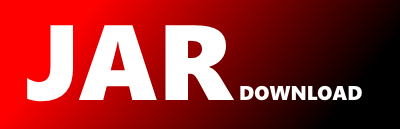
com.openfin.desktop.WindowMember Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openfin-snap-dock Show documentation
Show all versions of openfin-snap-dock Show documentation
OpenFin Window Docking Manager
The newest version!
package com.openfin.desktop;
import com.openfin.desktop.win32.ExternalWindowObserver;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.List;
/**
* Member registered with DockingMember
*
* Created by wche on 3/5/2016.
*/
class WindowMember {
private final static Logger logger = LoggerFactory.getLogger(WindowMember.class.getName());
private Window window;
private boolean isDocked;
private boolean isSnapped;
private WindowBounds bounds; // current bounds
private WindowMember anchor; // window this window is docked into
private List dockedChildren; // windows docked to this one
private AckListener ackListener;
private ExternalWindowObserver externalWindowObserver; // set for external Java window
private DesktopConnection desktopConnection;
WindowMember(Window window, DesktopConnection desktopConnection) {
this.window = window;
this.desktopConnection = desktopConnection;
this.isDocked = false;
this.isSnapped = false;
this.dockedChildren = new ArrayList<>();
this.ackListener = new AckListener() {
@Override
public void onSuccess(Ack ack) {
if (!ack.isSuccessful()) {
logger.error(String.format("notSuccessful %s", ack.getJsonObject().toString()));
}
}
@Override
public void onError(Ack ack) {
logger.error(String.format("onError %s", ack.getJsonObject().toString()));
}
};
}
static String getMemberKey(String appUuid, String windowName) {
return String.format("%s:%s", appUuid, windowName);
}
static String getMemberKey(Window window) {
return getMemberKey(window.getUuid(), window.getName());
}
public String getKey() {
return getMemberKey(this.window);
}
public Window getWindow() {
return window;
}
public boolean isDocked() {
return isDocked;
}
public void setDocked(boolean docked) {
isDocked = docked;
try {
JSONObject message = new JSONObject();
message.put("applicationUuid", this.window.getUuid());
message.put("windowName", this.window.getName());
String topic = docked ? "window-docked" : "window-undocked";
this.desktopConnection.getInterApplicationBus().publish(topic, message, this.ackListener);
} catch (Exception ex) {
logger.error(String.format("Error publishing docking status %s", this.getKey()), ex);
}
}
public boolean isSnapped() {
return isSnapped;
}
public void setSnapped(boolean snapped) {
isSnapped = snapped;
}
public WindowBounds getBounds() {
return bounds;
}
void setExternalWindowObserver(ExternalWindowObserver externalWindowObserver) {
this.externalWindowObserver = externalWindowObserver;
}
ExternalWindowObserver getExternalWindowObserver() {
return this.externalWindowObserver;
}
public void setBounds(WindowBounds bounds) {
this.bounds = bounds;
}
public void updateBounds(WindowBounds bounds) {
this.setBounds(bounds);
logger.debug(String.format("setBounds %s %s", getKey(), bounds.toString()));
this.window.setBounds(bounds.getLeft(), bounds.getTop(), bounds.getWidth(), bounds.getHeight(), ackListener);
}
void setAnchor(WindowMember member) {
this.anchor = member;
}
WindowMember getAnchor() {
return this.anchor;
}
List getDockedChildren() {
return this.dockedChildren;
}
/**
* Dock movingMember to this instance
* @param movingMember
*/
public void dock(WindowMember movingMember) {
try {
logger.debug(String.format("Docking %s to %s", movingMember.getKey(), this.getKey()));
movingMember.getWindow().joinGroup(this.window, this.ackListener);
this.addDockChild(movingMember);
this.setDocked(true);
movingMember.setDocked(true);
} catch (Exception ex) {
logger.error(String.format("Error docking window %s", this.getKey()), ex);
}
}
private void addDockChild(WindowMember child) {
logger.debug(String.format("Add to docked children %s %s", this.getKey(), child.getKey()));
child.setAnchor(this);
this.dockedChildren.add(child);
}
/**
* Check if this is docked to any window
* @return
*/
private boolean isStandAlone() {
return this.anchor == null && this.dockedChildren.size() == 0;
}
/**
*
* Undock this window from its group. All windows docked to this window will be undocked as well
*
*/
public void undock() {
undock(true);
}
private void undock(boolean removeFromAnchor) {
logger.debug(String.format("Undock %s", this.getKey()));
if (isDocked()) {
try {
this.getWindow().leaveGroup(this.ackListener);
this.setDocked(false);
// this.dockedChildren.forEach(child -> {
// child.undock();
// });
for (WindowMember child : this.dockedChildren) {
child.undock(false);
}
this.dockedChildren.clear();
if (this.getAnchor() != null) {
WindowMember anchor = this.getAnchor();
if (removeFromAnchor) {
anchor.getDockedChildren().remove(this);
if (anchor.isStandAlone()) {
anchor.undock(true);
}
}
this.setAnchor(null);
}
} catch (Exception ex) {
logger.error(String.format("Error undocking window %s", this.getKey()), ex);
}
}
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
WindowMember that = (WindowMember) o;
return getMemberKey(window).equals(getMemberKey(that.window));
}
@Override
public int hashCode() {
return getMemberKey(this.window).hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy