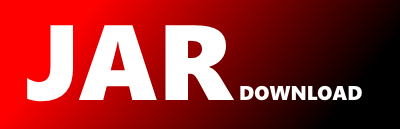
commonMain.co.touchlab.stately.collections.IsoArrayDeque.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stately-iso-collections-js Show documentation
Show all versions of stately-iso-collections-js Show documentation
Multithreaded Kotlin Multiplatform Utilities
package co.touchlab.stately.collections
import co.touchlab.stately.isolate.StateHolder
import co.touchlab.stately.isolate.StateRunner
import co.touchlab.stately.isolate.createState
open class IsoArrayDeque
internal constructor(stateHolder: StateHolder>) :
IsoMutableList(stateHolder) {
constructor(stateRunner: StateRunner? = null, producer: () -> ArrayDeque = { ArrayDeque() }) : this(createState(stateRunner, producer))
/**
* Returns the first element, or throws [NoSuchElementException] if this deque is empty.
*/
public fun first(): E = asAccess { it.first() }
/**
* Returns the first element, or `null` if this deque is empty.
*/
public fun firstOrNull(): E? = asAccess { it.firstOrNull() }
/**
* Returns the last element, or throws [NoSuchElementException] if this deque is empty.
*/
public fun last(): E = asAccess { it.last() }
/**
* Returns the last element, or `null` if this deque is empty.
*/
public fun lastOrNull(): E? = asAccess { it.lastOrNull() }
/**
* Prepends the specified [element] to this deque.
*/
public fun addFirst(element: E) = asAccess { it.addFirst(element) }
/**
* Appends the specified [element] to this deque.
*/
public fun addLast(element: E) = asAccess { it.addLast(element) }
/**
* Removes the first element from this deque and returns that removed element, or throws [NoSuchElementException] if this deque is empty.
*/
public fun removeFirst(): E = asAccess { it.removeFirst() }
/**
* Removes the first element from this deque and returns that removed element, or returns `null` if this deque is empty.
*/
public fun removeFirstOrNull(): E? = asAccess { it.removeFirstOrNull() }
/**
* Removes the last element from this deque and returns that removed element, or throws [NoSuchElementException] if this deque is empty.
*/
public fun removeLast(): E = asAccess { it.removeLast() }
/**
* Removes the last element from this deque and returns that removed element, or returns `null` if this deque is empty.
*/
public fun removeLastOrNull(): E? = asAccess { it.removeLastOrNull() }
private inline fun asAccess(crossinline block: (ArrayDeque) -> R): R =
access { block(it as ArrayDeque) }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy