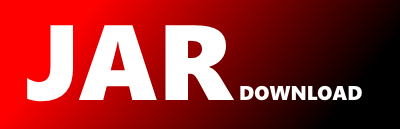
co.paralleluniverse.kotlin.Kotlin.kt Maven / Gradle / Ivy
/*
* Quasar: lightweight threads and actors for the JVM.
* Copyright (c) 2015, Parallel Universe Software Co. All rights reserved.
*
* This program and the accompanying materials are dual-licensed under
* either the terms of the Eclipse Public License v1.0 as published by
* the Eclipse Foundation
*
* or (per the licensee's choosing)
*
* under the terms of the GNU Lesser General Public License version 3.0
* as published by the Free Software Foundation.
*/
package co.paralleluniverse.kotlin
import co.paralleluniverse.fibers.Fiber
import co.paralleluniverse.fibers.FiberScheduler
import co.paralleluniverse.fibers.SuspendExecution
import co.paralleluniverse.fibers.Suspendable
import co.paralleluniverse.strands.SuspendableCallable
import co.paralleluniverse.strands.channels.*
import java.util.concurrent.TimeUnit
/**
* @author circlespainter
*/
Suspendable public fun fiber(start: Boolean, name: String?, scheduler: FiberScheduler?, stackSize: Int, block: () -> T): Fiber {
val sc = @Suspendable object : SuspendableCallable {
@throws(SuspendExecution::class) Suspendable override fun run(): T = block()
}
val ret =
if (scheduler != null)
Fiber(name, scheduler, stackSize, sc)
else
Fiber(name, stackSize, sc)
if (start) ret.start()
return ret
}
Suspendable public fun fiber(block: () -> T): Fiber =
fiber(true, null, null, -1, block)
Suspendable public fun fiber(start: Boolean, block: () -> T): Fiber =
fiber(start, null, null, -1, block)
Suspendable public fun fiber(name: String, block: () -> T): Fiber =
fiber(true, name, null, -1, block)
Suspendable public fun fiber(scheduler: FiberScheduler, block: () -> T): Fiber =
fiber(true, null, scheduler, -1, block)
Suspendable public fun fiber(name: String, scheduler: FiberScheduler, block: () -> T): Fiber =
fiber(true, name, scheduler, -1, block)
Suspendable public fun fiber(start: Boolean, scheduler: FiberScheduler, block: () -> T): Fiber =
fiber(start, null, scheduler, -1, block)
Suspendable public fun fiber(start: Boolean, name: String, scheduler: FiberScheduler, block: () -> T): Fiber =
fiber(start, name, scheduler, -1, block)
public abstract data class SelectOp(private val wrappedSA: SelectAction) {
public fun getWrappedSelectAction(): SelectAction = wrappedSA
}
public data class Receive(public val receivePort: ReceivePort) : SelectOp(Selector.receive(receivePort)) {
public var msg: M = null
internal set(value) {
$msg = value
}
get() = $msg
}
public data class Send(public val sendPort: SendPort, public val msg: M) : SelectOp(Selector.send(sendPort, msg))
Suspendable public fun select(actions: List>, b: (SelectOp?) -> R, priority: Boolean = false, timeout: Int = -1, unit: TimeUnit = TimeUnit.MILLISECONDS): R {
val sa = Selector.select(priority, timeout.toLong(), unit, actions.map{it.getWrappedSelectAction()}.toList())
if (sa != null) {
val sOp = actions.get(sa.index())
when (sOp) {
is Receive -> sOp.msg = sa.message()
}
return b(sOp)
} else
return b(null)
}
Suspendable public fun select(vararg actions: SelectOp, b: (SelectOp?) -> R): R = select(actions.toList(), b)
Suspendable public fun select(timeout: Int, unit: TimeUnit, vararg actions: SelectOp, b: (SelectOp?) -> R): R =
select(actions.toList(), b, false, timeout, unit)
Suspendable public fun select(priority: Boolean, timeout: Int, unit: TimeUnit, vararg actions: SelectOp, b: (SelectOp?) -> R): R =
select(actions.toList(), b, priority, timeout, unit)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy