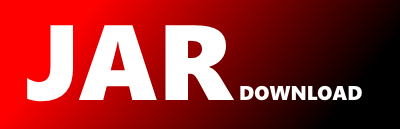
org.kawanfw.sql.api.server.firewall.SqlFirewallManager Maven / Gradle / Ivy
Show all versions of aceql-http Show documentation
/*
* Copyright (c)2022 KawanSoft S.A.S. All rights reserved.
*
* Use of this software is governed by the Business Source License included
* in the LICENSE.TXT file in the project's root directory.
*
* Change Date: 2026-11-01
*
* On the date above, in accordance with the Business Source License, use
* of this software will be governed by version 2.0 of the Apache License.
*/
package org.kawanfw.sql.api.server.firewall;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import org.kawanfw.sql.api.server.SqlEvent;
import org.kawanfw.sql.api.server.StatementAnalyzer;
/**
* Interface that allows to define firewall rules for AceQL HTTP SQL calls.
*
* Concrete implementations are defined in the {@code aceql-server.properties}
* file.
*
* A concrete implementation should be developed on the server side in order to:
*
* - Define a specific piece of Java code to analyze the source code of the
* SQL statement before allowing or not it's execution.
* - Define if a client user has the right to call a
*
Statement.execute
(i.e. call a raw execute).
* - Define if a client user has the right to call a raw
*
Statement
that is not a PreparedStatement
.
* - Define if a client user has the right to call a the AceQL Metadata
* API.
*
*
* Multiple {@code SqlFirewallManager} may be defined and chained.
* When {@code SqlFirewallManager} classes are chained, an {@code AND} condition
* is applied to all the SqlFirewallManager execution conditions in order to
* compute final allow.
* For example, the {@code allowExecuteUpdate()} of each chained
* {@code SqlFirewallManager} instance must return true in order to allow
* updates of the database.
*
* Built in and ready to use classes that don't require any coding are included.
* The classes may be chained. See each Javadoc for more details:
*
* - {@link CsvRulesManager}: manager that apply rules written in a CSV
* file.
* - {@link CsvRulesManagerNoReload}: same as {@code CsvRulesManager}, but
* dynamic reload of rules is prohibited if the CSV file is updated.
* - {@link DenyDatabaseWriteManager}: manager that denies any update of the
* database.
* - {@link DenyDclManager}: manager that denies any DCL (Data Control
* Language) call.
* - {@link DenyDdlManager}: manager that denies any DDL (Data Definition
* Language) call.
* - {@link DenyExceptOnWhitelistManager}: manager that allows only statements
* that are listed in a whitelist text file.
* - {@link DenyMetadataQueryManager}: manager that denies the use of the
* AceQL Metadata Query API.
* - {@link DenyOnBlacklistManager}: manager that denies statements that are
* listed in a blacklist text file.
* - {@link DenySqlInjectionManager}: manager that allows detecting
* SQL injection attacks, using
* Cloudmersive third-party API.
* - {@link DenySqlInjectionManagerAsync}: version of
* {@code DenySqlInjectionManager} that detects SQL injections asynchronously
* for faster response time.
* - {@link DenyStatementClassManager}: manager that denies any call of the
* raw Statement Java class. (Calling Statements without parameters is
* forbidden).
*
*
* TCL (Transaction Control Language) calls are always authorized.
*
* Note that the helper class {@link StatementAnalyzer} allows to do some simple
* tests on the SQL statement string representation.
*
* @author Nicolas de Pomereu
* @since 4.1
*/
public interface SqlFirewallManager {
/**
* Allows to analyze the SQL call event asked by the client side and thus allow
* or forbid the SQL execution on the server.
* If the analysis defined by the method returns false, the SQL statement won't
* be executed.
*
* @param sqlEvent the SQL event asked by the client side. Contains all info
* about the SQL call (client username, database name, IP
* Address of the client, and SQL statement details)
* @param connection The current SQL/JDBC Connection
* @return true
if the analyzed statement or prepared statement is
* validated and authorized to run, else false
*
* @throws IOException if an IOException occurs
* @throws SQLException if a SQLException occurs
*/
public boolean allowSqlRunAfterAnalysis(SqlEvent sqlEvent, Connection connection) throws IOException, SQLException;
/**
* Allows to define if the passed username is allowed to create and use a
* {@link Statement} instance that is not a PreparedStatement
.
*
* @param username the client username to check the rule for
* @param database the database name as defined in the JDBC URL field
* @param connection The current SQL/JDBC Connection
* @return true
if the user has the right to call a raw
* execute
*
* @throws IOException if an IOException occurs
* @throws SQLException if a SQLException occurs
*
*/
public boolean allowStatementClass(String username, String database, Connection connection)
throws IOException, SQLException;
/**
* Says if the username is allowed call the Metadata Query API for the passed
* database.
*
* @param username the client username to check the rule for
* @param database the database name as defined in the JDBC URL field
* @param connection The current SQL/JDBC Connection
* @return true
if the user has the right to call the Metadata
* Query API, else false
* @throws IOException if an IOException occurs
* @throws SQLException if a SQLException occurs
*/
public boolean allowMetadataQuery(String username, String database, Connection connection)
throws IOException, SQLException;
}