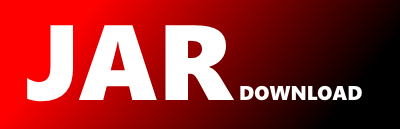
com.abiquo.model.rest.RESTLink Maven / Gradle / Ivy
/**
* Copyright (C) 2008 Abiquo Holdings S.L.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.abiquo.model.rest;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
import com.abiquo.model.enumerator.DiskControllerType;
import com.fasterxml.jackson.annotation.JsonIgnore;
/**
* extension to use the link fields as attributes
*/
@XmlRootElement(name = "link")
public class RESTLink
{
@XmlAttribute
private String diskAllocation;
@XmlAttribute
private String diskController;
@XmlAttribute
private DiskControllerType diskControllerType;
@XmlAttribute
private String diskLabel;
@XmlAttribute
private String length;
@XmlAttribute
private String title;
@XmlAttribute
private String hreflang;
@XmlAttribute
private String rel;
@XmlAttribute
private String type;
@XmlAttribute
private String href;
@XmlAttribute
private String name;
@XmlAttribute
private String uuid;
@XmlAttribute
private String providerId;
public RESTLink()
{
}
public RESTLink(final String rel, final String href)
{
this.rel = rel;
this.href = href;
}
public RESTLink(final String rel, final String href, final String type, final String title)
{
this.rel = rel;
this.href = href;
this.type = type;
this.title = title;
}
@XmlTransient
public String getDiskAllocation()
{
return diskAllocation;
}
public void setDiskAllocation(final String diskAllocation)
{
this.diskAllocation = diskAllocation;
}
@XmlTransient
public String getDiskController()
{
return diskController;
}
public void setDiskController(final String diskController)
{
this.diskController = diskController;
}
@XmlTransient
public DiskControllerType getDiskControllerType()
{
return diskControllerType;
}
public void setDiskControllerType(final DiskControllerType diskControllerType)
{
this.diskControllerType = diskControllerType;
}
@XmlTransient
public String getDiskLabel()
{
return diskLabel;
}
public void setDiskLabel(final String diskLabel)
{
this.diskLabel = diskLabel;
}
@XmlTransient
public String getLength()
{
return length;
}
public void setLength(final String length)
{
this.length = length;
}
@XmlTransient
public String getTitle()
{
return title;
}
public void setTitle(final String title)
{
this.title = title;
}
@XmlTransient
public String getHreflang()
{
return hreflang;
}
public void setHreflang(final String hreflang)
{
this.hreflang = hreflang;
}
@XmlTransient
public String getRel()
{
return rel;
}
public void setRel(final String rel)
{
this.rel = rel;
}
@XmlTransient
public String getType()
{
return type;
}
public void setType(final String type)
{
this.type = type;
}
@XmlTransient
public String getHref()
{
return href;
}
public void setHref(final String href)
{
this.href = href;
}
@XmlTransient
@JsonIgnore
public Integer getId()
{
// Maybe URIs don't have a trailing slash
String id = href.substring(href.lastIndexOf("/") + 1,
href.endsWith("/") ? href.length() - 1 : href.length());
try
{
return Integer.parseInt(id);
}
catch (NumberFormatException e)
{
return null;
}
}
@XmlTransient
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
@XmlTransient
public String getUuid()
{
return uuid;
}
@XmlTransient
public String getProviderId()
{
return providerId;
}
public void setUuid(String uuid)
{
this.uuid = uuid;
}
public void setProviderId(String providerId)
{
this.providerId = providerId;
}
@Override
public String toString()
{
StringBuilder sb = new StringBuilder();
sb.append("").toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy