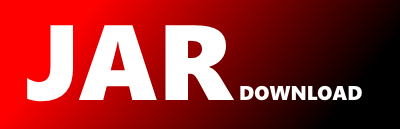
co.unruly.matchers.OptionalMatchers Maven / Gradle / Ivy
package co.unruly.matchers;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
import java.util.Optional;
import java.util.OptionalDouble;
import java.util.OptionalInt;
import java.util.OptionalLong;
public class OptionalMatchers {
/**
* Matches an empty Optional.
*/
public static Matcher> empty() {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Optional item) {
return !item.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("An empty Optional");
}
};
}
/**
* Matches a non empty Optional with the given content
*
* @param content Expected contents of the Optional
* @param The type of the Optional's content
*/
public static Matcher> contains(T content) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Optional item) {
return item.map(content::equals).orElse(false);
}
@Override
public void describeTo(Description description) {
description.appendText(Optional.of(content).toString());
}
};
}
/**
* Matches a non empty Optional with content matching the given matcher
*
* @param matcher To match against the Optional's content
* @param The type of the Optional's content
* @param The type matched by the matcher, a subtype of T
*/
public static Matcher> contains(Matcher matcher) {
return new TypeSafeMatcher>() {
@Override
protected boolean matchesSafely(Optional item) {
return item.map(matcher::matches).orElse(false);
}
@Override
public void describeTo(Description description) {
description.appendText("Optional with an item that matches" + matcher);
}
};
}
/**
* Matches an empty OptionalInt.
*/
public static Matcher emptyInt() {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalInt item) {
return !item.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("An empty OptionalInt");
}
};
}
/**
* Matches a non empty OptionalInt with the given content
*
* @param content Expected contents of the Optional
*/
public static Matcher containsInt(int content) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalInt item) {
return item.isPresent() && item.getAsInt() == content;
}
@Override
public void describeTo(Description description) {
description.appendText(Optional.of(content).toString());
}
};
}
/**
* Matches a non empty OptionalInt with content matching the given matcher
*
* @param matcher To match against the OptionalInt's content
*/
public static Matcher containsInt(Matcher matcher) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalInt item) {
return item.isPresent() && matcher.matches(item.getAsInt());
}
@Override
public void describeTo(Description description) {
description.appendText("OptionalInt with an item that matches" + matcher);
}
};
}
/**
* Matches an empty OptionalLong.
*/
public static Matcher emptyLong() {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalLong item) {
return !item.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("An empty OptionalLong");
}
};
}
/**
* Matches a non empty OptionalLong with the given content
*
* @param content Expected contents of the Optional
*/
public static Matcher containsLong(long content) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalLong item) {
return item.isPresent() && item.getAsLong() == content;
}
@Override
public void describeTo(Description description) {
description.appendText(Optional.of(content).toString());
}
};
}
/**
* Matches a non empty OptionalLong with content matching the given matcher
*
* @param matcher To match against the OptionalLong's content
*/
public static Matcher containsLong(Matcher matcher) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalLong item) {
return item.isPresent() && matcher.matches(item.getAsLong());
}
@Override
public void describeTo(Description description) {
description.appendText("OptionalLong with an item that matches" + matcher);
}
};
}
/**
* Matches an empty OptionalDouble.
*/
public static Matcher emptyDouble() {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalDouble item) {
return !item.isPresent();
}
@Override
public void describeTo(Description description) {
description.appendText("An empty OptionalDouble");
}
};
}
/**
* Matches a non empty OptionalDouble with the given content
*
* @param content Expected contents of the Optional
*/
public static Matcher containsDouble(double content) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalDouble item) {
return item.isPresent() && item.getAsDouble() == content;
}
@Override
public void describeTo(Description description) {
description.appendText(Optional.of(content).toString());
}
};
}
/**
* Matches a non empty OptionalDouble with content matching the given matcher
*
* @param matcher To match against the OptionalDouble's content
*/
public static Matcher containsDouble(Matcher matcher) {
return new TypeSafeMatcher() {
@Override
protected boolean matchesSafely(OptionalDouble item) {
return item.isPresent() && matcher.matches(item.getAsDouble());
}
@Override
public void describeTo(Description description) {
description.appendText("OptionalDouble with an item that matches" + matcher);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy