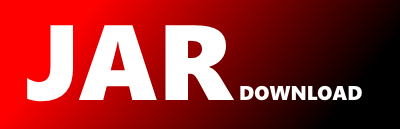
automation.library.api.core.RestAssuredHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of library-api Show documentation
Show all versions of library-api Show documentation
The 'api' library contains a set of java helper classes that support api testing by leveraging Rest-Assured.
The newest version!
package automation.library.api.core;
import static automation.library.common.JsonHelper.getJSONData;
import static automation.library.common.JsonHelper.jsonMerge;
import static io.restassured.matcher.RestAssuredMatchers.matchesXsd;
import static io.restassured.module.jsv.JsonSchemaValidator.matchesJsonSchemaInClasspath;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.contains;
import static org.hamcrest.Matchers.containsInAnyOrder;
import static org.hamcrest.Matchers.endsWith;
import static org.hamcrest.Matchers.equalTo;
import static org.hamcrest.Matchers.hasItem;
import static org.hamcrest.Matchers.hasItems;
import static org.hamcrest.Matchers.hasSize;
import static org.hamcrest.Matchers.is;
import static org.hamcrest.Matchers.lessThan;
import static org.hamcrest.Matchers.not;
import static org.hamcrest.Matchers.nullValue;
import static org.hamcrest.Matchers.startsWith;
import static org.hamcrest.Matchers.containsString;
import static org.hamcrest.Matchers.empty;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import automation.library.common.TestContext;
import com.google.gson.Gson;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
import org.hamcrest.core.IsEqual;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.json.JSONTokener;
import org.skyscreamer.jsonassert.JSONAssert;
import org.skyscreamer.jsonassert.JSONCompareMode;
/**
* Helper class with wrapper methods to leverage the Rest Assured library.
* Allows rest api calls to be configured and invoked followed by response validation.
*/
public class RestAssuredHelper {
public static RequestSpecification setBasicAuth(RequestSpecification request, String uname, String pword) {
return request.auth().preemptive().basic(uname, pword);
}
public static RequestSpecification setChallengedBasicAuth(RequestSpecification request, String uname, String pword) {
return request.auth().basic(uname, pword);
}
public static RequestSpecification setBaseURI(RequestSpecification request, String uri) {
return request.baseUri(uri);
}
public static RequestSpecification setBasePath(RequestSpecification request, String basepath) {
return request.basePath(basepath);
}
public static RequestSpecification setPort(RequestSpecification request, int port) {
return request.port(port);
}
public static RequestSpecification setHeader(RequestSpecification request, String key, String val) {
return request.header(key, val);
}
public static RequestSpecification setParam(RequestSpecification request, String type, String key, String val) {
switch (type) {
case "parameters":
request.param(key, val);
break;
case "form parameters":
request.formParam(key, val);
break;
case "query parameters":
request.queryParam(key, val);
break;
case "path parameters":
request.pathParam(key, val);
break;
}
return request;
}
public static RequestSpecification setMultiPart(RequestSpecification request, File file){
request.multiPart(file);
return request;
}
public static RequestSpecification setMultiPart(RequestSpecification request,String controlName, File file){
request.multiPart(controlName, file);
return request;
}
public static RequestSpecification setMultiPart(RequestSpecification request,String controlName, String contentBody){
request.multiPart(controlName, contentBody);
return request;
}
public static RequestSpecification setMultiPart(RequestSpecification request,String controlName, File file, String mimeType){
request.multiPart(controlName, file, mimeType);
return request;
}
public static RequestSpecification setMultiPart(RequestSpecification request,String controlName, String contentBody, String mimeType){
request.multiPart(controlName, contentBody, mimeType);
return request;
}
public static RequestSpecification setParamList(RequestSpecification request, String key, List val) {
return request.param(key, val);
}
public static RequestSpecification setBody(RequestSpecification request, String content) {
return request.body(content);
}
public static Response callAPI(RequestSpecification request, String method, String path) {
Response response = null;
switch (method) {
case "GET":
response = request.get(path);
break;
case "PUT":
response = request.put(path);
break;
case "POST":
response = request.post(path);
break;
case "PATCH":
response = request.patch(path);
break;
case "DELETE":
response = request.delete(path);
break;
}
return response;
}
public static void checkStatus(RestData restData, int statusCode) {
restData.getRespValidator().assertThat().statusCode(statusCode);
}
public static void checkStatus(RestData restData, String msg) {
restData.getRespValidator().assertThat().statusLine(containsString(msg));
}
public static void checkSchema(RestData restData, String type, String path) {
switch (type) {
case "json":
restData.getRespValidator().body(matchesJsonSchemaInClasspath(path));
break;
case "xml":
restData.getRespValidator().body(matchesXsd(path));
break;
}
}
public static void checkResponseTime(RestData restData, long duration) {
restData.getRespValidator().assertThat().time(lessThan(duration));
}
public static void checkHeader(RestData restData, String key, String matcher, Object val) {
String act = restData.getResponse().header(key);
switch (matcher) {
case "equals":
assertThat(act, equalTo(val));
break;
case "regex":
assertThat("value " + act.toString() + " does not match regex " + val.toString(), act.toString().matches(val.toString()), is(true));
break;
case "isNull":
assertThat(act, nullValue());
break;
case "!isNull":
assertThat(act, not(nullValue()));
break;
}
}
public static void checkBody(JsonPath jsonPath, Object[] obj, String element, String matcher) {
Object act = jsonPath.get(element);//from(respString).get(element);
Object nullObj = null;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy