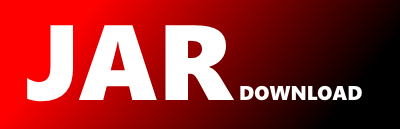
co.elastic.clients.elasticsearch.transform.Pivot Maven / Gradle / Ivy
Show all versions of elasticsearch-java Show documentation
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.transform;
import co.elastic.clients.elasticsearch._types.aggregations.Aggregation;
import co.elastic.clients.json.JsonpDeserializable;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.json.JsonpMapper;
import co.elastic.clients.json.JsonpSerializable;
import co.elastic.clients.json.ObjectBuilderDeserializer;
import co.elastic.clients.json.ObjectDeserializer;
import co.elastic.clients.util.ApiTypeHelper;
import co.elastic.clients.util.ObjectBuilder;
import co.elastic.clients.util.WithJsonObjectBuilderBase;
import jakarta.json.stream.JsonGenerator;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import javax.annotation.Nullable;
// typedef: transform._types.Pivot
/**
*
* @see API
* specification
*/
@JsonpDeserializable
public class Pivot implements JsonpSerializable {
private final Map aggregations;
private final Map groupBy;
// ---------------------------------------------------------------------------------------------
private Pivot(Builder builder) {
this.aggregations = ApiTypeHelper.unmodifiable(builder.aggregations);
this.groupBy = ApiTypeHelper.unmodifiable(builder.groupBy);
}
public static Pivot of(Function> fn) {
return fn.apply(new Builder()).build();
}
/**
* Defines how to aggregate the grouped data. The following aggregations are
* currently supported: average, bucket script, bucket selector, cardinality,
* filter, geo bounds, geo centroid, geo line, max, median absolute deviation,
* min, missing, percentiles, rare terms, scripted metric, stats, sum, terms,
* top metrics, value count, weighted average.
*
* API name: {@code aggregations}
*/
public final Map aggregations() {
return this.aggregations;
}
/**
* Defines how to group the data. More than one grouping can be defined per
* pivot. The following groupings are currently supported: date histogram,
* geotile grid, histogram, terms.
*
* API name: {@code group_by}
*/
public final Map groupBy() {
return this.groupBy;
}
/**
* Serialize this object to JSON.
*/
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if (ApiTypeHelper.isDefined(this.aggregations)) {
generator.writeKey("aggregations");
generator.writeStartObject();
for (Map.Entry item0 : this.aggregations.entrySet()) {
generator.writeKey(item0.getKey());
item0.getValue().serialize(generator, mapper);
}
generator.writeEnd();
}
if (ApiTypeHelper.isDefined(this.groupBy)) {
generator.writeKey("group_by");
generator.writeStartObject();
for (Map.Entry item0 : this.groupBy.entrySet()) {
generator.writeKey(item0.getKey());
item0.getValue().serialize(generator, mapper);
}
generator.writeEnd();
}
}
// ---------------------------------------------------------------------------------------------
/**
* Builder for {@link Pivot}.
*/
public static class Builder extends WithJsonObjectBuilderBase implements ObjectBuilder {
@Nullable
private Map aggregations;
@Nullable
private Map groupBy;
/**
* Defines how to aggregate the grouped data. The following aggregations are
* currently supported: average, bucket script, bucket selector, cardinality,
* filter, geo bounds, geo centroid, geo line, max, median absolute deviation,
* min, missing, percentiles, rare terms, scripted metric, stats, sum, terms,
* top metrics, value count, weighted average.
*
* API name: {@code aggregations}
*
* Adds all entries of map
to aggregations
.
*/
public final Builder aggregations(Map map) {
this.aggregations = _mapPutAll(this.aggregations, map);
return this;
}
/**
* Defines how to aggregate the grouped data. The following aggregations are
* currently supported: average, bucket script, bucket selector, cardinality,
* filter, geo bounds, geo centroid, geo line, max, median absolute deviation,
* min, missing, percentiles, rare terms, scripted metric, stats, sum, terms,
* top metrics, value count, weighted average.
*
* API name: {@code aggregations}
*
* Adds an entry to aggregations
.
*/
public final Builder aggregations(String key, Aggregation value) {
this.aggregations = _mapPut(this.aggregations, key, value);
return this;
}
/**
* Defines how to aggregate the grouped data. The following aggregations are
* currently supported: average, bucket script, bucket selector, cardinality,
* filter, geo bounds, geo centroid, geo line, max, median absolute deviation,
* min, missing, percentiles, rare terms, scripted metric, stats, sum, terms,
* top metrics, value count, weighted average.
*
* API name: {@code aggregations}
*
* Adds an entry to aggregations
using a builder lambda.
*/
public final Builder aggregations(String key, Function> fn) {
return aggregations(key, fn.apply(new Aggregation.Builder()).build());
}
/**
* Defines how to group the data. More than one grouping can be defined per
* pivot. The following groupings are currently supported: date histogram,
* geotile grid, histogram, terms.
*
* API name: {@code group_by}
*
* Adds all entries of map
to groupBy
.
*/
public final Builder groupBy(Map map) {
this.groupBy = _mapPutAll(this.groupBy, map);
return this;
}
/**
* Defines how to group the data. More than one grouping can be defined per
* pivot. The following groupings are currently supported: date histogram,
* geotile grid, histogram, terms.
*
* API name: {@code group_by}
*
* Adds an entry to groupBy
.
*/
public final Builder groupBy(String key, PivotGroupBy value) {
this.groupBy = _mapPut(this.groupBy, key, value);
return this;
}
/**
* Defines how to group the data. More than one grouping can be defined per
* pivot. The following groupings are currently supported: date histogram,
* geotile grid, histogram, terms.
*
* API name: {@code group_by}
*
* Adds an entry to groupBy
using a builder lambda.
*/
public final Builder groupBy(String key, Function> fn) {
return groupBy(key, fn.apply(new PivotGroupBy.Builder()).build());
}
@Override
protected Builder self() {
return this;
}
/**
* Builds a {@link Pivot}.
*
* @throws NullPointerException
* if some of the required fields are null.
*/
public Pivot build() {
_checkSingleUse();
return new Pivot(this);
}
}
// ---------------------------------------------------------------------------------------------
/**
* Json deserializer for {@link Pivot}
*/
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer.lazy(Builder::new,
Pivot::setupPivotDeserializer);
protected static void setupPivotDeserializer(ObjectDeserializer op) {
op.add(Builder::aggregations, JsonpDeserializer.stringMapDeserializer(Aggregation._DESERIALIZER),
"aggregations", "aggs");
op.add(Builder::groupBy, JsonpDeserializer.stringMapDeserializer(PivotGroupBy._DESERIALIZER), "group_by");
}
}