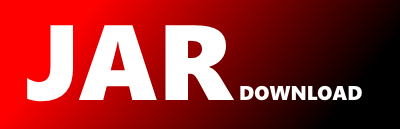
com.eightkdata.mongowp.mongoserver.api.safe.tools.bson.BsonDocumentBuilder Maven / Gradle / Ivy
The newest version!
package com.eightkdata.mongowp.mongoserver.api.safe.tools.bson;
import com.eightkdata.mongowp.mongoserver.pojos.OpTime;
import com.google.common.base.Function;
import com.google.common.base.Preconditions;
import com.google.common.net.HostAndPort;
import java.util.Map.Entry;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.bson.*;
import org.bson.types.ObjectId;
import org.threeten.bp.Instant;
/**
*
*/
public class BsonDocumentBuilder {
private final BsonDocument doc;
private boolean built;
public BsonDocumentBuilder() {
this.doc = new BsonDocument();
built = false;
}
public BsonDocumentBuilder(BsonDocument doc) {
this.doc = doc;
built = false;
}
public boolean containsField(@Nonnull BsonField field) {
return doc.containsKey(field.getFieldName());
}
public BsonDocumentBuilder copy(@Nonnull BsonDocument otherDoc) {
for (Entry entrySet : otherDoc.entrySet()) {
doc.append(entrySet.getKey(), entrySet.getValue());
}
return this;
}
public BsonDocumentBuilder appendUnsafe(String fieldName, @Nullable BsonValue value) {
Preconditions.checkState(!built);
if (value == null) {
doc.append(fieldName, BsonNull.VALUE);
return this;
}
doc.append(fieldName, value);
return this;
}
public BsonDocumentBuilder append(BsonField field, @Nullable BsonValue value) {
Preconditions.checkState(!built);
if (value == null) {
doc.append(field.getFieldName(), BsonNull.VALUE);
return this;
}
doc.append(field.getFieldName(), value);
return this;
}
public BsonDocumentBuilder append(BsonField field, T value, Function translator) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), translator.apply(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, boolean value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), BsonBoolean.valueOf(value));
return this;
}
public BsonDocumentBuilder appendNumber(BsonField extends Number> field, Number value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), toBsonNumber(value));
return this;
}
private BsonNumber toBsonNumber(Number number) {
if (number instanceof Double || number instanceof Float) {
return new BsonDouble(number.doubleValue());
}
if (number instanceof Long) {
long longValue = number.longValue();
if (longValue <= Integer.MAX_VALUE && longValue >= Integer.MAX_VALUE) {
return new BsonInt32((int) longValue);
}
return new BsonInt64(longValue);
}
if (number instanceof Integer || number instanceof Byte || number instanceof Short) {
return new BsonInt32(number.intValue());
}
throw new IllegalArgumentException("Numbers of class " + number.getClass() + " are not supported");
}
public BsonDocumentBuilder appendNumber(BsonField extends Number> field, int value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), new BsonInt32(value));
return this;
}
public BsonDocumentBuilder appendNumber(BsonField extends Number> field, long value) {
Preconditions.checkState(!built);
if (value < Integer.MAX_VALUE && value > Integer.MIN_VALUE) {
doc.append(field.getFieldName(), new BsonInt32((int) value));
}
else {
doc.append(field.getFieldName(), new BsonInt64(value));
}
return this;
}
public BsonDocumentBuilder append(BsonField field, int value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), new BsonInt32(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, long value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), new BsonInt64(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, String value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), new BsonString(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, double value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), new BsonDouble(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, Instant value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), new BsonDateTime(value.toEpochMilli()));
return this;
}
/**
*
* @param field
* @param value millis since Epoch
* @return
*/
public BsonDocumentBuilder appendInstant(BsonField field, long value) {
Preconditions.checkState(!built);
doc.append(field.getFieldName(), new BsonDateTime(value));
return this;
}
public BsonDocumentBuilder append(BsonField field, OpTime value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(
field.getFieldName(),
value.asBsonTimestamp()
);
return this;
}
public BsonDocumentBuilder append(BsonField field, BsonArray value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), value);
return this;
}
public BsonDocumentBuilder append(BsonField field, BsonDocument value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), value);
return this;
}
public BsonDocumentBuilder append(BsonField field, BsonDocumentBuilder value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), value.build());
return this;
}
public BsonDocumentBuilder append(BsonField field, HostAndPort value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), new BsonString(value.toString()));
return this;
}
public BsonDocumentBuilder append(BsonField field, ObjectId value) {
Preconditions.checkState(!built);
if (value == null) {
return appendNull(field);
}
doc.append(field.getFieldName(), new BsonObjectId(value));
return this;
}
public BsonDocumentBuilder appendNull(BsonField> field) {
doc.append(field.getFieldName(), BsonNull.VALUE);
return this;
}
public BsonDocument build() {
built = true;
return doc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy