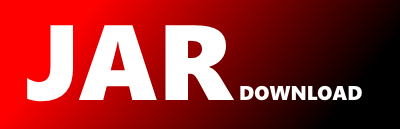
com.abubusoft.kripton.android.annotation.BindSqlInsert Maven / Gradle / Ivy
Show all versions of kripton-orm Show documentation
/*******************************************************************************
* Copyright 2015, 2017 Francesco Benincasa ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*******************************************************************************/
package com.abubusoft.kripton.android.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import com.abubusoft.kripton.android.sqlite.ConflictAlgorithmType;
/**
*
* Allows to insert a bean into database. You can use bean as input parameter or
* method parameters like bean property, but you can not use mixed case.
*
*
* Attributes
*
* - conflictAlgorithm: specifies the conflict algorithm to
* apply during the insert operation. See here for more information.
* - excludedFields: properties to exclude into INSERT
* command. To use only if the method has only one parameter and its type is the
* same of supported bean.
* - fields: allows to specify which fields method need to
* take from bean used as input parameter.
* - value: bean properties to include into INSERT command.
* To use only if method has only one parameter and its type is the same of
* supported bean.
* - includePrimaryKey: Allow to include primary key into
* INSERT command. To use only if the method has only one parameter and its type
* is the same of supported bean.
* - jql: allows specifying the entire query with JQL.
*
*
*
* For example suppose we want to persist bean Person
defined as
* follow:
*
*
*
* @BindType
* public class Person {
* public long id;
*
* public String name;
*
* public String surname;
*
* public String birthCity;
*
* public Date birthDay;
* }
*
*
*
* The associated DAO interface is
*
*
*
* @BindDao(Person.class)
* public interface PersonDAO {
* }
*
*
* Case 1 - Method use a bean type parameter
*
*
* It's possible define a INSERT query with annotation {@link BindSqlInsert}. It
* is possibile to define query parameter simply using method parameter with
* same name of the bean property.
*
*
*
* @BindDao(Person.class)
* public interface PersonDAO {
*
* @BindInsert
* void insertOne(String name, String surname, String birthCity, Date birthDay);
*
* @BindInsert
* long insertTwo(String name, String surname, String birthCity, Date birthDay);
* }
*
*
*
* Each method parameter will be use like input parameter for query. The name of
* parameters will be used to map field bean and then the column name of the
* associated table. If you specify a return type for methods (like method
* insertTwo
), it has to be of type
* int, long, Integer, Long
. In this case, the return value will be
* the id value of just inserted row.
*
*
Case 2 - method use its parameters like bean properties
*
*
* The other way to define an INSERT query is using a bean as input parameter:
*
*
* @BindDao(Person.class)
* public interface PersonDAO {
*
* @BindInsert(excludedFields = { "name", "surname" })
* void insertThree(Person bean);
* }
*
*
*
* You can use attribute value to define which property insert into query
* or you can use attribute excludedFields to avoid to insert some
* fields, but you can not use both attributes in the same method
* definition. Values of this attribute will be used like bean property
* name. At the end of the insert, bean will have id value set to last row id
* used to insert bean into table. If you specify a return type for methods, it
* has to be of type int, long, Integer, Long
and it will contains
* same value like row id.
*
*
* @author Francesco Benincasa ([email protected])
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface BindSqlInsert {
/**
*
* Type of INSERT. Default value is NONE. See
* here for more
* info.
*
*
* @return type of insert.
*/
ConflictAlgorithmType conflictAlgorithm() default ConflictAlgorithmType.NONE;
/**
*
* bean properties to include into INSERT command. To use only if method
* have only one parameter and its type is the same of DAO's supported
* bean.
*
*
* @return property's names to include
*/
String[] fields() default {};
/**
*
* Allow to include primary key into INSERT command. To use only if
* method have only one parameter and its type is the same of DAO's
* supported bean.
*
*
* @return true if you need to include primary key in INSERT COMMAND
*/
boolean includePrimaryKey() default false;
/**
*
* properties to exclude into INSERT command. To use only if method have
* only one parameter and its type is the same of DAO's supported bean.
*
*
* @return property's names to exclude
*/
String[] excludedFields() default {};
/**
*
*
* JQL value. With this attribute, it is possibile to specify directly the
* JQL code. JQL means that you can write SQL using field's names and class
* name indeed of column and table names. Moreover, it is possibile to
* specify where to use the dynamic parts of query through dynamic
* statements like DYNAMIC_WHERE, DYNAMIC_ORDER_BY, DYNAMIC_PAGE_SIZE,
* DYNAMIC_PAGE_OFFSET, encapsulated in #{dynamic-part-name}
*
*
*
* For example, for a select
statement, you can write:
*
*
*
* SELECT * FROM media WHERE mediaId IN (SELECT mediaId FROM fav WHERE #{DYNAMIC_WHERE}) ORDER BY indx DESC LIMIT 0, 100
*
*
* If you use this attribute, no other attributes can be defined for
* the annotation.
*
* @return JQL code specified by user
*/
String jql() default "";
}