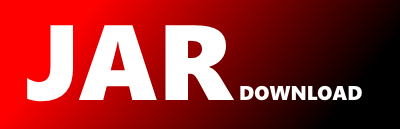
co.elastic.clients.elasticsearch.ml.InferTrainedModelDeploymentResponse Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.ml;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import co.elastic.clients.util.WithJsonObjectBuilderBase;
import jakarta.json.stream.JsonGenerator;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
import javax.annotation.Nullable;
// typedef: ml.infer_trained_model_deployment.Response
/**
*
* @see API
* specification
*/
@JsonpDeserializable
public class InferTrainedModelDeploymentResponse implements JsonpSerializable {
private final List entities;
@Nullable
private final Boolean isTruncated;
private final List predictedValue;
@Nullable
private final String predictedValueSequence;
@Nullable
private final Double predictionProbability;
private final List topClasses;
@Nullable
private final String warning;
// ---------------------------------------------------------------------------------------------
private InferTrainedModelDeploymentResponse(Builder builder) {
this.entities = ApiTypeHelper.unmodifiable(builder.entities);
this.isTruncated = builder.isTruncated;
this.predictedValue = ApiTypeHelper.unmodifiable(builder.predictedValue);
this.predictedValueSequence = builder.predictedValueSequence;
this.predictionProbability = builder.predictionProbability;
this.topClasses = ApiTypeHelper.unmodifiableRequired(builder.topClasses, this, "topClasses");
this.warning = builder.warning;
}
public static InferTrainedModelDeploymentResponse of(
Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* If the model is trained for named entity recognition (NER) tasks, the
* response contains the recognized entities.
*
* API name: {@code entities}
*/
public final List entities() {
return this.entities;
}
/**
* Indicates whether the input text was truncated to meet the model's maximum
* sequence length limit. This property is present only when it is true.
*
* API name: {@code is_truncated}
*/
@Nullable
public final Boolean isTruncated() {
return this.isTruncated;
}
/**
* If the model is trained for a text classification or zero shot classification
* task, the response is the predicted class. For named entity recognition (NER)
* tasks, it contains the annotated text output. For fill mask tasks, it
* contains the top prediction for replacing the mask token. For text embedding
* tasks, it contains the raw numerical text embedding values.
*
* API name: {@code predicted_value}
*/
public final List predictedValue() {
return this.predictedValue;
}
/**
* For fill mask tasks, the response contains the input text sequence with the
* mask token replaced by the predicted value.
*
* API name: {@code predicted_value_sequence}
*/
@Nullable
public final String predictedValueSequence() {
return this.predictedValueSequence;
}
/**
* Specifies a confidence score for the predicted value.
*
* API name: {@code prediction_probability}
*/
@Nullable
public final Double predictionProbability() {
return this.predictionProbability;
}
/**
* Required - For fill mask, text classification, and zero shot classification
* tasks, the response contains a list of top class entries.
*
* API name: {@code top_classes}
*/
public final List topClasses() {
return this.topClasses;
}
/**
* If the request failed, the response contains the reason for the failure.
*
* API name: {@code warning}
*/
@Nullable
public final String warning() {
return this.warning;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (ApiTypeHelper.isDefined(this.entities)) {
generator.writeKey("entities");
generator.writeStartArray();
for (TrainedModelEntities item0 : this.entities) {
item0.serialize(generator, mapper);
}
generator.writeEnd();
}
if (this.isTruncated != null) {
generator.writeKey("is_truncated");
generator.write(this.isTruncated);
}
if (ApiTypeHelper.isDefined(this.predictedValue)) {
generator.writeKey("predicted_value");
generator.writeStartArray();
for (String item0 : this.predictedValue) {
generator.write(item0);
}
generator.writeEnd();
}
if (this.predictedValueSequence != null) {
generator.writeKey("predicted_value_sequence");
generator.write(this.predictedValueSequence);
}
if (this.predictionProbability != null) {
generator.writeKey("prediction_probability");
generator.write(this.predictionProbability);
}
if (ApiTypeHelper.isDefined(this.topClasses)) {
generator.writeKey("top_classes");
generator.writeStartArray();
for (TopClassEntry item0 : this.topClasses) {
item0.serialize(generator, mapper);
}
generator.writeEnd();
}
if (this.warning != null) {
generator.writeKey("warning");
generator.write(this.warning);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link InferTrainedModelDeploymentResponse}.
*/
public static class Builder extends WithJsonObjectBuilderBase
implements
ObjectBuilder {
@Nullable
private List entities;
@Nullable
private Boolean isTruncated;
@Nullable
private List predictedValue;
@Nullable
private String predictedValueSequence;
@Nullable
private Double predictionProbability;
private List topClasses;
@Nullable
private String warning;
/**
* If the model is trained for named entity recognition (NER) tasks, the
* response contains the recognized entities.
*
* API name: {@code entities}
*
* Adds all elements of list
to entities
.
*/
public final Builder entities(List list) {
this.entities = _listAddAll(this.entities, list);
return this;
}
/**
* If the model is trained for named entity recognition (NER) tasks, the
* response contains the recognized entities.
*
* API name: {@code entities}
*
* Adds one or more values to entities
.
*/
public final Builder entities(TrainedModelEntities value, TrainedModelEntities... values) {
this.entities = _listAdd(this.entities, value, values);
return this;
}
/**
* If the model is trained for named entity recognition (NER) tasks, the
* response contains the recognized entities.
*
* API name: {@code entities}
*
* Adds a value to entities
using a builder lambda.
*/
public final Builder entities(Function> fn) {
return entities(fn.apply(new TrainedModelEntities.Builder()).build());
}
/**
* Indicates whether the input text was truncated to meet the model's maximum
* sequence length limit. This property is present only when it is true.
*
* API name: {@code is_truncated}
*/
public final Builder isTruncated(@Nullable Boolean value) {
this.isTruncated = value;
return this;
}
/**
* If the model is trained for a text classification or zero shot classification
* task, the response is the predicted class. For named entity recognition (NER)
* tasks, it contains the annotated text output. For fill mask tasks, it
* contains the top prediction for replacing the mask token. For text embedding
* tasks, it contains the raw numerical text embedding values.
*
* API name: {@code predicted_value}
*
* Adds all elements of list
to predictedValue
.
*/
public final Builder predictedValue(List list) {
this.predictedValue = _listAddAll(this.predictedValue, list);
return this;
}
/**
* If the model is trained for a text classification or zero shot classification
* task, the response is the predicted class. For named entity recognition (NER)
* tasks, it contains the annotated text output. For fill mask tasks, it
* contains the top prediction for replacing the mask token. For text embedding
* tasks, it contains the raw numerical text embedding values.
*
* API name: {@code predicted_value}
*
* Adds one or more values to predictedValue
.
*/
public final Builder predictedValue(String value, String... values) {
this.predictedValue = _listAdd(this.predictedValue, value, values);
return this;
}
/**
* For fill mask tasks, the response contains the input text sequence with the
* mask token replaced by the predicted value.
*
* API name: {@code predicted_value_sequence}
*/
public final Builder predictedValueSequence(@Nullable String value) {
this.predictedValueSequence = value;
return this;
}
/**
* Specifies a confidence score for the predicted value.
*
* API name: {@code prediction_probability}
*/
public final Builder predictionProbability(@Nullable Double value) {
this.predictionProbability = value;
return this;
}
/**
* Required - For fill mask, text classification, and zero shot classification
* tasks, the response contains a list of top class entries.
*
* API name: {@code top_classes}
*
* Adds all elements of list
to topClasses
.
*/
public final Builder topClasses(List list) {
this.topClasses = _listAddAll(this.topClasses, list);
return this;
}
/**
* Required - For fill mask, text classification, and zero shot classification
* tasks, the response contains a list of top class entries.
*
* API name: {@code top_classes}
*
* Adds one or more values to topClasses
.
*/
public final Builder topClasses(TopClassEntry value, TopClassEntry... values) {
this.topClasses = _listAdd(this.topClasses, value, values);
return this;
}
/**
* Required - For fill mask, text classification, and zero shot classification
* tasks, the response contains a list of top class entries.
*
* API name: {@code top_classes}
*
* Adds a value to topClasses
using a builder lambda.
*/
public final Builder topClasses(Function> fn) {
return topClasses(fn.apply(new TopClassEntry.Builder()).build());
}
/**
* If the request failed, the response contains the reason for the failure.
*
* API name: {@code warning}
*/
public final Builder warning(@Nullable String value) {
this.warning = value;
return this;
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link InferTrainedModelDeploymentResponse}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public InferTrainedModelDeploymentResponse build() {
_checkSingleUse();
return new InferTrainedModelDeploymentResponse(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link InferTrainedModelDeploymentResponse}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer
.lazy(Builder::new,
InferTrainedModelDeploymentResponse::setupInferTrainedModelDeploymentResponseDeserializer);
protected static void setupInferTrainedModelDeploymentResponseDeserializer(
ObjectDeserializer op) {
op.add(Builder::entities, JsonpDeserializer.arrayDeserializer(TrainedModelEntities._DESERIALIZER), "entities");
op.add(Builder::isTruncated, JsonpDeserializer.booleanDeserializer(), "is_truncated");
op.add(Builder::predictedValue, JsonpDeserializer.arrayDeserializer(JsonpDeserializer.stringDeserializer()),
"predicted_value");
op.add(Builder::predictedValueSequence, JsonpDeserializer.stringDeserializer(), "predicted_value_sequence");
op.add(Builder::predictionProbability, JsonpDeserializer.doubleDeserializer(), "prediction_probability");
op.add(Builder::topClasses, JsonpDeserializer.arrayDeserializer(TopClassEntry._DESERIALIZER), "top_classes");
op.add(Builder::warning, JsonpDeserializer.stringDeserializer(), "warning");
}
}