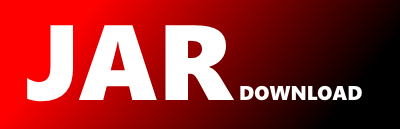
src.java.org.jnativehook.mouse.NativeMouseWheelEvent Maven / Gradle / Ivy
Show all versions of jnativehook Show documentation
/* JNativeHook: Global keyboard and mouse hooking for Java.
* Copyright (C) 2006-2015 Alexander Barker. All Rights Received.
* https://github.com/kwhat/jnativehook/
*
* JNativeHook is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published
* by the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JNativeHook is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package org.jnativehook.mouse;
// Imports.
import org.jnativehook.GlobalScreen;
/**
* An event which indicates that the mouse wheel was rotated on the system.
* This event is not limited to a particular component's scope or visibility.
*
*
* A NativeMouseWheelEvent
object is passed to every
* NativeMouseWheelListener
object which is registered to receive
* mouse wheel events using the
* {@link GlobalScreen#addNativeMouseWheelListener(NativeMouseWheelListener)}
* method. The GlobalScreen
object then dispatches a
* NativeMouseWheelEvent
to each listener.
*
*
* Platforms offer customization of the amount of scrolling that should take
* place when the mouse wheel is moved. The two most common settings are to
* scroll a certain number of "units" (commonly lines of text in a text-based
* component) or an entire "block" (similar to page-up/page-down). The
* NativeMouseWheelEvent
offers methods for conforming to the
* underlying platform settings. These platform settings can be changed at any
* time by the user. NativeMouseWheelEvents
reflect the most recent
* settings.
*
* @author Alexander Barker ([email protected])
* @version 2.0
* @since 1.1
*
* @see GlobalScreen
* @see NativeMouseWheelListener
*/
public class NativeMouseWheelEvent extends NativeMouseEvent {
/** The Constant serialVersionUID. */
private static final long serialVersionUID = -183110294708745910L;
/**
* Constant representing scrolling by "units" (like scrolling with the
* arrow keys).
*/
public static final int WHEEL_UNIT_SCROLL = 1;
/**
* Constant representing scrolling by a "block" (like scrolling with
* page-up, page-down keys).
*/
public static final int WHEEL_BLOCK_SCROLL = 2;
/**
* Only valid for scrollType WHEEL_UNIT_SCROLL. Indicates number of units
* that should be scrolled per click of mouse wheel rotation, based on
* platform settings.
*
* @see #getScrollAmount
* @see #getScrollType
*/
private int scrollAmount;
/**
* Indicates what sort of scrolling should take place in response to this
* event, based on platform settings. Legal values are:
*
* - WHEEL_UNIT_SCROLL
*
- WHEEL_BLOCK_SCROLL
*
*
* @see #getScrollType
*/
private int scrollType;
/**
* Indicates how far the mouse wheel was rotated.
*
* @see #getWheelRotation
*/
private int wheelRotation;
/**
* Instantiates a new NativeMouseWheelEvent
object.
*
* @param id an integer that identifies the native event type.
* @param when a long integer that gives the time the event occurred.
* @param modifiers a modifier mask describing the modifier keys and mouse
* buttons active for the event.
* NativeInputEvent _MASK
modifiers should be used as they are
* not compatible with the extended _DOWN_MASK or the old _MASK
* InputEvent
modifiers.
* @param x the x coordinate of the native pointer.
* @param y the y coordinate of the native pointer.
* @param clickCount the number of button clicks associated with this event.
* @param scrollType the type of scrolling which should take place in
* response to this event; valid values are WHEEL_UNIT_SCROLL
* and WHEEL_BLOCK_SCROLL
.
* @param scrollAmount for scrollType WHEEL_UNIT_SCROLL
, the
* number of units to be scrolled.
* @param wheelRotation the amount that the mouse wheel was rotated (the
* number of "clicks")
*
* @see NativeMouseEvent#NativeMouseEvent(int, long, int, int, int, int)
*/
public NativeMouseWheelEvent(int id, long when, int modifiers, int x, int y, int clickCount, int scrollType, int scrollAmount, int wheelRotation) {
super(id, when, modifiers, x, y, clickCount);
this.scrollType = scrollType;
this.scrollAmount = scrollAmount;
this.wheelRotation = wheelRotation;
}
/**
* Returns the number of units that should be scrolled per
* click of mouse wheel rotation.
* Only valid if getScrollType
returns
* NativeMouseWheelEvent.WHEEL_UNIT_SCROLL
*
* @return number of units to scroll, or an undefined value if
* getScrollType
returns
* NativeMouseWheelEvent.WHEEL_BLOCK_SCROLL
* @see #getScrollType
*/
public int getScrollAmount() {
return scrollAmount;
}
/**
* Returns the type of scrolling that should take place in response to this
* event. This is determined by the native platform. Legal values are:
*
* - MouseWheelEvent.WHEEL_UNIT_SCROLL
*
- MouseWheelEvent.WHEEL_BLOCK_SCROLL
*
*
* @return either NativeMouseWheelEvent.WHEEL_UNIT_SCROLL or
* NativeMouseWheelEvent.WHEEL_BLOCK_SCROLL, depending on the configuration of
* the native platform.
* @see java.awt.Adjustable#getUnitIncrement
* @see java.awt.Adjustable#getBlockIncrement
* @see javax.swing.Scrollable#getScrollableUnitIncrement
* @see javax.swing.Scrollable#getScrollableBlockIncrement
*/
public int getScrollType() {
return scrollType;
}
/**
* Returns the number of "clicks" the mouse wheel was rotated.
*
* @return negative values if the mouse wheel was rotated up/away from
* the user, and positive values if the mouse wheel was rotated down/
* toward(s) the user.
*/
public int getWheelRotation() {
return wheelRotation;
}
/**
* Returns a parameter string identifying the native event.
* This method is useful for event-logging and debugging.
*
* @return a string identifying the native event and its attributes.
*/
@Override
public String paramString() {
StringBuilder param = new StringBuilder(super.paramString());
param.append(",scrollType=");
switch(getScrollType()) {
case WHEEL_UNIT_SCROLL:
param.append("WHEEL_UNIT_SCROLL");
break;
case WHEEL_BLOCK_SCROLL:
param.append("WHEEL_BLOCK_SCROLL");
break;
default:
param.append("unknown scroll type");
break;
}
param.append(",scrollAmount=");
param.append(getScrollAmount());
param.append(",wheelRotation=");
param.append(getWheelRotation());
return param.toString();
}
}