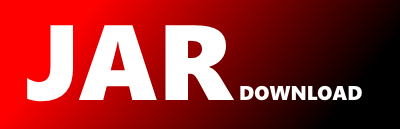
org.kawanfw.sql.api.server.DatabaseConfigurator Maven / Gradle / Ivy
Show all versions of aceql-http Show documentation
/*
* Copyright (c)2022 KawanSoft S.A.S. All rights reserved.
*
* Use of this software is governed by the Business Source License included
* in the LICENSE.TXT file in the project's root directory.
*
* Change Date: 2026-11-01
*
* On the date above, in accordance with the Business Source License, use
* of this software will be governed by version 2.0 of the Apache License.
*/
package org.kawanfw.sql.api.server;
import java.io.File;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import org.slf4j.Logger;
/**
*
* Interface that defines the database configurations for AceQL HTTP.
*
* The implemented methods will be called by AceQL when a client program,
* referred by a user username, asks for a JDBC operation from the Client side.
*
* A concrete implementation should be developed on the server side in order to:
*
* - Define how to extract a JDBC Connection from a Connection Pool.
* - Define the directories where the Blobs/Clobs are located for upload and
* download.
* - Define some Java code to execute before/after a
*
Connection.close()
.
* - Define the maximum number of rows that may be returned to the
* client.
* - Define the {@code Logger} to use to trap server Exceptions.
*
*
*
* @author Nicolas de Pomereu
*/
public interface DatabaseConfigurator {
/**
*
* Attempts to establish a connection with an underlying data source.
*
* @param database the database name to get the connection from
*
* @return a Connection
to the data source
* @exception SQLException if a database access error occurs
*/
public Connection getConnection(String database) throws SQLException;
/**
* Allows to define how to close the Connection acquired with
* {@link DatabaseConfigurator#getConnection(String)} and return it to the pool.
*
*
* Most connection pool implementations just require to call
* Connection.close()
to release the connection, but it is
* sometimes necessary for applications to add some operation before/after the
* close. This method allows you to add specific code before or after the close.
*
*
* It is not required to implement this method, because the default
* implementation {@link DefaultDatabaseConfigurator#close(Connection)} closes
* the connection with an explicit call to Connection.close()
.
* If you implement this method, you *must* close the connection.
*
* @param connection a connection to the data source
* @throws SQLException if a SQLException occurs
*/
public void close(Connection connection) throws SQLException;
/**
* Allows to define the maximum rows per request to be returned to the client.
* If this limit is exceeded, the excess rows are silently dropped.
*
* @param username the client username to check the max rows for.
* @param database the database name as defined in the JDBC URL field
* @return the maximum rows per request to be returned to the client; zero means
* there is no limit
* @throws IOException if an IOException occurs
* @throws SQLException if a SQLException occurs
*/
public int getMaxRows(String username, String database) throws IOException, SQLException;
/**
* Allows to define the directory into which Blobs/Clobs are uploaded by client
* side, and from which Blobs/Clobs are downloaded by client side.
* See default implementation in:
* {@link DefaultDatabaseConfigurator#getBlobsDirectory(String)}.
*
* @param username the client username
* @return the Blob/Clob upload and download directory for this username
* @throws IOException if an IOException occurs
* @throws SQLException if a SQLException occurs
*/
public File getBlobsDirectory(String username) throws IOException, SQLException;
/**
* Returns the SLF4J {@link Logger} that will be used by AceQL for logging:
*
* - All Exceptions thrown by server side will be logged.
* - Exceptions thrown are flattened when logged for compatible usage with tools like Logstash.
*
* It is not necessary nor recommended to implement this method; do it only if
* you want take control of the logging to modify the default characteristics of
* {@link DefaultDatabaseConfigurator#getLogger()}.
*
* @return the {@code Logger} that will be used by AceQL logging;
* @throws IOException if an IOException occurs
*/
public Logger getLogger() throws IOException;
}