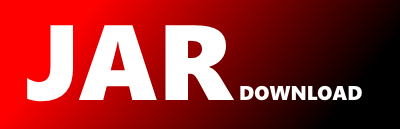
co.elastic.clients.elasticsearch.snapshot.ElasticsearchSnapshotClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.snapshot;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the snapshot namespace.
*/
public class ElasticsearchSnapshotClient extends ApiClient {
public ElasticsearchSnapshotClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchSnapshotClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchSnapshotClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchSnapshotClient(this.transport, transportOptions);
}
// ----- Endpoint: snapshot.cleanup_repository
/**
* Removes stale data from repository.
*
* @see Documentation
* on elastic.co
*/
public CleanupRepositoryResponse cleanupRepository(CleanupRepositoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CleanupRepositoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Removes stale data from repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link CleanupRepositoryRequest}
* @see Documentation
* on elastic.co
*/
public final CleanupRepositoryResponse cleanupRepository(
Function> fn)
throws IOException, ElasticsearchException {
return cleanupRepository(fn.apply(new CleanupRepositoryRequest.Builder()).build());
}
// ----- Endpoint: snapshot.clone
/**
* Clones indices from one snapshot into another snapshot in the same
* repository.
*
* @see Documentation
* on elastic.co
*/
public CloneSnapshotResponse clone(CloneSnapshotRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CloneSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Clones indices from one snapshot into another snapshot in the same
* repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link CloneSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final CloneSnapshotResponse clone(
Function> fn)
throws IOException, ElasticsearchException {
return clone(fn.apply(new CloneSnapshotRequest.Builder()).build());
}
// ----- Endpoint: snapshot.create
/**
* Creates a snapshot in a repository.
*
* @see Documentation
* on elastic.co
*/
public CreateSnapshotResponse create(CreateSnapshotRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a snapshot in a repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final CreateSnapshotResponse create(
Function> fn)
throws IOException, ElasticsearchException {
return create(fn.apply(new CreateSnapshotRequest.Builder()).build());
}
// ----- Endpoint: snapshot.create_repository
/**
* Creates a repository.
*
* @see Documentation
* on elastic.co
*/
public CreateRepositoryResponse createRepository(CreateRepositoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) CreateRepositoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link CreateRepositoryRequest}
* @see Documentation
* on elastic.co
*/
public final CreateRepositoryResponse createRepository(
Function> fn)
throws IOException, ElasticsearchException {
return createRepository(fn.apply(new CreateRepositoryRequest.Builder()).build());
}
// ----- Endpoint: snapshot.delete
/**
* Deletes one or more snapshots.
*
* @see Documentation
* on elastic.co
*/
public DeleteSnapshotResponse delete(DeleteSnapshotRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes one or more snapshots.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteSnapshotResponse delete(
Function> fn)
throws IOException, ElasticsearchException {
return delete(fn.apply(new DeleteSnapshotRequest.Builder()).build());
}
// ----- Endpoint: snapshot.delete_repository
/**
* Deletes a repository.
*
* @see Documentation
* on elastic.co
*/
public DeleteRepositoryResponse deleteRepository(DeleteRepositoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteRepositoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deletes a repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteRepositoryRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteRepositoryResponse deleteRepository(
Function> fn)
throws IOException, ElasticsearchException {
return deleteRepository(fn.apply(new DeleteRepositoryRequest.Builder()).build());
}
// ----- Endpoint: snapshot.get
/**
* Returns information about a snapshot.
*
* @see Documentation
* on elastic.co
*/
public GetSnapshotResponse get(GetSnapshotRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetSnapshotRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns information about a snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetSnapshotRequest}
* @see Documentation
* on elastic.co
*/
public final GetSnapshotResponse get(Function> fn)
throws IOException, ElasticsearchException {
return get(fn.apply(new GetSnapshotRequest.Builder()).build());
}
// ----- Endpoint: snapshot.get_repository
/**
* Returns information about a repository.
*
* @see Documentation
* on elastic.co
*/
public GetRepositoryResponse getRepository(GetRepositoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetRepositoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns information about a repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetRepositoryRequest}
* @see Documentation
* on elastic.co
*/
public final GetRepositoryResponse getRepository(
Function> fn)
throws IOException, ElasticsearchException {
return getRepository(fn.apply(new GetRepositoryRequest.Builder()).build());
}
/**
* Returns information about a repository.
*
* @see Documentation
* on elastic.co
*/
public GetRepositoryResponse getRepository() throws IOException, ElasticsearchException {
return this.transport.performRequest(new GetRepositoryRequest.Builder().build(), GetRepositoryRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: snapshot.restore
/**
* Restores a snapshot.
*
* @see Documentation
* on elastic.co
*/
public RestoreResponse restore(RestoreRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) RestoreRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Restores a snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link RestoreRequest}
* @see Documentation
* on elastic.co
*/
public final RestoreResponse restore(Function> fn)
throws IOException, ElasticsearchException {
return restore(fn.apply(new RestoreRequest.Builder()).build());
}
// ----- Endpoint: snapshot.status
/**
* Returns information about the status of a snapshot.
*
* @see Documentation
* on elastic.co
*/
public SnapshotStatusResponse status(SnapshotStatusRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) SnapshotStatusRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Returns information about the status of a snapshot.
*
* @param fn
* a function that initializes a builder to create the
* {@link SnapshotStatusRequest}
* @see Documentation
* on elastic.co
*/
public final SnapshotStatusResponse status(
Function> fn)
throws IOException, ElasticsearchException {
return status(fn.apply(new SnapshotStatusRequest.Builder()).build());
}
/**
* Returns information about the status of a snapshot.
*
* @see Documentation
* on elastic.co
*/
public SnapshotStatusResponse status() throws IOException, ElasticsearchException {
return this.transport.performRequest(new SnapshotStatusRequest.Builder().build(),
SnapshotStatusRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: snapshot.verify_repository
/**
* Verifies a repository.
*
* @see Documentation
* on elastic.co
*/
public VerifyRepositoryResponse verifyRepository(VerifyRepositoryRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) VerifyRepositoryRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Verifies a repository.
*
* @param fn
* a function that initializes a builder to create the
* {@link VerifyRepositoryRequest}
* @see Documentation
* on elastic.co
*/
public final VerifyRepositoryResponse verifyRepository(
Function> fn)
throws IOException, ElasticsearchException {
return verifyRepository(fn.apply(new VerifyRepositoryRequest.Builder()).build());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy