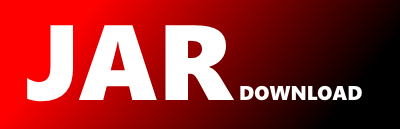
org.kawanfw.sql.api.server.session.SessionConfigurator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aceql-http Show documentation
Show all versions of aceql-http Show documentation
AceQL HTTP is a framework of REST like http APIs that allow to access to remote SQL databases over http from any device that supports http.
AceQL HTTP is provided with four client SDK:
- The AceQL C# Client SDK allows to wrap the HTTP APIs using Microsoft SQL Server like calls in their code, just like they would for a local database.
- The AceQL Java Client SDK allows to wrap the HTTP APIs using JDBC calls in their code, just like they would for a local database.
- The AceQL Python Client SDK allows SQL calls to be encoded with standard unmodified DB-API 2.0 syntax
/*
* Copyright (c)2022 KawanSoft S.A.S. All rights reserved.
*
* Use of this software is governed by the Business Source License included
* in the LICENSE.TXT file in the project's root directory.
*
* Change Date: 2026-11-01
*
* On the date above, in accordance with the Business Source License, use
* of this software will be governed by version 2.0 of the Apache License.
*/
package org.kawanfw.sql.api.server.session;
import java.io.IOException;
/**
* Interface that defines how to generate and verify session id for (username,
* database) sessions.
*
* Interface implementation allows to:
*
* - Define how to generate a session id after client /connect call.
* - Define the sessions lifetime.
* - Define how to verify that the stored session is valid and not
* expired.
*
*
* Two implementations are provided:
*
* - The {@link DefaultSessionConfigurator} default implementation stores user
* info on the server.
* - The {@link JwtSessionConfigurator} implementation generates self
* contained JWT (JSON Web Tokens) and there is no info storage on the
* server.
*
*
* @author Nicolas de Pomereu
*/
public interface SessionConfigurator {
/**
* Generates a unique session id for the (username, database) couple. The
* session id is used to authenticate clients calls that pass it at each http
* call.
*
* Method may be also use to store in server memory the (username, database)
* couple for the generated session id. This is done in the default
* implementation {@link DefaultSessionConfigurator}.
*
* @param username the username to store for the passed session id
* @param database the database to store for the passed session id
*
* @return a unique session id for the (username, database) couple.
* @throws IOException if any I/O error
*/
public String generateSessionId(String username, String database) throws IOException;
/**
* Loads the username stored for the passed session id
*
* @param sessionId the session id
* @return the username stored for the passed session Id
*/
public String getUsername(String sessionId);
/**
* Loads the database stored for the passed session id
*
* @param sessionId the session id
* @return the database stored for the passed session Id
*/
public String getDatabase(String sessionId);
/**
* Removes storage for the passed session Id. Method is called by AceQL when
* client side calls {@code logout}
*
* @param sessionId the session id
*/
public void remove(String sessionId);
/**
* Verify that this session id corresponds to an existing and is still valid
* (non expired).
*
* @param sessionId the session id to verify
* @return true if the sessionId is valid
* @throws IOException if any I/O error
*/
public boolean verifySessionId(String sessionId) throws IOException;
/**
* Allows to define the sessions lifetime in minutes
*
* @return the sessions lifetime in minutes
* @throws IOException if any I/O error
*/
public int getSessionTimelifeMinutes() throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy