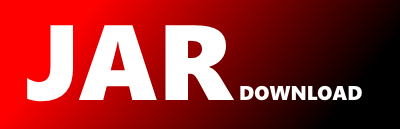
co.leantechniques.maven.buildtime.MojoTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-buildtime-extension Show documentation
Show all versions of maven-buildtime-extension Show documentation
This extension displays the duration of each plugin
to discover why your build is slow.
The newest version!
package co.leantechniques.maven.buildtime;
import java.lang.Comparable;
public class MojoTimer implements Comparable {
private final String projectName;
private String name;
private long startTime = 0;
private long endTime = 0;
private SystemClock systemClock;
public MojoTimer(String projectName, String name, SystemClock systemClock) {
this(projectName, name, 0,0, systemClock);
}
public MojoTimer(String projectName, String name, long startTime, long endTime){
this(projectName, name, startTime, endTime, new SystemClock());
}
public MojoTimer(String projectName, String name, long startTime, long endTime, SystemClock systemClock) {
this.projectName = projectName;
this.name = name;
this.startTime = startTime;
this.endTime = endTime;
this.systemClock = systemClock;
}
public Long getDuration() {
return endTime - startTime;
}
public String getName() {
return name;
}
public String getProjectName() {
return projectName;
}
public void stop() {
this.endTime = systemClock.currentTimeMillis();
}
public void start() {
this.startTime = systemClock.currentTimeMillis();
}
public void accept(TimerVisitor visitor){
visitor.visit(this);
}
public int compareTo(MojoTimer that) {
if (that == null)
return 1;
if (this == that)
return 0;
if (this.startTime > that.startTime)
return 1;
else if (this.startTime < that.startTime)
return -1;
return 0;
}
public long getStartTime() {
return startTime;
}
public long getEndTime() {
return endTime;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy