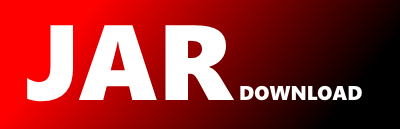
co.privacyone.sdk.ldar.LdarSdk Maven / Gradle / Ivy
/*************************************************************************
*
* Privacy1 AB CONFIDENTIAL
* ________________________
*
* [2017] - [2020] Privacy1 AB
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of Privacy1 AB. The intellectual and technical concepts contained herein
* are proprietary to Privacy1 AB and may be covered by European, U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright law.
*
* Dissemination of this information or reproduction of this material
* is strictly forbidden.
*/
package co.privacyone.sdk.ldar;
import javax.annotation.Nonnull;
import co.privacyone.sdk.ldar.client.LdarClient;
/**
* Java SDK for accessing the ldar service.
*
*/
public class LdarSdk {
/**
* Create a new {@link LdarSdkBuilder}
*
* @return LarSdkBuilder
*/
public static LdarSdkBuilder builder() {
return new LdarSdkBuilder();
}
/**
* Use this builder to construct the Ldar manager. You can create either a sync version or an
* async version of the Ldar SDK manager.
*/
public static class LdarSdkBuilder {
private String ldarHost;
private int ldarPort = 8080;
private String authHost;
private int authPort;
private String serviceName;
private String servicePassword;
LdarSdkBuilder() {
super();
}
/**
* Build ldar sdk object with its remote host name.
*
* @param ldarHost the host of the deployed ldar service
* @return the ldar sdk builder object
*/
@Nonnull
public LdarSdkBuilder ldarHost(final String ldarHost) {
this.ldarHost = ldarHost;
return this;
}
/**
* Build ldar sdk object with its ldar service port.
*
* @param ldarPort the port of the deployed ldar service
* @return the ldar sdk builder object
*/
public LdarSdkBuilder ldarPort(final int ldarPort) {
this.ldarPort = ldarPort;
return this;
}
/**
* Build ldar sdk object with its authentication host.
*
* @param authHost
* @return
*/
@Nonnull
public LdarSdkBuilder authHost(final String authHost) {
this.authHost = authHost;
return this;
}
/**
* Build ldar sdk object with its authentication port.
*
* @param authPort
* @return
*/
@Nonnull
public LdarSdkBuilder authPort(final int authPort) {
this.authPort = authPort;
return this;
}
/**
* Build ldar sdk object with the accessing service name.
*
* @param serviceName the name of your backend service. This name is used for service
* authentication in the ldar backend system
* @return the ldar sdk builder object
*/
@Nonnull
public LdarSdkBuilder serviceName(final String serviceName) {
this.serviceName = serviceName;
return this;
}
/**
* Build ldar sdk object with service password for authentication.
*
* @param servicePassword
* @return
*/
@Nonnull
public LdarSdkBuilder servicePassword(final String servicePassword) {
this.servicePassword = servicePassword;
return this;
}
/**
* Build a sync ldar manager.
*/
public LdarClient build() {
return new LdarClient(ldarHost, ldarPort, authHost, authPort, serviceName, servicePassword);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy