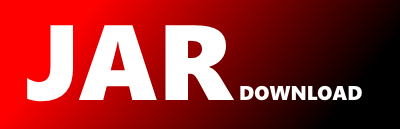
co.fingerprintsoft.payment.paygate.PaymentResult Maven / Gradle / Ivy
package co.fingerprintsoft.payment.paygate;
import java.util.HashMap;
import java.util.Map;
public enum PaymentResult {
CALL_FOR_APPROVAL(900001, "Call for Approval"),
CARD_EXPIRED(900002, "Card Expired"),
INSUFFICIENT_FUNDS(900003, "Insufficient Funds"),
INVALID_CARD_NUMBER(900004, "Invalid Card Number"),
BANK_INTERFACE_TIMEOUT(900005, "Bank Interface Timeout"),
INVALID_CARD(900006, "Invalid Card"),
DECLINED(900007, "Declined"),
LOST_CARD(900009, "Lost Card"),
INVALID_CARD_LENGTH(900010, "Invalid Card Length"),
SUSPECTED_FRAUD(900011, "Suspected Fraud"),
CARD_REPORTED_AS_STOLEN(900012, "Card Reported As Stolen"),
RESTRICTED_CARD(900013, "Restricted Card"),
EXCESSIVE_CARD_USAGE(900014, "Excessive Card Usage"),
CARD_BLACKLISTED(900015, "Card Blacklisted"),
DECLINED_AUTHENTICATION_FAILED(900207, "Declined; authentication failed"),
AUTH_DECLINED(990020, "Auth Declined"),
INVALID_EXPIRY_DATE(991001, "Invalid expiry date"),
INVALID_AMOUNT(991002, "Invalid Amount"),
AUTH_DONE(990017, "Auth Done", true),
AUTH_INCOMPLETE(900201, "Auth Incomplete"),
UNEXPECTED_AUTHENTICATION_RESULT_1(900205, "Unexpected authentication result (phase 1)"),
UNEXPECTED_AUTHENTICATION_RESULT_2(900206, "Unexpected authentication result (phase 1)"),
COULD_NOT_INSERT_INTO_DATABASE(990001, "Could not insert into Database"),
BANK_NOT_AVAILABLE(990022, "Bank not available"),
ERROR_PROCESSING_TRANSACTION(990053, "Error processing transaction"),
TRANSACTION_VERIFICATION_FAILED(900209, "Transaction verification failed (phase 2)"),
AUTHENTICATION_COMPLETE(900210, "Authentication complete; transaction must be restarted"),
DUPLICATE_TRANSACTION_DETECTED(990024, "Duplicate Transaction Detected. Please check before submitting"),
TRANSACTION_CANCELLED(990028, "Transaction cancelled"),
CARD_NOT_FULLY_AUTHENTICATED(900211, "Card not fully authenticated");
private static final Map CODE_TO_ENUM_MAP = new HashMap();
private static final Map DESC_TO_ENUM_MAP = new HashMap();
static {
for (PaymentResult type : PaymentResult.values()) {
CODE_TO_ENUM_MAP.put(type.getCode(), type);
DESC_TO_ENUM_MAP.put(type.getDescription(), type);
}
}
private Integer code;
private String description;
private boolean success;
PaymentResult(Integer code, String description) {
this.code = code;
this.description = description;
}
PaymentResult(Integer code, String description, boolean success) {
this.code = code;
this.description = description;
this.success = success;
}
public Integer getCode() {
return code;
}
public String getDescription() {
return description;
}
public boolean isSuccess() {
return success;
}
public static PaymentResult fromDescription(String description) {
if (description == null) {
return null;
}
PaymentResult type = DESC_TO_ENUM_MAP.get(description);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for description " + description);
}
public static PaymentResult fromCode(Integer code) {
if (code == null) {
return null;
}
PaymentResult type = CODE_TO_ENUM_MAP.get(code);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for code " + code);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy