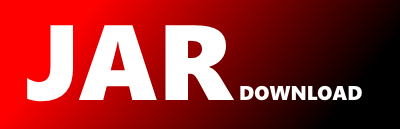
co.paralleluniverse.actors.behaviors.MessageSelector Maven / Gradle / Ivy
/*
* Copyright (c) 2013-2014, Parallel Universe Software Co. All rights reserved.
*
* This program and the accompanying materials are dual-licensed under
* either the terms of the Eclipse Public License v1.0 as published by
* the Eclipse Foundation
*
* or (per the licensee's choosing)
*
* under the terms of the GNU Lesser General Public License version 3.0
* as published by the Free Software Foundation.
*/
package co.paralleluniverse.actors.behaviors;
import co.paralleluniverse.actors.ActorRef;
import co.paralleluniverse.actors.MessageProcessor;
import java.util.Objects;
/**
* A fluent interface for creating {@link MessageProcessor}s that select messages matching a few simple criteria.
*
* @author pron
*/
public abstract class MessageSelector implements MessageProcessor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy