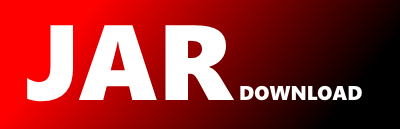
com.abubusoft.kripton.android.annotation.BindDao Maven / Gradle / Ivy
Show all versions of kripton-orm Show documentation
/*******************************************************************************
* Copyright 2015, 2016 Francesco Benincasa.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*******************************************************************************/
package com.abubusoft.kripton.android.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
*
* This annotation mark an interface for DAO (Data Access Object) associated to
* a specific bean associated with {@link #value()} attribute. For each defined
* methods in the associated interface will be generated an method which use SQL
* statement. The kind of SQL used in methods will depends from annotation used
* to mark method.
*
*
*
* Supported queries are:
*
*
* INSERT
: with {@link BindSqlInsert} annotation
* UPDATE
: with {@link BindSqlUpdate} annotation
* SELECT
: with {@link BindSqlSelect} annotation
* DELETE
: with {@link BindSqlDelete} annotation
*
*
*
* Referred {@link #value()} bean must be annotated with {@link BindSqlType}
* annotation.
*
*
* Attributes
*
* - value: bean class to associate with this dao
* definition.
*
*
* Usage
*
* Suppose we have the following DaoBean03 DAO interface
*
*
*
* @BindDao(Bean03.class)
* public interface DaoBean03 {
*
* @BindSqlSelect(where = "id=${id}")
* Bean03 selectOne(long id);
*
* @BindSqlDelete(where = "id=${id}")
* long deleteOne(long id);
* }
*
*
* And Bean03 definition:
*
*
*
* @BindType
* public class Bean03 {
*
* @Bind
* @BindColumn(columnType = ColumnType.PRIMARY_KEY)
* protected Long id;
*
* public Long getId() {
* return id;
* }
*
* public void setId(Long id) {
* this.id = id;
* }
*
* @Bind
* @BindColumn
* protected String text;
*
* public String getText() {
* return text;
* }
*
* public void setText(String text) {
* this.text = text;
* }
* }
*
*
*
* When Kripton Annotation Processor evaluate DaoBean03, it generate the
* following DAO implementations:
*
*
*
* public class DaoBean03Impl extends AbstractDao implements DaoBean03 {
* public DaoBean03Impl(BindDummy03DataSource dataSet) {
* super(dataSet);
* }
*
* @Override
* public Bean03 selectOne(long id) {
* // build where condition
* String[] args = { String.valueOf(id) };
*
* Logger.info(StringUtils.formatSQL("SELECT id, text FROM bean03 WHERE id='%s'"), (Object[]) args);
* Cursor cursor = database().rawQuery("SELECT id, text FROM bean03 WHERE id=?", args);
* Logger.info("Rows found: %s", cursor.getCount());
*
* Bean03 resultBean = null;
*
* if (cursor.moveToFirst()) {
*
* int index0 = cursor.getColumnIndex("id");
* int index1 = cursor.getColumnIndex("text");
*
* resultBean = new Bean03();
*
* if (!cursor.isNull(index0)) {
* resultBean.setId(cursor.getLong(index0));
* }
* if (!cursor.isNull(index1)) {
* resultBean.setText(cursor.getString(index1));
* }
*
* }
* cursor.close();
*
* return resultBean;
* }
*
* @Override
* public long deleteOne(long id) {
* String[] whereConditions = { String.valueOf(id) };
*
* Logger.info(StringUtils.formatSQL("DELETE bean03 WHERE id=%s"), (Object[]) whereConditions);
* int result = database().delete("bean03", "id=?", whereConditions);
* return result;
* }
* }
*
*
*
* DAO implementations contains null checks and javadoc for every method that
* are present in DAO interface and log for executed query (if in DataSource
* attribute log is set to true).
*
*
*
* @author Francesco Benincasa ([email protected])
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface BindDao {
/**
*
* Bean class to associate with this dao definition.
*
*
*
* Referred {@link #value()} bean must be annotated with {@link BindSqlType}
* annotation.
*
*
* @return class of assocaited bean
*/
Class> value();
}