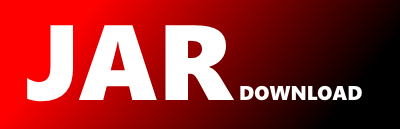
com.openfin.desktop.channel.ChannelProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openfin-desktop-java-adapter Show documentation
Show all versions of openfin-desktop-java-adapter Show documentation
The Java API for OpenFin Runtime
package com.openfin.desktop.channel;
import java.util.ArrayList;
import java.util.Iterator;
import org.json.JSONObject;
import com.openfin.desktop.AckListener;
public class ChannelProvider extends ChannelBase {
ArrayList clients;
ChannelProvider(Channel messageChannelFactory, JSONObject providerIdentity) {
super(messageChannelFactory, providerIdentity);
this.clients = new ArrayList<>();
}
public void processConnection(JSONObject clientIdentity, JSONObject connectionPayload) {
this.clients.add(clientIdentity);
this.channel.addChannelListener(new ChannelListener() {
@Override
public void onChannelConnect(ConnectionEvent connectionEvent) {
}
@Override
public void onChannelDisconnect(ConnectionEvent connectionEvent) {
if (connectionEvent.getName().equals(clientIdentity.getString("name"))
&& connectionEvent.getUuid().equals(clientIdentity.getString("uuid"))) {
processDisconnection(clientIdentity);
}
}
});
}
public void processDisconnection(JSONObject clientIdentity) {
String uuid = clientIdentity.getString("uuid");
String name = clientIdentity.getString("name");
Iterator allClients = this.clients.iterator();
while (allClients.hasNext()) {
JSONObject client = allClients.next();
if (client.getString("uuid").equals(uuid) && client.getString("name").equals(name)) {
allClients.remove();
}
}
}
public void publish(String action, JSONObject actionPayload, AckListener ackListener) {
for (JSONObject client : this.clients) {
this.dispatch(client, action, actionPayload, ackListener);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy