn.apply(new GetModelSnapshotsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_overall_buckets
/**
* Retrieves overall bucket results that summarize the bucket results of
* multiple anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public GetOverallBucketsResponse getOverallBuckets(GetOverallBucketsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetOverallBucketsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves overall bucket results that summarize the bucket results of
* multiple anomaly detection jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetOverallBucketsRequest}
* @see Documentation
* on elastic.co
*/
public final GetOverallBucketsResponse getOverallBuckets(
Function> fn)
throws IOException, ElasticsearchException {
return getOverallBuckets(fn.apply(new GetOverallBucketsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_records
/**
* Retrieves anomaly records for an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public GetRecordsResponse getRecords(GetRecordsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRecordsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves anomaly records for an anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRecordsRequest}
* @see Documentation
* on elastic.co
*/
public final GetRecordsResponse getRecords(Function> fn)
throws IOException, ElasticsearchException {
return getRecords(fn.apply(new GetRecordsRequest.Builder()).build());
}
// ----- Endpoint: ml.get_trained_models
/**
* Retrieves configuration information for a trained inference model.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsResponse getTrainedModels(GetTrainedModelsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves configuration information for a trained inference model.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsRequest}
* @see Documentation
* on elastic.co
*/
public final GetTrainedModelsResponse getTrainedModels(
Function> fn)
throws IOException, ElasticsearchException {
return getTrainedModels(fn.apply(new GetTrainedModelsRequest.Builder()).build());
}
/**
* Retrieves configuration information for a trained inference model.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsResponse getTrainedModels() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetTrainedModelsRequest.Builder().build(),
GetTrainedModelsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.get_trained_models_stats
/**
* Retrieves usage information for trained inference models.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsStatsResponse getTrainedModelsStats(GetTrainedModelsStatsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetTrainedModelsStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves usage information for trained inference models.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetTrainedModelsStatsRequest}
* @see Documentation
* on elastic.co
*/
public final GetTrainedModelsStatsResponse getTrainedModelsStats(
Function> fn)
throws IOException, ElasticsearchException {
return getTrainedModelsStats(fn.apply(new GetTrainedModelsStatsRequest.Builder()).build());
}
/**
* Retrieves usage information for trained inference models.
*
* @see Documentation
* on elastic.co
*/
public GetTrainedModelsStatsResponse getTrainedModelsStats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetTrainedModelsStatsRequest.Builder().build(),
GetTrainedModelsStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.infer_trained_model_deployment
/**
* Evaluate a trained model.
*
* @see Documentation
* on elastic.co
*/
public InferTrainedModelDeploymentResponse inferTrainedModelDeployment(InferTrainedModelDeploymentRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) InferTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Evaluate a trained model.
*
* @param fn
* a function that initializes a builder to create the
* {@link InferTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final InferTrainedModelDeploymentResponse inferTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return inferTrainedModelDeployment(fn.apply(new InferTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.info
/**
* Returns defaults and limits used by machine learning.
*
* @see Documentation
* on elastic.co
*/
public MlInfoResponse info() throws IOException, ElasticsearchException {
return this.transport.performRequest(MlInfoRequest._INSTANCE, MlInfoRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.open_job
/**
* Opens one or more anomaly detection jobs.
*
* @see Documentation
* on elastic.co
*/
public OpenJobResponse openJob(OpenJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) OpenJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Opens one or more anomaly detection jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link OpenJobRequest}
* @see Documentation
* on elastic.co
*/
public final OpenJobResponse openJob(Function> fn)
throws IOException, ElasticsearchException {
return openJob(fn.apply(new OpenJobRequest.Builder()).build());
}
// ----- Endpoint: ml.post_calendar_events
/**
* Posts scheduled events in a calendar.
*
* @see Documentation
* on elastic.co
*/
public PostCalendarEventsResponse postCalendarEvents(PostCalendarEventsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PostCalendarEventsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Posts scheduled events in a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostCalendarEventsRequest}
* @see Documentation
* on elastic.co
*/
public final PostCalendarEventsResponse postCalendarEvents(
Function> fn)
throws IOException, ElasticsearchException {
return postCalendarEvents(fn.apply(new PostCalendarEventsRequest.Builder()).build());
}
// ----- Endpoint: ml.post_data
/**
* Sends data to an anomaly detection job for analysis.
*
* @see Documentation
* on elastic.co
*/
public PostDataResponse postData(PostDataRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint, PostDataResponse, ErrorResponse> endpoint = (JsonEndpoint, PostDataResponse, ErrorResponse>) PostDataRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Sends data to an anomaly detection job for analysis.
*
* @param fn
* a function that initializes a builder to create the
* {@link PostDataRequest}
* @see Documentation
* on elastic.co
*/
public final PostDataResponse postData(
Function, ObjectBuilder>> fn)
throws IOException, ElasticsearchException {
return postData(fn.apply(new PostDataRequest.Builder()).build());
}
// ----- Endpoint: ml.preview_data_frame_analytics
/**
* Previews that will be analyzed given a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics(PreviewDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PreviewDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Previews that will be analyzed given a data frame analytics config.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return previewDataFrameAnalytics(fn.apply(new PreviewDataFrameAnalyticsRequest.Builder()).build());
}
/**
* Previews that will be analyzed given a data frame analytics config.
*
* @see Documentation
* on elastic.co
*/
public PreviewDataFrameAnalyticsResponse previewDataFrameAnalytics() throws IOException, ElasticsearchException {
return this.transport.performRequest(new PreviewDataFrameAnalyticsRequest.Builder().build(),
PreviewDataFrameAnalyticsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.preview_datafeed
/**
* Previews a datafeed.
*
* @see Documentation
* on elastic.co
*/
public PreviewDatafeedResponse previewDatafeed(PreviewDatafeedRequest request,
Class tDocumentClass) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint, ErrorResponse> endpoint = (JsonEndpoint, ErrorResponse>) PreviewDatafeedRequest._ENDPOINT;
endpoint = new EndpointWithResponseMapperAttr<>(endpoint,
"co.elastic.clients:Deserializer:ml.preview_datafeed.TDocument", getDeserializer(tDocumentClass));
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Previews a datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link PreviewDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final PreviewDatafeedResponse previewDatafeed(
Function> fn,
Class tDocumentClass) throws IOException, ElasticsearchException {
return previewDatafeed(fn.apply(new PreviewDatafeedRequest.Builder()).build(), tDocumentClass);
}
// ----- Endpoint: ml.put_calendar
/**
* Instantiates a calendar.
*
* @see Documentation
* on elastic.co
*/
public PutCalendarResponse putCalendar(PutCalendarRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutCalendarRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Instantiates a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutCalendarRequest}
* @see Documentation
* on elastic.co
*/
public final PutCalendarResponse putCalendar(
Function> fn)
throws IOException, ElasticsearchException {
return putCalendar(fn.apply(new PutCalendarRequest.Builder()).build());
}
// ----- Endpoint: ml.put_calendar_job
/**
* Adds an anomaly detection job to a calendar.
*
* @see Documentation
* on elastic.co
*/
public PutCalendarJobResponse putCalendarJob(PutCalendarJobRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutCalendarJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Adds an anomaly detection job to a calendar.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutCalendarJobRequest}
* @see Documentation
* on elastic.co
*/
public final PutCalendarJobResponse putCalendarJob(
Function> fn)
throws IOException, ElasticsearchException {
return putCalendarJob(fn.apply(new PutCalendarJobRequest.Builder()).build());
}
// ----- Endpoint: ml.put_data_frame_analytics
/**
* Instantiates a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public PutDataFrameAnalyticsResponse putDataFrameAnalytics(PutDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Instantiates a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final PutDataFrameAnalyticsResponse putDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return putDataFrameAnalytics(fn.apply(new PutDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.put_datafeed
/**
* Instantiates a datafeed.
*
* @see Documentation
* on elastic.co
*/
public PutDatafeedResponse putDatafeed(PutDatafeedRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Instantiates a datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final PutDatafeedResponse putDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return putDatafeed(fn.apply(new PutDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.put_filter
/**
* Instantiates a filter.
*
* @see Documentation
* on elastic.co
*/
public PutFilterResponse putFilter(PutFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Instantiates a filter.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutFilterRequest}
* @see Documentation
* on elastic.co
*/
public final PutFilterResponse putFilter(Function> fn)
throws IOException, ElasticsearchException {
return putFilter(fn.apply(new PutFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.put_job
/**
* Instantiates an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public PutJobResponse putJob(PutJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Instantiates an anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutJobRequest}
* @see Documentation
* on elastic.co
*/
public final PutJobResponse putJob(Function> fn)
throws IOException, ElasticsearchException {
return putJob(fn.apply(new PutJobRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model
/**
* Creates an inference trained model.
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelResponse putTrainedModel(PutTrainedModelRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates an inference trained model.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelResponse putTrainedModel(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModel(fn.apply(new PutTrainedModelRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_alias
/**
* Creates a new model alias (or reassigns an existing one) to refer to the
* trained model
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelAliasResponse putTrainedModelAlias(PutTrainedModelAliasRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelAliasRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a new model alias (or reassigns an existing one) to refer to the
* trained model
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelAliasRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelAliasResponse putTrainedModelAlias(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelAlias(fn.apply(new PutTrainedModelAliasRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_definition_part
/**
* Creates part of a trained model definition
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelDefinitionPartResponse putTrainedModelDefinitionPart(
PutTrainedModelDefinitionPartRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelDefinitionPartRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates part of a trained model definition
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelDefinitionPartRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelDefinitionPartResponse putTrainedModelDefinitionPart(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelDefinitionPart(fn.apply(new PutTrainedModelDefinitionPartRequest.Builder()).build());
}
// ----- Endpoint: ml.put_trained_model_vocabulary
/**
* Creates a trained model vocabulary
*
* @see Documentation
* on elastic.co
*/
public PutTrainedModelVocabularyResponse putTrainedModelVocabulary(PutTrainedModelVocabularyRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutTrainedModelVocabularyRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a trained model vocabulary
*
* @param fn
* a function that initializes a builder to create the
* {@link PutTrainedModelVocabularyRequest}
* @see Documentation
* on elastic.co
*/
public final PutTrainedModelVocabularyResponse putTrainedModelVocabulary(
Function> fn)
throws IOException, ElasticsearchException {
return putTrainedModelVocabulary(fn.apply(new PutTrainedModelVocabularyRequest.Builder()).build());
}
// ----- Endpoint: ml.reset_job
/**
* Resets an existing anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public ResetJobResponse resetJob(ResetJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ResetJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Resets an existing anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link ResetJobRequest}
* @see Documentation
* on elastic.co
*/
public final ResetJobResponse resetJob(Function> fn)
throws IOException, ElasticsearchException {
return resetJob(fn.apply(new ResetJobRequest.Builder()).build());
}
// ----- Endpoint: ml.revert_model_snapshot
/**
* Reverts to a specific snapshot.
*
* @see Documentation
* on elastic.co
*/
public RevertModelSnapshotResponse revertModelSnapshot(RevertModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RevertModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Reverts to a specific snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link RevertModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final RevertModelSnapshotResponse revertModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return revertModelSnapshot(fn.apply(new RevertModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.set_upgrade_mode
/**
* Sets a cluster wide upgrade_mode setting that prepares machine learning
* indices for an upgrade.
*
* @see Documentation
* on elastic.co
*/
public SetUpgradeModeResponse setUpgradeMode(SetUpgradeModeRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SetUpgradeModeRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Sets a cluster wide upgrade_mode setting that prepares machine learning
* indices for an upgrade.
*
* @param fn
* a function that initializes a builder to create the
* {@link SetUpgradeModeRequest}
* @see Documentation
* on elastic.co
*/
public final SetUpgradeModeResponse setUpgradeMode(
Function> fn)
throws IOException, ElasticsearchException {
return setUpgradeMode(fn.apply(new SetUpgradeModeRequest.Builder()).build());
}
/**
* Sets a cluster wide upgrade_mode setting that prepares machine learning
* indices for an upgrade.
*
* @see Documentation
* on elastic.co
*/
public SetUpgradeModeResponse setUpgradeMode() throws IOException, ElasticsearchException {
return this.transport.performRequest(new SetUpgradeModeRequest.Builder().build(),
SetUpgradeModeRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: ml.start_data_frame_analytics
/**
* Starts a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public StartDataFrameAnalyticsResponse startDataFrameAnalytics(StartDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Starts a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final StartDataFrameAnalyticsResponse startDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return startDataFrameAnalytics(fn.apply(new StartDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.start_datafeed
/**
* Starts one or more datafeeds.
*
* @see Documentation
* on elastic.co
*/
public StartDatafeedResponse startDatafeed(StartDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Starts one or more datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final StartDatafeedResponse startDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return startDatafeed(fn.apply(new StartDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.start_trained_model_deployment
/**
* Start a trained model deployment.
*
* @see Documentation
* on elastic.co
*/
public StartTrainedModelDeploymentResponse startTrainedModelDeployment(StartTrainedModelDeploymentRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StartTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Start a trained model deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link StartTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final StartTrainedModelDeploymentResponse startTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return startTrainedModelDeployment(fn.apply(new StartTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_data_frame_analytics
/**
* Stops one or more data frame analytics jobs.
*
* @see Documentation
* on elastic.co
*/
public StopDataFrameAnalyticsResponse stopDataFrameAnalytics(StopDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stops one or more data frame analytics jobs.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final StopDataFrameAnalyticsResponse stopDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return stopDataFrameAnalytics(fn.apply(new StopDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_datafeed
/**
* Stops one or more datafeeds.
*
* @see Documentation
* on elastic.co
*/
public StopDatafeedResponse stopDatafeed(StopDatafeedRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stops one or more datafeeds.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final StopDatafeedResponse stopDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return stopDatafeed(fn.apply(new StopDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.stop_trained_model_deployment
/**
* Stop a trained model deployment.
*
* @see Documentation
* on elastic.co
*/
public StopTrainedModelDeploymentResponse stopTrainedModelDeployment(StopTrainedModelDeploymentRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) StopTrainedModelDeploymentRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Stop a trained model deployment.
*
* @param fn
* a function that initializes a builder to create the
* {@link StopTrainedModelDeploymentRequest}
* @see Documentation
* on elastic.co
*/
public final StopTrainedModelDeploymentResponse stopTrainedModelDeployment(
Function> fn)
throws IOException, ElasticsearchException {
return stopTrainedModelDeployment(fn.apply(new StopTrainedModelDeploymentRequest.Builder()).build());
}
// ----- Endpoint: ml.update_data_frame_analytics
/**
* Updates certain properties of a data frame analytics job.
*
* @see Documentation
* on elastic.co
*/
public UpdateDataFrameAnalyticsResponse updateDataFrameAnalytics(UpdateDataFrameAnalyticsRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateDataFrameAnalyticsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates certain properties of a data frame analytics job.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateDataFrameAnalyticsRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateDataFrameAnalyticsResponse updateDataFrameAnalytics(
Function> fn)
throws IOException, ElasticsearchException {
return updateDataFrameAnalytics(fn.apply(new UpdateDataFrameAnalyticsRequest.Builder()).build());
}
// ----- Endpoint: ml.update_datafeed
/**
* Updates certain properties of a datafeed.
*
* @see Documentation
* on elastic.co
*/
public UpdateDatafeedResponse updateDatafeed(UpdateDatafeedRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateDatafeedRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates certain properties of a datafeed.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateDatafeedRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateDatafeedResponse updateDatafeed(
Function> fn)
throws IOException, ElasticsearchException {
return updateDatafeed(fn.apply(new UpdateDatafeedRequest.Builder()).build());
}
// ----- Endpoint: ml.update_filter
/**
* Updates the description of a filter, adds items, or removes items.
*
* @see Documentation
* on elastic.co
*/
public UpdateFilterResponse updateFilter(UpdateFilterRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateFilterRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates the description of a filter, adds items, or removes items.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateFilterRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateFilterResponse updateFilter(
Function> fn)
throws IOException, ElasticsearchException {
return updateFilter(fn.apply(new UpdateFilterRequest.Builder()).build());
}
// ----- Endpoint: ml.update_job
/**
* Updates certain properties of an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public UpdateJobResponse updateJob(UpdateJobRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateJobRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates certain properties of an anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateJobRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateJobResponse updateJob(Function> fn)
throws IOException, ElasticsearchException {
return updateJob(fn.apply(new UpdateJobRequest.Builder()).build());
}
// ----- Endpoint: ml.update_model_snapshot
/**
* Updates certain properties of a snapshot.
*
* @see Documentation
* on elastic.co
*/
public UpdateModelSnapshotResponse updateModelSnapshot(UpdateModelSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpdateModelSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Updates certain properties of a snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpdateModelSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final UpdateModelSnapshotResponse updateModelSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return updateModelSnapshot(fn.apply(new UpdateModelSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.upgrade_job_snapshot
/**
* Upgrades a given job snapshot to the current major version.
*
* @see Documentation
* on elastic.co
*/
public UpgradeJobSnapshotResponse upgradeJobSnapshot(UpgradeJobSnapshotRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) UpgradeJobSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Upgrades a given job snapshot to the current major version.
*
* @param fn
* a function that initializes a builder to create the
* {@link UpgradeJobSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final UpgradeJobSnapshotResponse upgradeJobSnapshot(
Function> fn)
throws IOException, ElasticsearchException {
return upgradeJobSnapshot(fn.apply(new UpgradeJobSnapshotRequest.Builder()).build());
}
// ----- Endpoint: ml.validate
/**
* Validates an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public ValidateResponse validate(ValidateRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ValidateRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Validates an anomaly detection job.
*
* @param fn
* a function that initializes a builder to create the
* {@link ValidateRequest}
* @see Documentation
* on elastic.co
*/
public final ValidateResponse validate(Function> fn)
throws IOException, ElasticsearchException {
return validate(fn.apply(new ValidateRequest.Builder()).build());
}
/**
* Validates an anomaly detection job.
*
* @see Documentation
* on elastic.co
*/
public ValidateResponse validate() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ValidateRequest.Builder().build(), ValidateRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: ml.validate_detector
/**
* Validates an anomaly detection detector.
*
* @see Documentation
* on elastic.co
*/
public ValidateDetectorResponse validateDetector(ValidateDetectorRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ValidateDetectorRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Validates an anomaly detection detector.
*
* @param fn
* a function that initializes a builder to create the
* {@link ValidateDetectorRequest}
* @see Documentation
* on elastic.co
*/
public final ValidateDetectorResponse validateDetector(
Function> fn)
throws IOException, ElasticsearchException {
return validateDetector(fn.apply(new ValidateDetectorRequest.Builder()).build());
}
/**
* Validates an anomaly detection detector.
*
* @see Documentation
* on elastic.co
*/
public ValidateDetectorResponse validateDetector() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ValidateDetectorRequest.Builder().build(),
ValidateDetectorRequest._ENDPOINT, this.transportOptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy