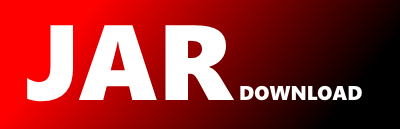
com.abubusoft.kripton.BinderContext Maven / Gradle / Ivy
Show all versions of kripton Show documentation
/*******************************************************************************
* Copyright 2018 Francesco Benincasa ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
******************************************************************************/
package com.abubusoft.kripton;
import java.io.File;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.util.Collection;
import java.util.List;
import java.util.Map;
// TODO: Auto-generated Javadoc
/**
*
* Binder context is interface used to define a persistence context. Every
* persistence context allow to convert Java objects in specific data format and
* viceversa.
*
*
* @author Francesco Benincasa ([email protected])
*
*/
public interface BinderContext {
/**
* return true if class can be persisted.
*
* @param cls
* the cls
* @return true or false
*/
boolean canPersist(Class> cls);
/**
*
* Specifies which data format is supported by this binding context.
*
*
* @return supported data format
*/
BinderType getSupportedFormat();
/**
*
* Parse a byte array to create an instance of objectClazz
* class.
*
*
* @param
* the element type
* @param source
* byte array as input
* @param objectClazz
* class of object to create
* @return instance of object of type objectClazz
*/
E parse(byte[] source, Class objectClazz);
/**
*
* Parse a file to create an instance of objectClazz
class.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return instance of object of type objectClazz
*/
E parse(File source, Class objectClazz);
/**
*
* Parse an input stream to create an instance of objectClazz
* class.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return instance of object of type objectClazz
*/
E parse(InputStream source, Class objectClazz);
/**
*
* Use a reader to create an instance of objectClazz
class.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return instance of object of type objectClazz
*/
E parse(Reader source, Class objectClazz);
/**
*
* Parse a string to create an instance of objectClazz
class.
*
*
* @param
* the element type
* @param buffer
* input
* @param objectClazz
* class of object to create
* @return instance of object of type objectClazz
*/
E parse(String buffer, Class objectClazz);
/**
*
* Parse a string to fill a collection of objectClazz
instance.
*
*
* @param
* the generic type
* @param
* the element type
* @param source
* input
* @param collection
* instance of collection to fill during parsing
* @param objectClazz
* class of object to create
* @return collection of objects of type objectClazz
. It's
* equals to collection param.
*/
, E> L parseCollection(String source, L collection, Class objectClazz);
/**
*
* Parse a byte array to fill a collection of objectClazz
* instance.
*
*
* @param
* the generic type
* @param
* the element type
* @param source
* input
* @param collection
* instance of collection to fill during parsing
* @param objectClazz
* class of object to create
* @return collection of objects of type objectClazz
. It's
* equals to collection param.
*/
, E> L parseCollection(byte[] source, L collection, Class objectClazz);
/**
*
* Parse an inputstream to fill a collection of objectClazz
* instance.
*
*
* @param
* the generic type
* @param
* the element type
* @param source
* input
* @param collection
* instance of collection to fill during parsing
* @param objectClazz
* class of object to create
* @return collection of objects of type objectClazz
. It's
* equals to collection param.
*/
, E> L parseCollection(InputStream source, L collection, Class objectClazz);
/**
*
* Use a reader to fill a collection of objectClazz
instance.
*
*
* @param
* the generic type
* @param
* the element type
* @param source
* input
* @param collection
* instance of collection to fill during parsing
* @param objectClazz
* class of object to create
* @return collection of objects of type objectClazz
. It's
* equals to collection param.
*/
, E> L parseCollection(Reader source, L collection, Class objectClazz);
/**
*
* Parse a byte array to create and populate a list of
* objectClazz
instance.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return list of objects of type objectClazz
*/
List parseList(byte[] source, Class objectClazz);
/**
*
* Parse a string to create and populate a list of objectClazz
* instance.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return list of objects of type objectClazz
*/
List parseList(String source, Class objectClazz);
/**
*
* Parse a string to create and populate a map of key/value. The key is the
* name of field, value is its content.
*
*
* @param source
* input
* @return map of key/value.
*/
Map parseMap(String source);
/**
*
* Parse an inputstream to create and populate a map of key/value. The key
* is the name of field, value is its content.
*
*
* @param source
* input
* @return map of key/value.
*/
Map parseMap(InputStream source);
/**
*
* Use a reader to create and populate a map of key/value. The key is the
* name of field, value is its content.
*
*
* @param source
* input
* @return map of key/value.
*/
Map parseMap(Reader source);
/**
*
* Parse a inputstream to create and populate a list of
* objectClazz
instance.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return list of objects of type objectClazz
*/
List parseList(InputStream source, Class objectClazz);
/**
*
* Use a reader to create and populate a list of objectClazz
* instance.
*
*
* @param
* the element type
* @param source
* input
* @param objectClazz
* class of object to create
* @return list of objects of type objectClazz
*/
List parseList(Reader source, Class objectClazz);
/**
*
* Serialize on a string the object passed as parameter. The used data
* format is the one supported by the binding context
*
*
* @param
* the element type
* @param object
* object to serialize
* @return output
*/
String serialize(E object);
/**
*
* Serialize on a file the object passed as parameter. The used data format
* is the one supported by the binding context
*
*
* @param
* the element type
* @param object
* object to serialize
* @param output
* output
*/
void serialize(E object, File output);
/**
*
* Serialize on an outputstream the object passed as parameter. The used
* data format is the one supported by the binding context
*
*
* @param
* the element type
* @param object
* object to serialize
* @param output
* output
*/
void serialize(E object, OutputStream output);
/**
*
* Serialize on an writer the object passed as parameter. The used data
* format is the one supported by the binding context
*
*
* @param
* the element type
* @param object
* object to serialize
* @param output
* output
*/
void serialize(E object, Writer output);
/**
*
* Serialize a collection on a string. The used data format is the one
* supported by the binding context
*
*
* @param
* the element type
* @param list
* list to serialize
* @param objectClazz
* type of object to persist
* @return output
*/
String serializeCollection(Collection list, Class objectClazz);
/**
*
* Serialize a collection on an output stream. The used data format is the
* one supported by the binding context
*
*
* @param
* the element type
* @param list
* list to serialize
* @param objectClazz
* type of object to persist
* @param output
* output
*/
void serializeCollection(Collection list, Class objectClazz, OutputStream output);
/**
*
* Serialize a collection on an file. The used data format is the one
* supported by the binding context
*
*
* @param
* the element type
* @param list
* list to serialize
* @param objectClazz
* type of object to persist
* @param output
* output
*/
void serializeCollection(Collection list, Class objectClazz, File output);
}