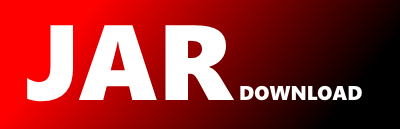
co.elastic.clients.elasticsearch.watcher.ElasticsearchWatcherClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
//----------------------------------------------------
// THIS CODE IS GENERATED. MANUAL EDITS WILL BE LOST.
//----------------------------------------------------
package co.elastic.clients.elasticsearch.watcher;
import co.elastic.clients.ApiClient;
import co.elastic.clients.elasticsearch._types.ElasticsearchException;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.transport.ElasticsearchTransport;
import co.elastic.clients.transport.Endpoint;
import co.elastic.clients.transport.JsonEndpoint;
import co.elastic.clients.transport.Transport;
import co.elastic.clients.transport.TransportOptions;
import co.elastic.clients.util.ObjectBuilder;
import java.io.IOException;
import java.util.function.Function;
import javax.annotation.Nullable;
/**
* Client for the watcher namespace.
*/
public class ElasticsearchWatcherClient extends ApiClient {
public ElasticsearchWatcherClient(ElasticsearchTransport transport) {
super(transport, null);
}
public ElasticsearchWatcherClient(ElasticsearchTransport transport, @Nullable TransportOptions transportOptions) {
super(transport, transportOptions);
}
@Override
public ElasticsearchWatcherClient withTransportOptions(@Nullable TransportOptions transportOptions) {
return new ElasticsearchWatcherClient(this.transport, transportOptions);
}
// ----- Endpoint: watcher.ack_watch
/**
* Acknowledges a watch, manually throttling the execution of the watch's
* actions.
*
* @see Documentation
* on elastic.co
*/
public AckWatchResponse ackWatch(AckWatchRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) AckWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Acknowledges a watch, manually throttling the execution of the watch's
* actions.
*
* @param fn
* a function that initializes a builder to create the
* {@link AckWatchRequest}
* @see Documentation
* on elastic.co
*/
public final AckWatchResponse ackWatch(Function> fn)
throws IOException, ElasticsearchException {
return ackWatch(fn.apply(new AckWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.activate_watch
/**
* Activates a currently inactive watch.
*
* @see Documentation
* on elastic.co
*/
public ActivateWatchResponse activateWatch(ActivateWatchRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ActivateWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Activates a currently inactive watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link ActivateWatchRequest}
* @see Documentation
* on elastic.co
*/
public final ActivateWatchResponse activateWatch(
Function> fn)
throws IOException, ElasticsearchException {
return activateWatch(fn.apply(new ActivateWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.deactivate_watch
/**
* Deactivates a currently active watch.
*
* @see Documentation
* on elastic.co
*/
public DeactivateWatchResponse deactivateWatch(DeactivateWatchRequest request)
throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeactivateWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Deactivates a currently active watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeactivateWatchRequest}
* @see Documentation
* on elastic.co
*/
public final DeactivateWatchResponse deactivateWatch(
Function> fn)
throws IOException, ElasticsearchException {
return deactivateWatch(fn.apply(new DeactivateWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.delete_watch
/**
* Removes a watch from Watcher.
*
* @see Documentation
* on elastic.co
*/
public DeleteWatchResponse deleteWatch(DeleteWatchRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) DeleteWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Removes a watch from Watcher.
*
* @param fn
* a function that initializes a builder to create the
* {@link DeleteWatchRequest}
* @see Documentation
* on elastic.co
*/
public final DeleteWatchResponse deleteWatch(
Function> fn)
throws IOException, ElasticsearchException {
return deleteWatch(fn.apply(new DeleteWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.execute_watch
/**
* Forces the execution of a stored watch.
*
* @see Documentation
* on elastic.co
*/
public ExecuteWatchResponse executeWatch(ExecuteWatchRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) ExecuteWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Forces the execution of a stored watch.
*
* @param fn
* a function that initializes a builder to create the
* {@link ExecuteWatchRequest}
* @see Documentation
* on elastic.co
*/
public final ExecuteWatchResponse executeWatch(
Function> fn)
throws IOException, ElasticsearchException {
return executeWatch(fn.apply(new ExecuteWatchRequest.Builder()).build());
}
/**
* Forces the execution of a stored watch.
*
* @see Documentation
* on elastic.co
*/
public ExecuteWatchResponse executeWatch() throws IOException, ElasticsearchException {
return this.transport.performRequest(new ExecuteWatchRequest.Builder().build(), ExecuteWatchRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: watcher.get_watch
/**
* Retrieves a watch by its ID.
*
* @see Documentation
* on elastic.co
*/
public GetWatchResponse getWatch(GetWatchRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) GetWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves a watch by its ID.
*
* @param fn
* a function that initializes a builder to create the
* {@link GetWatchRequest}
* @see Documentation
* on elastic.co
*/
public final GetWatchResponse getWatch(Function> fn)
throws IOException, ElasticsearchException {
return getWatch(fn.apply(new GetWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.put_watch
/**
* Creates a new watch, or updates an existing one.
*
* @see Documentation
* on elastic.co
*/
public PutWatchResponse putWatch(PutWatchRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) PutWatchRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Creates a new watch, or updates an existing one.
*
* @param fn
* a function that initializes a builder to create the
* {@link PutWatchRequest}
* @see Documentation
* on elastic.co
*/
public final PutWatchResponse putWatch(Function> fn)
throws IOException, ElasticsearchException {
return putWatch(fn.apply(new PutWatchRequest.Builder()).build());
}
// ----- Endpoint: watcher.query_watches
/**
* Retrieves stored watches.
*
* @see Documentation
* on elastic.co
*/
public QueryWatchesResponse queryWatches(QueryWatchesRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) QueryWatchesRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves stored watches.
*
* @param fn
* a function that initializes a builder to create the
* {@link QueryWatchesRequest}
* @see Documentation
* on elastic.co
*/
public final QueryWatchesResponse queryWatches(
Function> fn)
throws IOException, ElasticsearchException {
return queryWatches(fn.apply(new QueryWatchesRequest.Builder()).build());
}
/**
* Retrieves stored watches.
*
* @see Documentation
* on elastic.co
*/
public QueryWatchesResponse queryWatches() throws IOException, ElasticsearchException {
return this.transport.performRequest(new QueryWatchesRequest.Builder().build(), QueryWatchesRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: watcher.start
/**
* Starts Watcher if it is not already running.
*
* @see Documentation
* on elastic.co
*/
public StartWatcherResponse start() throws IOException, ElasticsearchException {
return this.transport.performRequest(StartWatcherRequest._INSTANCE, StartWatcherRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: watcher.stats
/**
* Retrieves the current Watcher metrics.
*
* @see Documentation
* on elastic.co
*/
public WatcherStatsResponse stats(WatcherStatsRequest request) throws IOException, ElasticsearchException {
@SuppressWarnings("unchecked")
JsonEndpoint endpoint = (JsonEndpoint) WatcherStatsRequest._ENDPOINT;
return this.transport.performRequest(request, endpoint, this.transportOptions);
}
/**
* Retrieves the current Watcher metrics.
*
* @param fn
* a function that initializes a builder to create the
* {@link WatcherStatsRequest}
* @see Documentation
* on elastic.co
*/
public final WatcherStatsResponse stats(
Function> fn)
throws IOException, ElasticsearchException {
return stats(fn.apply(new WatcherStatsRequest.Builder()).build());
}
/**
* Retrieves the current Watcher metrics.
*
* @see Documentation
* on elastic.co
*/
public WatcherStatsResponse stats() throws IOException, ElasticsearchException {
return this.transport.performRequest(new WatcherStatsRequest.Builder().build(), WatcherStatsRequest._ENDPOINT,
this.transportOptions);
}
// ----- Endpoint: watcher.stop
/**
* Stops Watcher if it is running.
*
* @see Documentation
* on elastic.co
*/
public StopWatcherResponse stop() throws IOException, ElasticsearchException {
return this.transport.performRequest(StopWatcherRequest._INSTANCE, StopWatcherRequest._ENDPOINT,
this.transportOptions);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy