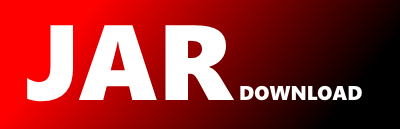
co.elastic.clients.elasticsearch.watcher.ElasticsearchWatcherAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.watcher;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the watcher namespace.
*/
public class ElasticsearchWatcherAsyncClient
extends
ApiClient {
public ElasticsearchWatcherAsyncClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchWatcherAsyncClient(ElasticsearchTransport transport,
@Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchWatcherAsyncClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchWatcherAsyncClient(this.transport, transportOptions);
}
// ----- Endpoint: watcher.ack_watch
/**
* Acknowledges a watch, manually throttling the execution of the watch's
* actions.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture ackWatch(AckWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AckWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Acknowledges a watch, manually throttling the execution of the watch's
* actions.
*
* @param fn
* a function that initializes a builder to create the
* {@link AckWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture ackWatch(
Function> fn) {
return ackWatch(fn.apply(new AckWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.activate_watch
/**
* Activates a currently inactive watch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture activateWatch(ActivateWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ActivateWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Activates a currently inactive watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link ActivateWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture activateWatch(
Function> fn) {
return activateWatch(fn.apply(new ActivateWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.deactivate_watch
/**
* Deactivates a currently active watch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deactivateWatch(DeactivateWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeactivateWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Deactivates a currently active watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeactivateWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deactivateWatch(
Function> fn) {
return deactivateWatch(fn.apply(new DeactivateWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.delete_watch
/**
* Removes a watch from Watcher.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture deleteWatch(DeleteWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Removes a watch from Watcher.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture deleteWatch(
Function> fn) {
return deleteWatch(fn.apply(new DeleteWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.execute_watch
/**
* Forces the execution of a stored watch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture executeWatch(ExecuteWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExecuteWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Forces the execution of a stored watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExecuteWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture executeWatch(
Function> fn) {
return executeWatch(fn.apply(new ExecuteWatchRequest.Builder()).build());
}
/**
* Forces the execution of a stored watch.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture executeWatch() {
return this.transport.performRequestAsync(new ExecuteWatchRequest.Builder().build(),
ExecuteWatchRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: watcher.get_watch
/**
* Retrieves a watch by its ID.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture getWatch(GetWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves a watch by its ID.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture getWatch(
Function> fn) {
return getWatch(fn.apply(new GetWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.put_watch
/**
* Creates a new watch, or updates an existing one.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture putWatch(PutWatchRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutWatchRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Creates a new watch, or updates an existing one.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutWatchRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture putWatch(
Function> fn) {
return putWatch(fn.apply(new PutWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.query_watches
/**
* Retrieves stored watches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryWatches(QueryWatchesRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryWatchesRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves stored watches.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryWatchesRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture queryWatches(
Function> fn) {
return queryWatches(fn.apply(new QueryWatchesRequest.Builder()).build());
}
/**
* Retrieves stored watches.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture queryWatches() {
return this.transport.performRequestAsync(new QueryWatchesRequest.Builder().build(),
QueryWatchesRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: watcher.start
/**
* Starts Watcher if it is not already running.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture start() {
return this.transport.performRequestAsync(StartWatcherRequest._INSTANCE, StartWatcherRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: watcher.stats
/**
* Retrieves the current Watcher metrics.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stats(WatcherStatsRequest request) {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) WatcherStatsRequest._ENDPOINT;
return this.transport.performRequestAsync(request, endpoint, this.transportOptions);
}
/**
* Retrieves the current Watcher metrics.
*
* @param fn
* a function that initializes a builder to create the
* {@link WatcherStatsRequest}
* @see Documentation
* on elastic.co
*/
public final CompletableFuture stats(
Function> fn) {
return stats(fn.apply(new WatcherStatsRequest.Builder()).build());
}
/**
* Retrieves the current Watcher metrics.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stats() {
return this.transport.performRequestAsync(new WatcherStatsRequest.Builder().build(),
WatcherStatsRequest._ENDPOINT, this.transportOptions);
}
// ----- Endpoint: watcher.stop
/**
* Stops Watcher if it is running.
*
* @see Documentation
* on elastic.co
*/
public CompletableFuture stop() {
return this.transport.performRequestAsync(StopWatcherRequest._INSTANCE, StopWatcherRequest._ENDPOINT,
this.transportOptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy