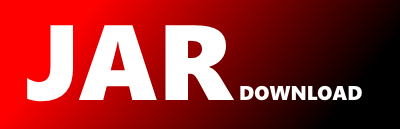
com.zoi7.component.web.handler.ControllerExceptionHandler Maven / Gradle / Ivy
package com.zoi7.component.web.handler;
import com.zoi7.component.core.exception.*;
import com.zoi7.component.web.base.BaseController;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestControllerAdvice;
/**
*
* Controller异常处理
*
* 处理 Controller 抛出 或 未处理的异常
*
* @author yjy
* 2018-07-06 10:24
*/
@RestControllerAdvice
public class ControllerExceptionHandler extends BaseController {
private static final Logger ARGUMENTS_LOG = LoggerFactory.getLogger(ArgumentsInvalidException.class);
private static final Logger NOT_FOUND_LOG = LoggerFactory.getLogger(NotFoundException.class);
private static final Logger OPER_FAILED_LOG = LoggerFactory.getLogger(OperationFailedException.class);
private static final Logger SERVICE_LOG = LoggerFactory.getLogger(ServiceException.class);
private static final Logger NOTLOGIN_LOG = LoggerFactory.getLogger(NotLoginException.class);
private static final Logger EXCEPTION_LOG = LoggerFactory.getLogger(Exception.class);
@Autowired
private SimplePage simplePage;
/**
* 参数异常
* @return 错误信息
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String argumentsHandler(ArgumentsInvalidException e) {
ARGUMENTS_LOG.warn("参数错误", e);
return response(e.getMessage(), 400, "参数错误");
}
/**
* 资源找不到异常
* @return 错误提示
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String notFoundHandler(NotFoundException e) {
NOT_FOUND_LOG.warn("404", e);
return response(e.getMessage(), 404, "NOT FOUND");
}
/**
* 操作失败异常
* @return 错误提示
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String failedHandler(OperationFailedException e) {
OPER_FAILED_LOG.warn("failed", e);
return response(e.getMessage(), 1006, "操作失败");
}
/**
* 服务调用异常
* @param e 异常
* @return 错误信息
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String serviceHandler(ServiceException e) {
SERVICE_LOG.warn("service unavailable", e);
return response(e.getMessage(), 503, "503 Service Unavailable");
}
/**
* 处理未登入异常
* @param e 异常
* @return 错误信息
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String loginHandler(NotLoginException e) {
NOTLOGIN_LOG.warn("game not login", e);
return response(e.getMessage(), 1001, "未登入");
}
/**
* 全局异常
* @param e 异常
* @return 错误信息
*/
@ExceptionHandler
@ResponseStatus(HttpStatus.OK)
public String exceptionHandler(Exception e) {
EXCEPTION_LOG.error("请求出错", e);
return response("System error", 500, "未知错误");
}
/**
* 根据请求类型返回信息
* @param info 错误信息
* @param code 错误码
* @param title 错误页面标题
*/
private String response(String info, Integer code, String title) {
String requestType = request.getHeader("X-Requested-With");
// if 是ajax请求则返回json串
if (requestType != null && requestType.equals("XMLHttpRequest")) {
return ajaxToJson(code, info);
}
return simplePage.getPage(title, info);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy