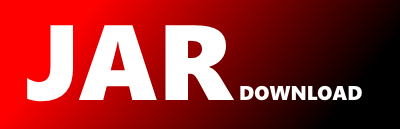
co.fingerprintsoft.payment.paygate.TransactionStatus Maven / Gradle / Ivy
package co.fingerprintsoft.payment.paygate;
import java.util.HashMap;
import java.util.Map;
public enum TransactionStatus {
NOT_DONE(0,"Not Done"),
APPROVED(1, "Approved", true),
DECLINED(2, "Declined"),
CANCELLED(3, "Cancelled"),
USER_CANCELLED(4, "User Cancelled");
private static final Map CODE_TO_ENUM_MAP = new HashMap();
private static final Map DESC_TO_ENUM_MAP = new HashMap();
static {
for (TransactionStatus type : TransactionStatus.values()) {
CODE_TO_ENUM_MAP.put(type.getTransactionCode(), type);
DESC_TO_ENUM_MAP.put(type.getDescription(), type);
}
}
private int transactionCode;
private String description;
private boolean success;
TransactionStatus(int transactionCode, String description) {
this.transactionCode = transactionCode;
this.description = description;
}
TransactionStatus(int transactionCode, String description, boolean success) {
this.transactionCode = transactionCode;
this.description = description;
this.success = success;
}
public int getTransactionCode() {
return transactionCode;
}
public String getDescription() {
return description;
}
public boolean isSuccess() {
return success;
}
public static TransactionStatus fromDescription(String description) {
if (description == null) {
return null;
}
TransactionStatus type = DESC_TO_ENUM_MAP.get(description);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for description " + description);
}
public static TransactionStatus fromTransactionCode(Integer transactionCode) {
if (transactionCode == null) {
return null;
}
TransactionStatus type = CODE_TO_ENUM_MAP.get(transactionCode);
if(type != null) {
return type;
}
throw new IllegalArgumentException("No matching type for transactionCode " + transactionCode);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy