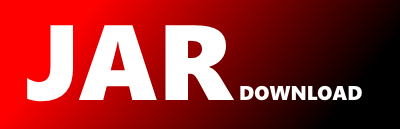
com.abubusoft.kripton.android.sqlite.SQLiteEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kripton-orm Show documentation
Show all versions of kripton-orm Show documentation
Kripton Persistence Library - ORM module
The newest version!
/*******************************************************************************
* Copyright 2016-2019 Francesco Benincasa ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
******************************************************************************/
package com.abubusoft.kripton.android.sqlite;
import com.abubusoft.kripton.android.SqlModificationType;
/**
* Rapresents operations generated by insert, update, delete operations on tables.
*
* In INSERT case, value is the id of the object.
*
* In UPDATE, DELETE situation, it is the number of affected rows.
*
* @author Francesco Benincasa ([email protected])
*
*/
public class SQLiteEvent {
@Override
public String toString() {
return "SQLiteEvent [lastInsertedId=" + lastInsertedId + ", lastInsertedUid=" + lastInsertedUid
+ ", operationType=" + operationType + ", updatedRows=" + updatedRows + "]";
}
/**
* Creates the delete.
*
* @param result
* the result
* @return the SQ lite event
*/
public static SQLiteEvent createDelete(long result) {
return new SQLiteEvent(SqlModificationType.DELETE, result, null, null);
}
/**
* Creates the insert.
*
* @param result
* the result
* @return the SQ lite event
*/
public static SQLiteEvent createInsertWithId(Long result) {
return new SQLiteEvent(SqlModificationType.INSERT, null, result, null);
}
/**
* Creates the insert.
*
* @param result
* the result
* @return the SQ lite event
*/
public static SQLiteEvent createInsertWithUid(String result) {
return new SQLiteEvent(SqlModificationType.INSERT, null, null, result);
}
/**
* Creates the update.
*
* @param result
* the result
* @return the SQ lite event
*/
public static SQLiteEvent createUpdate(long result) {
return new SQLiteEvent(SqlModificationType.UPDATE, result, null, null);
}
/**
* last inserted id
*/
private final Long lastInsertedId;
/**
* last inserted uid
*/
private final String lastInsertedUid;
/** The operation type. */
private final SqlModificationType operationType;
/**
* updated rows
*/
private final Long updatedRows;
/**
* Instantiates a new SQ lite event.
*
* @param operationType
* the operation type
* @param value
* the value
*/
private SQLiteEvent(SqlModificationType operationType, Long updatedRows, Long lastInsertedId,
String lastInsertedUid) {
this.operationType = operationType;
this.updatedRows = updatedRows;
this.lastInsertedId = lastInsertedId;
this.lastInsertedUid = lastInsertedUid;
}
public long getLastInsertedId() {
return lastInsertedId;
}
public String getLastInsertedUid() {
return lastInsertedUid;
}
public SqlModificationType getOperationType() {
return operationType;
}
public Long getUpdatedRows() {
return updatedRows;
}
public boolean hasLastInsertedId() {
return lastInsertedId != null;
}
public boolean hasLastInsertedUid() {
return lastInsertedUid != null;
}
public boolean hasUpdatedRows() {
return updatedRows != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy