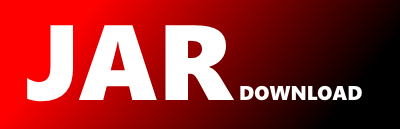
co.fingerprintsoft.payment.paygate.api.PayGatePaymentService Maven / Gradle / Ivy
package co.fingerprintsoft.payment.paygate.api;
import co.fingerprintsoft.payment.paygate.FieldNameConstants;
import co.fingerprintsoft.payment.paygate.domain.Error;
import co.fingerprintsoft.payment.paygate.domain.*;
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.Unirest;
import com.mashape.unirest.http.exceptions.UnirestException;
import com.mashape.unirest.request.body.MultipartBody;
import lombok.extern.slf4j.Slf4j;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.security.NoSuchAlgorithmException;
import java.util.HashMap;
import java.util.Map;
import java.util.StringTokenizer;
import static co.fingerprintsoft.payment.paygate.FieldNameConstants.*;
@Slf4j
public class PayGatePaymentService {
private PayGateSettings payGateSettings;
public PayGatePaymentService(PayGateSettings payGateSettings) {
this.payGateSettings = payGateSettings;
}
public PaymentResponse paymentRequest(PaymentRequest request) throws UnirestException, NoSuchAlgorithmException, UnsupportedEncodingException {
String strLocalDate = request.getFormattedDate();
MultipartBody post = Unirest.post(PayWebConstants.INITIATE)
.field(PAYGATE_ID, payGateSettings.getPayGateId())
.field(REFERENCE, request.getReference())
.field(FieldNameConstants.AMOUNT, request.getAmount())
.field(FieldNameConstants.CURRENCY, request.getCurrency().getCurrencyCode())
.field(FieldNameConstants.RETURN_URL, payGateSettings.getReturnUrl())
.field(FieldNameConstants.TRANSACTION_DATE, strLocalDate)
.field(FieldNameConstants.LOCALE, request.getLocale().getCode())
.field(FieldNameConstants.COUNTRY, request.getCountry().getCountryCode())
.field(FieldNameConstants.EMAIL, request.getEmail());
if (payGateSettings.getNotifyUrl() != null) {
post.field("NOTIFY_URL", payGateSettings.getNotifyUrl());
}
request.getPayMethod().ifPresent(value -> post.field(FieldNameConstants.PAY_METHOD, value));
request.getPayMethodDetail().ifPresent(value -> post.field(FieldNameConstants.PAY_METHOD_DETAIL, value));
request.getUser1().ifPresent(value -> post.field(FieldNameConstants.USER1, value));
request.getUser2().ifPresent(value -> post.field(FieldNameConstants.USER2, value));
request.getUser3().ifPresent(value -> post.field(FieldNameConstants.USER3, value));
request.getVault().ifPresent(value -> post.field(FieldNameConstants.VAULT, value));
request.getVaultId().ifPresent(value -> post.field(FieldNameConstants.VAULT_ID, value));
post.field(CHECKSUM, request.calculateChecksum(payGateSettings));
HttpResponse response = post.asString();
String responseDetail = URLDecoder.decode(response.getBody(), "UTF-8");
log.debug("Response Detail : {}", responseDetail);
StringTokenizer tokenizer = new StringTokenizer(responseDetail, "&");
Map responseMap = new HashMap();
while (tokenizer.hasMoreElements()) {
String[] split = tokenizer.nextToken().split("=");
responseMap.put(split[0], split[1]);
}
PaymentResponse paymentResponse = PaymentResponse.constructFromMap(responseMap);
paymentResponse.setHttpStatus(response.getStatus());
if (Status.SUCCESS.equals(paymentResponse.getStatus()) && !payGateSettings.getPayGateId().equals(paymentResponse
.getPayGateId())) {
paymentResponse.setStatus(Status.VERIFICATION_FAILURE);
paymentResponse.setError(Error.PGID_MOD);
}
return paymentResponse;
}
public NotifyResponse paymentStatusCheck(TransactionStatusRequest statusCheck)
throws UnirestException, NoSuchAlgorithmException, UnsupportedEncodingException {
MultipartBody post = Unirest.post(PayWebConstants.QUERY)
.field(PAYGATE_ID, payGateSettings.getPayGateId())
.field(PAY_REQUEST_ID, statusCheck.getPayRequestId())
.field(REFERENCE, statusCheck.getReference());
post.field(CHECKSUM,
statusCheck.calculateChecksum(payGateSettings.getEncryptionKey(), payGateSettings.getPayGateId())
);
HttpResponse response = post.asString();
String responseDetail = URLDecoder.decode(response.getBody(), "UTF-8");
log.debug("Response Detail : {}", responseDetail);
StringTokenizer tokenizer = new StringTokenizer(responseDetail, "&");
Map responseMap = new HashMap();
while (tokenizer.hasMoreElements()) {
String[] split = tokenizer.nextToken().split("=");
if (split.length == 2) {
responseMap.put(split[0], split[1]);
}
}
NotifyResponse notifyResponse = NotifyResponse.constructFromMap(responseMap);
notifyResponse.setHttpStatus(response.getStatus());
if (Status.SUCCESS.equals(notifyResponse.getStatus()) && !payGateSettings.getPayGateId().equals(notifyResponse
.getPayGateId())) {
notifyResponse.setStatus(Status.VERIFICATION_FAILURE);
notifyResponse.setError(Error.ND_INV_PGID);
}
return notifyResponse;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy