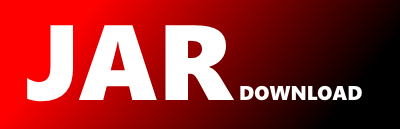
co.fingerprintsoft.notification.clickatell.ClickatellSMSSender Maven / Gradle / Ivy
package co.fingerprintsoft.notification.clickatell;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.Unirest;
import com.mashape.unirest.http.exceptions.UnirestException;
import com.mashape.unirest.request.body.RequestBodyEntity;
import con.fingerprintsoft.notification.sms.SMS;
import con.fingerprintsoft.notification.sms.SMSResponse;
import con.fingerprintsoft.notification.sms.SMSSender;
import con.fingerprintsoft.notification.sms.Status;
import java.io.IOException;
public class ClickatellSMSSender implements SMSSender {
private ClickatellSettings settings;
private ObjectMapper objectMapper;
public ClickatellSMSSender(ClickatellSettings settings, ObjectMapper objectMapper) {
this.settings = settings;
this.objectMapper = objectMapper;
Unirest.setObjectMapper(new com.mashape.unirest.http.ObjectMapper() {
public T readValue(String value, Class valueType) {
try {
return objectMapper.readValue(value, valueType);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public String writeValue(Object value) {
try {
return objectMapper.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
});
}
@Override
public SMSResponse sendSingleSMS(SMS sms) {
ClickatellSMS clickatellSMS = new ClickatellSMS();
clickatellSMS.addTo(parseMobileNumber(sms.getTo().get(0)));
clickatellSMS.setText(clickatellSMS.getText());
RequestBodyEntity requestWithBody = Unirest.post(settings.getUrl())
.header("Authorization", settings.getGrantType() + " " + settings.getToken())
.header("Content-Type", "application/json")
.header("Accept", "application/json")
.header("X-Version", settings.getVersion())
.body(clickatellSMS);
return getSMSResponse(requestWithBody);
}
private SMSResponse getSMSResponse(RequestBodyEntity requestWithBody) {
HttpResponse request = null;
try {
request = requestWithBody.asString();
} catch (UnirestException e) {
throw new RuntimeException(e);
}
ClickatellSMSResponse smsResponse;
switch (request.getStatus()) {
case 202:
String responseDetails = request.getBody();
ClickatellResponse clickatellResponse = null;
try {
clickatellResponse = objectMapper.readValue(responseDetails, ClickatellResponse.class);
} catch (IOException e) {
throw new RuntimeException(e);
}
smsResponse = new ClickatellSMSResponse();
smsResponse.setExternalReference(clickatellResponse.getData().getMessage().get(0).getApiMessageId());
if(clickatellResponse.getData().getMessage().get(0).getAccepted().booleanValue()) {
smsResponse.setStatus(Status.SUCCESSFULL);
} else {
smsResponse.setStatus(Status.FAILED);
}
smsResponse.setHttpStatus(request.getStatus());
smsResponse.setMessage(clickatellResponse.getData().getMessage().get(0).getError());
return smsResponse;
default:
smsResponse = new ClickatellSMSResponse();
smsResponse.setStatus(Status.FAILED);
smsResponse.setHttpStatus(request.getStatus());
return smsResponse;
}
}
String parseMobileNumber(String mobileNumber) {
if (!mobileNumber.startsWith("27") && !mobileNumber.startsWith("+27")) {
mobileNumber = mobileNumber.replaceFirst("0", "27");
}
return mobileNumber;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy