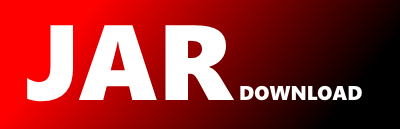
org.kawanfw.sql.api.server.SqlEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aceql-http Show documentation
Show all versions of aceql-http Show documentation
AceQL HTTP is a framework of REST like http APIs that allow to access to remote SQL databases over http from any device that supports http.
AceQL HTTP is provided with four client SDK:
- The AceQL C# Client SDK allows to wrap the HTTP APIs using Microsoft SQL Server like calls in their code, just like they would for a local database.
- The AceQL Java Client SDK allows to wrap the HTTP APIs using JDBC calls in their code, just like they would for a local database.
- The AceQL Python Client SDK allows SQL calls to be encoded with standard unmodified DB-API 2.0 syntax
/*
* Copyright (c)2022 KawanSoft S.A.S. All rights reserved.
*
* Use of this software is governed by the Business Source License included
* in the LICENSE.TXT file in the project's root directory.
*
* Change Date: 2026-11-01
*
* On the date above, in accordance with the Business Source License, use
* of this software will be governed by version 2.0 of the Apache License.
*/
package org.kawanfw.sql.api.server;
import java.util.List;
import java.util.Objects;
import org.kawanfw.sql.util.SqlEventUtil;
/**
* Allows to get all details of a SQL event asked by the client side.
*
* @author Nicolas de Pomereu
* @since 9.0
*/
public class SqlEvent {
private String username;
private String database;
private String ipAddress;
private String sql;
private boolean isPreparedStatement;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy