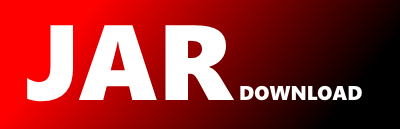
co.fingerprintsoft.payment.paygate.PaymentMethod Maven / Gradle / Ivy
package co.fingerprintsoft.payment.paygate;
import java.util.HashMap;
import java.util.Map;
public enum PaymentMethod {
CREDIT_CARD("CC", "Credit Card"),
DEBIT_CARD("DC", "Debit Card"),
EWALLET("EW", "eWallet"),
BANK_TRANSFER("BT", "Bank Transfer"),
CASH_VOUCHER("CV", "Cash Voucher"),
PRE_PAID_CARD("PC", "Pre Paid Card");
private static final Map METH_TO_ENUM_MAP = new HashMap();
private static final Map DESC_TO_ENUM_MAP = new HashMap();
static {
for (PaymentMethod type : PaymentMethod.values()) {
METH_TO_ENUM_MAP.put(type.getPayMethod(), type);
DESC_TO_ENUM_MAP.put(type.getDescription(), type);
}
}
private String payMethod;
private String description;
PaymentMethod(String payMethod, String description) {
this.payMethod = payMethod;
this.description = description;
}
public String getPayMethod() {
return payMethod;
}
public String getDescription() {
return description;
}
public static PaymentMethod fromDescription(String description) {
if (description == null) {
return null;
}
PaymentMethod type = DESC_TO_ENUM_MAP.get(description);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for description " + description);
}
public static PaymentMethod fromPayMethod(String payMethod) {
if (payMethod == null) {
return null;
}
PaymentMethod type = METH_TO_ENUM_MAP.get(payMethod);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for payMethod " + payMethod);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy