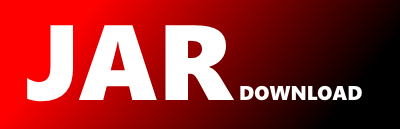
com.abissell.javautil.rusty.Opt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-util Show documentation
Show all versions of java-util Show documentation
Java utilities, some incorporating modern language features
The newest version!
/*
* Copyright 2023 Andrew Bissell. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.abissell.javautil.rusty;
import java.util.NoSuchElementException;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
public sealed interface Opt permits Some, None {
default void ifPresent(Consumer super T> consumer) {
switch (this) {
case Some(T t) -> consumer.accept(t);
case None() -> {
}
}
}
default T orElse(T ifAbsent) {
return switch (this) {
case Some(T t) -> t;
case None() -> ifAbsent;
};
}
default T orElseGet(Supplier extends T> supplier) {
return switch (this) {
case Some(T t) -> t;
case None() -> supplier.get();
};
}
default Opt map(Function super T, ? extends U> mapper) {
return switch (this) {
case Some(T t) -> Opt.of(mapper.apply(t));
case None() -> Opt.none();
};
}
default boolean isSome() {
return this instanceof Some;
}
default boolean isNone() {
return !isSome();
}
default T get() throws NoSuchElementException {
return switch (this) {
case Some(T t) -> t;
case None() -> throw new NoSuchElementException();
};
}
static Opt none() {
return None.none();
}
static Opt of(T t) {
return new Some<>(t);
}
static Opt ofNullable(T t) {
return t == null ? None.none() : new Some<>(t);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy