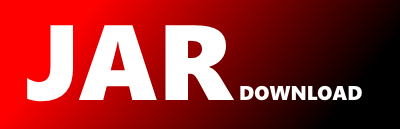
com.nls.util.CollectionDifference Maven / Gradle / Ivy
The newest version!
package com.nls.util;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
public class CollectionDifference {
private final Collection oldCollection;
private final Collection newCollection;
private final Function comparisonMapper;
public CollectionDifference(Collection oldCollection, Collection newCollection) {
this(oldCollection, newCollection, Function.identity());
}
public CollectionDifference(Collection oldCollection, Collection newCollection, Function comparisonMapper) {
this.oldCollection = oldCollection;
this.newCollection = newCollection;
this.comparisonMapper = comparisonMapper;
}
public List added() {
Set> newValues = newCollection.stream().map(comparisonMapper).collect(Collectors.toSet());
Set> oldValues = oldCollection.stream().map(comparisonMapper).collect(Collectors.toSet());
newValues.removeAll(oldValues);
return newCollection.stream().filter(o -> newValues.contains(comparisonMapper.apply(o))).collect(Collectors.toList());
}
public List removed() {
Set> newValues = newCollection.stream().map(comparisonMapper).collect(Collectors.toSet());
Set> oldValues = oldCollection.stream().map(comparisonMapper).collect(Collectors.toSet());
oldValues.removeAll(newValues);
return oldCollection.stream().filter(o -> oldValues.contains(comparisonMapper.apply(o))).collect(Collectors.toList());
}
public > C addToCollection(C collection) {
collection.addAll(added());
return collection;
}
public > C removeFromCollection(C collection) {
collection.removeAll(removed());
return collection;
}
public > C updateCollection(C collection) {
addToCollection(collection);
removeFromCollection(collection);
return collection;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy