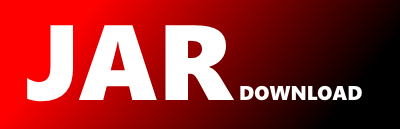
co.verisoft.fw.pages.WebBasePageJS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Selenium4-Junit5 Show documentation
Show all versions of Selenium4-Junit5 Show documentation
VeriSoft framework for testing web and mobile applications. Selenium Module
/*
* (C) Copyright 2022 VeriSoft (http://www.verisoft.co)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package co.verisoft.fw.pages;
import co.verisoft.fw.selenium.drivers.VerisoftDriverManager;
import co.verisoft.fw.utils.Property;
import co.verisoft.fw.utils.Waits;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import java.util.Objects;
import java.util.Set;
/**
* Default interface the main goal is to concentrate all mainly Java Script functions
*
* @author David Yehezkel
* 29 Mar 2020
*/
public interface WebBasePageJS {
int timeout = new Property().getIntProperty("selenium.wait.timeout");
/**
* Main function that performs the actual click on a web element using JavaScript.
* This is the core implementation, and all other overloaded methods delegate to it.
*
* @param driver the WebDriver instance to use for executing the JavaScript
* @param element the WebElement to be clicked
* @throws NullPointerException if the provided driver is null
*/
default void clickOnElementByJS(WebDriver driver, WebElement element) {
((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript("arguments[0].click();", element);
}
/**
* This method is a convenient overload that uses the default WebDriver from
* {@code VerisoftDriverManager}.
*/
default void clickOnElementByJS(WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver();
clickOnElementByJS(driver, element);
}
/**
* This method allows specifying a driver by its name.
* in scenarios where multiple drivers are managed dynamically.
*/
default void clickOnElementByJS(String driverName, WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
clickOnElementByJS(driver, element);
}
/**
* Main function that performs the actual mouse hover action on a web element using JavaScript.
* This is the core implementation, and all other overloaded methods delegate to it.
*
* @param driver the WebDriver instance to use for executing the JavaScript
* @param element the WebElement on which to perform the mouse hover action
* @throws NullPointerException if the provided driver is null
*/
default void mouseHoverByJS(WebDriver driver, WebElement element) {
String mouseOverScript =
"if(document.createEvent){" +
"var evObj = document.createEvent('MouseEvents');" +
"evObj.initEvent('mouseover',true, false); " +
"arguments[0].dispatchEvent(evObj);} " +
"else if(document.createEventObject) { " +
"arguments[0].fireEvent('onmouseover');}";
((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript(mouseOverScript, element);
}
/**
* Simulates a mouse hover action on a web element using the default driver.
* This method is a convenient overload that uses the default WebDriver from
* {@code VerisoftDriverManager}.
*/
default void mouseHoverByJS(WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver();
mouseHoverByJS(driver, element);
}
/**
* Simulates a mouse hover action on a web element using a WebDriver identified by its name.
* This method allows specifying a driver by its name.
* in scenarios where multiple drivers are managed dynamically.
*/
default void mouseHoverByJS(String driverName, WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
mouseHoverByJS(driver, element);
}
/**
* Main function that retrieves the RGB color of a pseudo-element from the WebElement
* using JavaScript.
* This is the core implementation, and all other overloaded methods delegate to it.
*
* @param driver the WebDriver instance to use for executing the JavaScript
* @param element Webelement element
* @return RGB(Red, Green, Blue)
* @throws NullPointerException if the provided driver is null
*/
default String getBeforePseudoCode(WebDriver driver, WebElement element) {
return ((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript("return window.getComputedStyle(arguments[0], ':before')" +
".getPropertyValue('background-color');"
, element).toString();
}
/**
* Retrieves the RGB color of a pseudo-element from the WebElement using the default driver.
* This method is a convenient overload that uses the default WebDriver from
* {@code VerisoftDriverManager}.
*/
default String getBeforePseudoCode(WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver();
return getBeforePseudoCode(driver, element);
}
/**
* Retrieves the RGB color of a pseudo-element from the WebElement using a WebDriver
* identified by its name.
* This method allows specifying a driver by its name.
* in scenarios where multiple drivers are managed dynamically.
*/
default String getBeforePseudoCode(String driverName, WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
return getBeforePseudoCode(driver, element);
}
/**
* Provides overloaded methods to open a new browser tab using JavaScript.
* These methods offer flexibility in how the WebDriver is specified:
*/
default void openNewTab(WebDriver driver) {
((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript("window.open()");
}
/**
* Opens a new browser tab using the default WebDriver.
*/
default void openNewTab() {
WebDriver driver = VerisoftDriverManager.getDriver();
openNewTab(driver);
}
/**
* Opens a new browser tab using a WebDriver identified by its name.
*/
default void openNewTab(String driverName) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
openNewTab(driver);
}
/**
* Retrieves the shadow root element of a WebElement using JavaScript.
* This method accesses the shadow DOM of a given WebElement and returns the
* This is the core implementation, and all other overloaded methods delegate to it.
*
* @param driver the WebDriver instance to use for executing the JavaScript
* @param rootElement Root shadow element
* @return return Shadow root element
*/
default WebElement getShadowRoot(WebDriver driver, WebElement rootElement) {
return (WebElement) ((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript("return arguments[0].shadowRoot", rootElement);
}
/**
* Initializes the default WebDriver using the {@code VerisoftDriverManager}
* and delegates the task to retrieves the shadow root element
* of a WebElement using JavaScript.
*/
default WebElement getShadowRoot(WebElement rootElement) {
WebDriver driver = VerisoftDriverManager.getDriver();
return getShadowRoot(driver, rootElement);
}
/**
* Initializes a WebDriver based on the provided driver name (String)
* and delegates the task to retrieves the shadow root element
* of a WebElement using JavaScript.
*/
default WebElement getShadowRoot(String driverName, WebElement rootElement) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
return getShadowRoot(driver, rootElement);
}
/**
* This method accesses the shadow DOM of a given WebElement and returns the
* get css of ::before attribute
*
* @param driver the WebDriver instance to use for executing the JavaScript
* @param element Webelement element
* @return RGB(red, green, blue, blur)
*/
default String getColorOfBeforeCssAtter(WebDriver driver, WebElement element) {
return ((JavascriptExecutor) Objects.requireNonNull(driver))
.executeScript("return window.getComputedStyle(arguments[0], ':before')" +
".getPropertyValue('background-color');",
element).toString();
}
/**
* Initializes the default WebDriver using the {@code VerisoftDriverManager}
* and delegates the task to given WebElement and returns the
* get css of ::before attribute
*/
default String getColorOfBeforeCssAtter(WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver();
return getColorOfBeforeCssAtter(driver, element);
}
/**
* Initializes a WebDriver based on the provided driver name (String)
* and delegates the task to given WebElement and returns the
* get css of ::before attribute
*/
default String getColorOfBeforeCssAtter(String driverName, WebElement element) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
return getColorOfBeforeCssAtter(driver, element);
}
/**
* Opens a new browser tab and switches to it using the provided WebDriver instance.
*
* @param driver the WebDriver instance used to perform the action
*/
default void openNewTabAndSwitchToIt(WebDriver driver) {
Set windows = driver.getWindowHandles();
((JavascriptExecutor) driver).executeScript("window.open();");
Waits.numberOfWindowsToBeAndSwitchTo(driver, timeout,
windows.size() + 1, windows.size());
}
/**
* Opens a new browser tab and switches to it using the default WebDriver instance.
*/
default void openNewTabAndSwitchToIt() {
WebDriver driver = VerisoftDriverManager.getDriver();
openNewTabAndSwitchToIt(driver);
}
/**
* Opens a new browser tab and switches to it using the specified WebDriver instance name.
*/
default void openNewTabAndSwitchToIt(String driverName) {
WebDriver driver = VerisoftDriverManager.getDriver(driverName);
openNewTabAndSwitchToIt(driver);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy