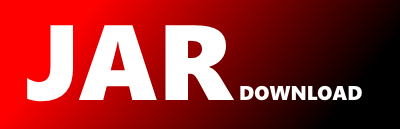
com.abubusoft.kripton.android.annotation.BindDataSourceOptions Maven / Gradle / Ivy
Show all versions of kripton-orm Show documentation
/*******************************************************************************
* Copyright 2015, 2017 Francesco Benincasa ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*******************************************************************************/
package com.abubusoft.kripton.android.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import com.abubusoft.kripton.android.sqlite.DatabaseLifecycleHandler;
import com.abubusoft.kripton.android.sqlite.KriptonSQLiteHelperFactory;
import com.abubusoft.kripton.android.sqlite.NoDatabaseErrorHandler;
import com.abubusoft.kripton.android.sqlite.NoDatabaseLifecycleHandler;
import com.abubusoft.kripton.android.sqlite.NoPopulator;
import com.abubusoft.kripton.android.sqlite.SQLitePopulator;
import android.database.DatabaseErrorHandler;
import androidx.sqlite.db.SupportSQLiteOpenHelper;
/**
*
* Used to define data-source options with annotation. There are two ways to
* customize data source creating: by code or by annotation. This annotation
* implements the second way. When data-source is created, usually at
* application startup, it is possible to define some features: if it is
* in-memory, if DDL log is enabled, the populator and the migration-version
* tasks and so on.
*
*
*
DataSourceOptions.Builder optionsBuilder=DataSourceOptions.builder();
optionsBuilder.inMemory(false);
optionsBuilder.databaseLifecycleHandler(new DatabaseLifecycleHandler() {
@Override
public void onUpdate(SupportSQLiteDatabase database, int oldVersion, int newVersion, boolean upgrade) {
Logger.info("databaseLifecycleHandler - onUpdate");
}
@Override
public void onCreate(SupportSQLiteDatabase database) {
Logger.info("databaseLifecycleHandler - onCreate "+database.getVersion());
...
}
@Override
public void onConfigure(SupportSQLiteDatabase database) {
Logger.info("databaseLifecycleHandler - onConfigure");
...
}
@Override
public void onCorruption(SupportSQLiteDatabase database) {
Logger.info("databaseLifecycleHandler - onCreate "+database.getVersion());
...
}
});
optionsBuilder.addUpdateTask(1, new SQLiteUpdateTask() {
@Override
public void execute(SupportSQLiteDatabase database, int previousVersion, int currentVersion) {
...
}
});
// create data source
BindAppWithConfigDataSource ds=BindAppWithConfigDataSource.build(optionsBuilder.build());
*
*
*
* To achieve the same result with @BindDataSourceOptions just
* define data source:
*
*
*
* @BindDataSourceOptions(logEnabled = true, populator = PersonPopulator.class, openHelperFactory = KriptonSQLiteOpenHelperFactory.class, databaseLifecycleHandler = PersonLifecycleHandler.class, updateTasks = {
* @BindDataSourceUpdateTask(version = 2, task = PersonUpdateTask.class) })
* @BindDataSource(daoSet = { DaoPerson.class }, fileName = "app.db")
* public interface AppWithConfigDataSource {
*
* }
*
*
* And the code for creating data source will become
*
*
*
* // create data source
* BindAppWithConfigDataSource ds = BindAppWithConfigDataSource.getInstance();
*
*
* Attributes
*
* - openHelperFactory: factory for openHelper used to create
* database.
*
* - databaseLifecycleHandler: databaseLifecycleHandler
* - inMemory: if true, generate database in memory.
* - logEnabled: Log enabled. This flag controls log that is
* not generated by annotation processor, but the log associated to database
* operation like open/close connnections, create tables and so on.
* - populator: is executed after database creation.
* - updateTasks: Allows to specify migration task between
* versions.
*
*
* @author Francesco Benincasa ([email protected])
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface BindDataSourceOptions {
/**
* open helper factory.
*
* @return the class<? extends open helper factory>. Default
* constructor must be defined and public.
*/
Class extends SupportSQLiteOpenHelper.Factory> openHelperFactory() default KriptonSQLiteHelperFactory.class;
/**
* Database error handler.
*
* @return the class<? extends database error handler>
*/
Class extends DatabaseErrorHandler> databaseErrorHandler() default NoDatabaseErrorHandler.class;
/**
* Database lifecycle handler.
*
* @return the class<? extends database lifecycle handler>
*/
Class extends DatabaseLifecycleHandler> databaseLifecycleHandler() default NoDatabaseLifecycleHandler.class;
/**
* if true, generate database in memory.
*
* @return true if you want to generate database in memory
*/
boolean inMemory() default false;
/**
* Log enabled.
*
* @return true to show operations log
*/
boolean logEnabled() default true;
/**
* Add force instance properties to force instance creation during
* datasource build.
*
* It can be very usefull when you need to cipher an existing database and you need to
* open the db before set the upgrade
.
*
* @return forces instance properties to force instance creation during
* datasource build
*/
boolean forceBuild() default false;
/**
* Usually datasource is opened, used and close. If you want to keep it opened after first use, simply
* put neverClose
false
.
* @return
*/
boolean neverClose() default false;
/**
* {@link SQLitePopulator#execute()} is executed after database creation.
*
* @return the class<? extends SQlitePopulator>
*/
Class extends SQLitePopulator> populator() default NoPopulator.class;
/**
* Allows to specify migration task between versions.
*
* @return the bind data source update task[]
*/
BindDataSourceUpdateTask[] updateTasks() default {};
}