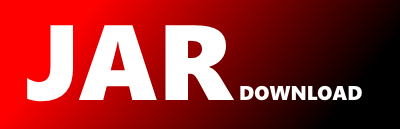
co.paralleluniverse.actors.LocalActorRef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quasar-actors Show documentation
Show all versions of quasar-actors Show documentation
lightweight threads and actors for the JVM.
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package co.paralleluniverse.actors;
import co.paralleluniverse.fibers.Joinable;
import co.paralleluniverse.fibers.SuspendExecution;
import co.paralleluniverse.strands.channels.SendPort;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
/**
*
* @author pron
*/
final class LocalActorRef extends ActorRefImpl implements ActorBuilder, Joinable, java.io.Serializable {
private Actor actor;
public LocalActorRef(Actor actor, String name, SendPort
© 2015 - 2025 Weber Informatics LLC | Privacy Policy