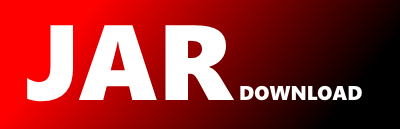
co.verisoft.fw.xray.XrayJsonStepDefObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of test-api Show documentation
Show all versions of test-api Show documentation
VeriSoft framework for testing web and mobile applications. junit 5 Module
The newest version!
package co.verisoft.fw.xray;
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* See the NOTICE file distributed with this work for additional
* information regarding copyright ownership.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import co.verisoft.fw.utils.Builder;
import co.verisoft.fw.utils.JsonObject;
import lombok.ToString;
import org.jetbrains.annotations.Nullable;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Representation of Xray object - Step Definition. This class follows the builder design pattern
*
*
From Xray documentation:
* "step def" object - step definition
* This object allows you to define the step fields for manual tests. Custom test step fields are also supported.
*
* @author Nir Gallner
* @see
* Using Xray JSON format to import execution results - Step Definition
* @since 0.0.2 (Jan 2022)
*/
@ToString
public class XrayJsonStepDefObject extends XrayJsonFormat implements JsonObject {
private final Map fields;
public @Nullable String getAction() {
return (String) fields.get("action");
}
public @Nullable String getData() {
return (String) fields.get("data");
}
public @Nullable String getResult() {
return (String) fields.get("result");
}
public @Nullable List getCustomFields() {
//noinspection unchecked
return (List) fields.get("customFields");
}
public @Nullable XrayJsonCustomFieldObject getCustomField(String id) {
List fields = this.getCustomFields();
return fields.stream()
.filter(custField -> custField.getId().equalsIgnoreCase(id))
.findAny()
.orElse(null);
}
private XrayJsonStepDefObject(XrayJsonStepDefObjectBuilder builder) {
this.fields = builder.fields;
}
@SuppressWarnings("unchecked")
@Override
public JSONObject asJsonObject() {
JSONObject obj = new JSONObject();
if (this.getAction() != null)
obj.put("action", this.getAction());
if (this.getData() != null)
obj.put("data", this.getData());
if (this.getResult() != null)
obj.put("result", this.getResult());
if (this.getCustomFields() != null) {
JSONArray arr = new JSONArray();
for (XrayJsonCustomFieldObject custField : getCustomFields())
arr.add(custField.asJsonObject());
if (!arr.isEmpty()) {
obj.put("customFields", arr);
}
}
return obj;
}
@Override
public String asString() {
return this.asJsonObject().toJSONString();
}
/**
* Builder class for XrayInfoObject
*
* @author Nir Gallner
* @since 0.0.2 (Jan 2022)
*/
@ToString
public static class XrayJsonStepDefObjectBuilder implements Builder {
private final Map fields;
public XrayJsonStepDefObjectBuilder(XrayJsonStepDefObject obj) {
this.fields = obj.fields;
}
public XrayJsonStepDefObjectBuilder() {
fields = new HashMap<>();
}
public XrayJsonStepDefObjectBuilder action(String action) {
fields.put("action", action);
return this;
}
public XrayJsonStepDefObjectBuilder data(String data) {
fields.put("data", data);
return this;
}
public XrayJsonStepDefObjectBuilder result(String result) {
fields.put("result", result);
return this;
}
public XrayJsonStepDefObjectBuilder customFields(List customFields) {
fields.put("customFields", customFields);
return this;
}
public XrayJsonStepDefObjectBuilder customField(XrayJsonCustomFieldObject customField) {
if (fields.get("customFields") != null) {
List p = ((List) fields.get("customFields"));
p.add(customField);
fields.put("customFields", p);
} else {
List p = new ArrayList<>();
p.add(customField);
this.customFields(p);
}
return this;
}
public XrayJsonStepDefObject build() {
XrayJsonStepDefObject info = new XrayJsonStepDefObject(this);
validateXrayStepDefObject(info);
return info;
}
private void validateXrayStepDefObject(XrayJsonStepDefObject obj) {
// TOOD: some validation code here
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy