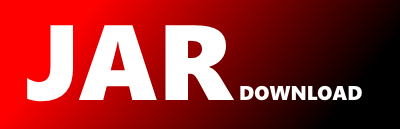
co.elastic.clients.transport.endpoints.SimpleEndpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-java Show documentation
Show all versions of elasticsearch-java Show documentation
Elasticsearch Java API Client
/*
* Licensed to Elasticsearch B.V. under one or more contributor
* license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright
* ownership. Elasticsearch B.V. licenses this file to you under
* the Apache License, Version 2.0 (the "License"); you may
* not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package co.elastic.clients.transport.endpoints;
import co.elastic.clients.elasticsearch._types.ErrorResponse;
import co.elastic.clients.json.JsonpDeserializer;
import co.elastic.clients.transport.JsonEndpoint;
import org.apache.http.client.utils.URLEncodedUtils;
import java.util.Collections;
import java.util.Map;
import java.util.function.Function;
public class SimpleEndpoint implements JsonEndpoint {
private static final Function, Map> EMPTY_MAP = x -> Collections.emptyMap();
/**
* Returns a function that always returns an empty String to String map. Useful to avoid creating lots of
* duplicate lambdas in endpoints that don't have headers or parameters.
*/
@SuppressWarnings("unchecked")
public static Function> emptyMap() {
return (Function>) EMPTY_MAP;
}
private final String id;
private final Function method;
private final Function requestUrl;
private final Function> queryParameters;
private final Function> headers;
private final boolean hasRequestBody;
private final JsonpDeserializer responseParser;
public SimpleEndpoint(
String id,
Function method,
Function requestUrl,
Function> queryParameters,
Function> headers,
boolean hasRequestBody,
JsonpDeserializer responseParser
) {
this.id = id;
this.method = method;
this.requestUrl = requestUrl;
this.queryParameters = queryParameters;
this.headers = headers;
this.hasRequestBody = hasRequestBody;
this.responseParser = responseParser;
}
@Override
public String id() {
return this.id;
}
@Override
public String method(RequestT request) {
return this.method.apply(request);
}
@Override
public String requestUrl(RequestT request) {
return this.requestUrl.apply(request);
}
@Override
public Map queryParameters(RequestT request) {
return this.queryParameters.apply(request);
}
@Override
public Map headers(RequestT request) {
return this.headers.apply(request);
}
@Override
public boolean hasRequestBody() {
return this.hasRequestBody;
}
@Override
public JsonpDeserializer responseDeserializer() {
return this.responseParser;
}
// ES-specific
@Override
public boolean isError(int statusCode) {
return statusCode >= 400;
}
@Override
public JsonpDeserializer errorDeserializer(int statusCode) {
return ErrorResponse._DESERIALIZER;
}
public SimpleEndpoint withResponseDeserializer(
JsonpDeserializer newResponseParser
) {
return new SimpleEndpoint<>(
id,
method,
requestUrl,
queryParameters,
headers,
hasRequestBody,
newResponseParser
);
}
public static RuntimeException noPathTemplateFound(String what) {
return new RuntimeException("Could not find a request " + what + " with this set of properties. " +
"Please check the API documentation, or raise an issue if this should be a valid request.");
}
public static void pathEncode(String src, StringBuilder dest) {
// TODO: avoid dependency on HttpClient here (and use something more efficient)
dest.append(URLEncodedUtils.formatSegments(src).substring(1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy